INTRODUCTION
I intend to make an automated system for my home with a combination of PLC SIEMENS and ARDUINO. I think we can combine their own strengths to make the automation system running in stability, optimization and acceptable price. So I took times to consult, study and perform some below experiments before applying them to my home.
* Toogle a lamp 220 VAC from touch button of Arduino via Profibus-DP.
* Display the PT100 temperature value on the 64x64 led matrix via Profibus-DP
- 01pcs x DFRduino UNO R3 - Arduino Compatible.
- 01pcs x DFRobot Mega 2560 (Optional).
- 01pcs x DFRobot RS485 Shield for Arduino.
- 01pcs x PLC S7-300.
- 01pcs x Relay 24VDC.
- 01pcs x Inductive sensor.
- 01pcs x Lamp 220VAC.
- 01pcs x Touch button with LEDs indicator.
- 03 meters x Profibus cable.
- 03pcs x Profibus connectors.
2.1. SOFTWARES:
- SIMATIC STEP 7 V5.6/ SIMATIC STEP 7 V5.6 PROFESSIONAL.
- Arduino IDE.
2.2. REFERENCE MANUALS:
- Arduino's UART, Timer.
- Learn about *.GSD file to configure Arduino into Siemens PLC hardware system.
The PROFIBUS-DP network is based on an RS485 physical communication and it has to strictly follow the telegram format structure and telegram sequences defined by PROFIBUS network. And RS485 designs use the same basic UART but it convert the UART signal into a bi-directional differential signal. RS 485 transmission technology uses two-cores cable, with positive red core B+ and negative green core A-. The benefit of using a differential signal to minimize noises and allowing for longer cable lengths, up to 1.2 km. It allows for fast data communications up to a bit rate of 12 Mbit/s.
* UART CODING AND FRAME FORMAT

* PROFIBUS-DP TELEGRAM FORMATS

Each byte in a telegram is transferred as 11 bits (START = 0 ; DATA = 8 BIT ; PARITY = EVEN ; STOP = 1), see FRAME FORMAT picture above.
On PROFIBUS-DP, certain time ratios must be respected to ensure that a telegram sequence can function correctly.
The duration of a bit differs depending on bit rate: for 12 MBit/s tBit = 83 ns and for 1.5 MBit/s tBit = 0.67 µs.
4.1. PLC CONNECTION

4.2. ARDUINO CONNECTION

4.3. TESTING DESCRIPTIONS
The 220VAC lamp can be controlled in toogle mode by virtual and physical input as follows:
* Touch button is connected to Arduino plus RS485 shield and send the command to PLC S7-300 via Profibus-DP network at Virtual Input I0.0
* Inductive sensor is connected to PLC S7-300 directly at Physical Input I124.0
* 24VDC relay coil is connected to PLC Physical Output Q124.0 and lamp 220VAC is connected to N.O (normal open) contact of this relay. Even though the lamp is controlled by Arduino or PLC, Arduino receive the feedback of lamp status from PLC at virtual output Q0.0 and display lamp status by red led (lamp 220VAC off) /green led (lamp 220VAC on) on touch button.
We can see more detail in "PLC PROGRAMING" step.
5.1. PLC HARDWARE CONFIG
In Simatic Step 7 program (HW CONFIG), we have to install ARDUINO *.GSD file and update your Hardware Catalog using the menu command "Options --> Update catalog". You can check detail at this link to know how to integrate a GSD file into the STEP 7 HW Config for PROFIBUS.
With Arduino - F_CPU = 16MHz, Profibus network works well at baudrate 45.45 Kbps.
We need to define the number of bytes transmitted and received over the Profibus connection, we can see this setup on above picture:
- 1 Byte Input: PLC receive 1 byte input at virtual input address IB0.
- 1 Byte Output: PLC transmit 1 byte output at virtual output address QB0.

5.2. PLC PROGRAMING
It is simple PLC program with:
* OB1 - Main program.
* DB1 - Data Block for storage toogle state of LAMP (at DB1.DBX0.0).
* FB1 - Function Block for carrying out toggle function. It toggles the output Q state with every rising edge of clock (CLK).
* DB2 - Instant Data Block for FB1.

Program explanation:
* I0.0 - PLC Virtual Input which is received from Touch Button of Arduino via Profibus-DP.
* I124.0 - PLC Physical Input which is directly connected to Inductive Sensor.
* Q124.0 - PLC Physical Output which is connected to coil relay 24VDC.
* PQB0 - PLC Virtual Output Byte (8bit) which is transmitted to Arduino via Profibus-DP.
"TOOGLE" Function Block FB1 written in SCL (Structured Control Language) can be copied to S7 program/ Sources folder and compiled to LAD/STL. This Function Block toggles the output Q state when it detects every rising edge of clock (CLK). We can see in program, "TOOGLE" function block will toogle Q124.0 when it detects one rising edge pulse at virtual input I0.0 or physical input I124.0
FUNCTION_BLOCK TOGGLE
TITLE = 'TOGGLE'
VAR_INPUT
CLK : BOOL;
RST : BOOL;
END_VAR
VAR_OUTPUT
Q : BOOL;
END_VAR
VAR
EDGE : BOOL;
END_VAR
BEGIN
IF RST THEN
Q := 0;
ELSIF CLK AND NOT EDGE THEN
Q := NOT Q;
END_IF;
EDGE := CLK;
END_FUNCTION_BLOCK
The Arduino code including *.GSD file for PLC Hardware Configuration is available at my GitHub.
My program is referenced from German mikrocontroller website by Jörg S., Marc H., Johannes F. and originally it is written for MSP430F2252. If you are German I believe you will collect a lot of information here.
For RS485 Shield designed by DFROBOT, it is useful when it is equipped with additional button "Operation and programming mode switch" because Arduino Uno has only 1 UART port for programming and RS485 communication.
* Switch to OFF: you can download the program for the Arduino controller.
* Switch to ON: the shield will convert the UART to the RS485.

Important note:
* The Profibus-DP based on RS485 physical communication has two independent lines known as negative line - A and positive line - B, which transmit similar voltage levels however with opposite polarities. For this reason, it is important that the network is connected with the correct polarity. As my testings with some RS485 modules, in order to correct their polarities, I had to swap 2 cores A and B of Profibus cable together for most RS485 modules at Arduino-side.
* In order for network working properly, the GND of PLC and the GND of Arduino must be connected together.
PLC S7-300 read temperature value from PT100 then send this value via Profibus-DP to Arduino and display on RGB led matrix 64x64.
Take note that PLC SIEMENS S7 ™ use "Big-Endian" data format and ARDUINO™ use "Little-Endian" data format. The terms "Big-Endian" and "Little-Endian" to describe differences in reading and writing data.
You can check more detail at my post on Instructable.

Thank for your watching!
Please LIKE and SUBSCRIBE to my YouTube channel.
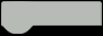