This is a smart and cool way to count Euro coins using a scale and Arduino. It's very easy to build and can be done with simple components like:
- #ArduinoNano
- Oled display I2C 128x32
- Push Button
- Weight Sensor with #HX711 converter
HARDWARE LIST
1 Arduino Nano
1 Push Button
1 OLED 128x32 Display
1 Scale sensor with HX711
CODE
//
// FILE: Coin_Count.ino
// AUTHOR: Carlo Stramaglia
// URL: https://www.youtube.com/c/CarloStramaglia
//
#include "HX711.h"
#include <U8x8lib.h>
#include "OneButton.h"
HX711 scale;
uint8_t dataPin = 6;
uint8_t clockPin = 7;
OneButton button(4, true);
U8X8_SSD1306_128X32_UNIVISION_SW_I2C u8x8(/* clock=*/ SCL, /* data=*/ SDA, /* reset=*/ U8X8_PIN_NONE);
int status = 0;
float Cent = 0;
float TwoCent = 0;
float FiveCent = 0;
float TenCent = 0;
float TwentyCent = 0;
float FiftyCent = 0;
float OneEuro = 0;
float TwoEuro = 0;
int TotalCents = 0;
float TotalEuro = 0;
void setup()
{
Serial.begin(115200);
scale.begin(dataPin, clockPin);
u8x8.begin();
u8x8.setPowerSave(0);
button.attachClick(Click);
}
void loop()
{
char ValueEuro [8];
button.tick();
u8x8.setFont(u8x8_font_chroma48medium8_r);
delay (50);
if (status == 0) {
Serial.println("\nEmpty the scale and press the button to continue");
u8x8.drawString(0,1,"Empty scale");
u8x8.drawString(0,2,"Press button");
u8x8.drawString(0,3,"To Continue");
}
if (status == 1) {
Serial.println("\nPlace 4 coins of 2€ and press the button to continue");
u8x8.drawString(0,1,"Put 4 2E coins");
u8x8.drawString(0,2,"Press button");
u8x8.drawString(0,3,"To Continue");
}
if (status == 2) {
Serial.println("\nPlace 1 Cent coins and press the button to continue");
u8x8.drawString(0,1,"Put 1 cent coins");
u8x8.drawString(0,2,"Press button");
u8x8.drawString(0,3,"To Continue");
}
if (status == 3) {
Serial.println("\nAdd 2 Cents coins and press the button to continue");
u8x8.drawString(0,1,"Put 2 cent coins");
u8x8.drawString(0,2,"Press button");
u8x8.drawString(0,3,"To Continue");
}
if (status == 4) {
Serial.println("\nAdd 5 Cents coins and press the button to continue");
u8x8.drawString(0,1,"Put 5 cent coins");
u8x8.drawString(0,2,"Press button");
u8x8.drawString(0,3,"To Continue");
}
if (status == 5) {
Serial.println("\nAdd 10 Cents coins and press the button to continue");
u8x8.drawString(0,1,"Put 10 cent coin");
u8x8.drawString(0,2,"Press button");
u8x8.drawString(0,3,"To Continue");
}
if (status == 6) {
Serial.println("\nAdd 20 Cents coins and press the button to continue");
u8x8.drawString(0,1,"Put 20 cent coin");
u8x8.drawString(0,2,"Press button");
u8x8.drawString(0,3,"To Continue");
}
if (status == 7) {
Serial.println("\nAdd 50 Cents coins and press the button to continue");
u8x8.drawString(0,1,"Put 50 cent coin");
u8x8.drawString(0,2,"Press button");
u8x8.drawString(0,3,"To Continue");
}
if (status == 8) {
Serial.println("\nAdd 1 Euro coins and press the button to continue");
u8x8.drawString(0,1,"Put 1 Euro coins");
u8x8.drawString(0,2,"Press button");
u8x8.drawString(0,3,"To Continue");
}
if (status == 9) {
Serial.println("\nAdd 2 Euro coins and press the button to continue");
u8x8.drawString(0,1,"Put 2 Euro coins");
u8x8.drawString(0,2,"Press button");
u8x8.drawString(0,3,"To Continue");
