Let's teach Arduino some drum lessons! We're using an Arduino, a servo motor and a sound sensor.

HARDWARE LIST
1 DFRobot Bluno M3
1 Adafruit Microphone Sound Sensor KY-038 Module
1 SG90 Micro-servo motor
1 LED (generic)
1 Resistor 220 ohm
1 Jumper wires (generic)
Software apps and online services
Arduino IDE
Hand tools and fabrication machines
Hot glue gun (generic)
Story
We are going to give Arduino some drum lessons.
Everything is nicely explained in the video below.
Connect all components according to the diagram at the bottom of this page.
PAY ATTENTION:
The video uses a Bluno M3 from DFRobot, but you can ALSO create the project using ANOTHER ARDUINO BOARD such as an ARDUINO UNO. You just need to use a different library in the code (see comment).
Schematics
Schematic for Bluno M3 Drum Lessons

Code
CODE
/*
* Version 1.0 August, 2021
* Copyright (c) 2021 Korneel Verraes
* https://lego-projecten.webnode.nl/
*
* Bluno M3 Arduino Drum Lessons:
* A sound sensor with digital output is connected to pin 7
* A servo motor is connected to pin 9
* An LED is connected to pin 13
*
* Accompanying video: https://youtu.be/OXwlFxWnDI8
*/
#include <ServoM3.h> //use this on Bluno M3
//#include <Servo.h> //use this on other Arduino boards (e.g.: Arduino UNO, Arduino MEGA...)
Servo servo;
int clapCount = 0;
int clapTime[50];
int led = 13;
int sensor = 7;
boolean clap = 0;
void setup() {
servo.attach(9);
servo.write(0);
pinMode(led, OUTPUT);
pinMode(sensor, INPUT);
}
void loop() {
clap = digitalRead(sensor);
if (clap == 1) {
digitalWrite(led, HIGH);
clapTime[clapCount] = millis();
clapCount++;
delay(200);
}
else {
digitalWrite(led, LOW);
}
if ((millis() - clapTime[clapCount - 1]) > 2000 && clapCount != 0) {
for (int i = 1; i < clapCount ; i++) {
servo.write(13);
delay(80);
servo.write(0);
delay (clapTime[i] - clapTime[i - 1] - 80);
}
servo.write(13);
delay(80);
servo.write(0);
delay(800);
clapCount = 0;
}
}
The article was first published in hackster, August 22, 2021
cr: https://www.hackster.io/legoev3projects/arduino-drum-lessons-73e065
author: legoev3projects
License 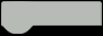
All Rights
Reserved
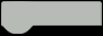
0
More from this category