}
if (status == 10) {
sprintf(ValueEuro, "%d", TotalEuro);
dtostrf(TotalEuro, 2, 2, ValueEuro);
u8x8.drawString(0,1,ValueEuro);
ValueEuro[5]=byte(0xb0);
ValueEuro[6]='C';
ValueEuro[7]='\0';
u8x8.drawString(0,2," ");
u8x8.drawString(0,3,"End of process");
Serial.println(ValueEuro);
}
// Serial.print("UNITS: ");
// Serial.println(scale.get_units(10));
// delay(150);
}
void Click() {
Serial.println ("Button pressed");
if (status == 9) {
Serial.read();
Serial.print("2 Euro Coins float: ");
TwoEuro = scale.get_units(10)-Cent-TwoCent-FiveCent-TenCent-TwentyCent-FiftyCent-OneEuro;
TotalCents = TotalCents + ((int (TwoEuro/8.50+0.5))*200);
TotalEuro = (float) TotalCents/100;
Serial.print("Total Cents so far: €");
Serial.println(TotalEuro);
status = 10;
Serial.print("");
}
else if (status == 8) {
Serial.read();
Serial.print("1 Euro Coins float: ");
OneEuro = scale.get_units(10)-Cent-TwoCent-FiveCent-TenCent-TwentyCent-FiftyCent;
TotalCents = TotalCents + ((int (OneEuro/7.50+0.5))*100);
Serial.print("Total Cents so far: ");
Serial.println(TotalCents);
status = 9;
Serial.print("");
}
else if (status == 7) {
Serial.read();
Serial.print("50 Cent Coins float: ");
FiftyCent = scale.get_units(10)-Cent-TwoCent-FiveCent-TenCent-TwentyCent;
TotalCents = TotalCents + ((int (FiftyCent/7.80+0.5))*50);
Serial.print("Total Cents so far: ");
Serial.println(TotalCents);
status = 8;
Serial.print("");
}
else if (status == 6) {
Serial.read();
Serial.print("20 Cent Coins float: ");
TwentyCent = scale.get_units(10)-Cent-TwoCent-FiveCent-TenCent;
TotalCents = TotalCents + ((int (TwentyCent/5.74+0.5))*20);
Serial.print("Total Cents so far: ");
Serial.println(TotalCents);
status = 7;
Serial.print("");
}
else if (status == 5) {
Serial.read();
Serial.print("10 Cent Coins float: ");
TenCent = scale.get_units(10)-Cent-TwoCent-FiveCent;
TotalCents = TotalCents + ((int (TenCent/4.1+0.5))*10);
Serial.print("Total Cents so far: ");
Serial.println(TotalCents);
status = 6;
Serial.print("");
}
else if (status == 4) {
Serial.read();
Serial.print("5 Cent Coins float: ");
FiveCent = scale.get_units(10)-Cent-TwoCent;
TotalCents = TotalCents + ((int (FiveCent/3.92+0.5))*5);
Serial.print("Total Cents so far: ");
Serial.println(TotalCents);
status = 5;
Serial.print("");
}
else if (status == 3) {
Serial.read();
Serial.print("2 Cent Coins float: ");
TwoCent = scale.get_units(10)-Cent;
TotalCents = TotalCents + ((int (TwoCent/3.06+0.5))*2);
Serial.print("Total Cents so far: ");
Serial.println(TotalCents);
status = 4;
Serial.print("");
}
else if (status == 2) {
Serial.read();
Serial.print("1 Cent Coins float: ");
Cent = scale.get_units(10);
TotalCents = int (Cent/2.30+0.5);
Serial.print("Total Cents so far: ");
Serial.println(TotalCents);
status = 3;
Serial.print("");
}
else if (status == 1) {
Serial.read();
scale.callibrate_scale(34, 5);
Serial.print("Grams: ");
Serial.println(scale.get_units(10));
status = 2;
Serial.print("Calibrated");
}
else if (status == 0) {
Serial.read();
scale.tare();
Serial.print("Grams: ");
Serial.println(scale.get_units(10));
status = 1;
}
}
License 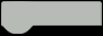
All Rights
Reserved
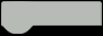
0
More from this category