This is a timer that can be setup without watching the device using just a push button. It can be useful for blind.

Things used in this project
Hardware components
Hand tools and fabrication machines
Drill / Driver, Cordless
Story
I was looking for a timer that could be setup without looking at the display. Mainly the purpose was to have, while cooking, a voice telling me how many minutes were left before the end.
So I created this simple device with the intent to use it in the kitchen.
The use is very simple. There is only one push button that every time you press it, there is an increase of one minute and a voice tells you how many minutes you have accumulated. If you make a mistake, you need to keep a long push on the button and the counter is reset.
Once you have finished, you just need to double click the push button and then the counter starts.
Every minute, a voice tells you how many minutes are missing before the end.
I used very simple components and for the voice I used a DFRobot Gravity DFR0760 module. It works quite well.
Schematics
Smart Timer
Code
Smart Timer
Arduino
/*
* Carlo Stramaglia
* https://www.youtube.com/c/CarloStramaglia
* This is a Smart Timer that
* once you set it up, will remind you every quarter to check the food in the pan
* June 1st 2021
*/
#include "DFRobot_SpeechSynthesis.h"
#include <TM1637.h>
#include "OneButton.h"
int minutes=00;
int seconds=0;
char tmp[5];
DFRobot_SpeechSynthesis_I2C ss;
// Display Pins configurations
// Pin 3 - > DIO
// Pin 2 - > CLK
TM1637 tm1637(2, 3);
// Push Button PIN definition
#define PIN_INPUT A2
OneButton button(PIN_INPUT, true);
void setup() {
Serial.begin(115200);
Serial.println("Smart Timer sketch. Carlo Stramaglia https://www.youtube.com/c/CarloStramaglia");
//Init speech synthesis sensor
ss.begin();
//Set voice volume to 7
ss.setVolume(7);
//Set playback speed to 5
ss.setSpeed(5);
//Set speaker to female
ss.setSoundType(ss.MALE1);
//Set tone to 5
ss.setTone(5);
ss.setSpeechStyle(ss.SMOOTH);
ss.setEnglishPron(ss.WORD);
ss.setDigitalPron(ss.AUTOJUDGED);
ss.setLanguage(ss.ENGLISHL);
tm1637.init();
tm1637.setBrightness(5);
button.attachClick(singleClick);
button.attachDoubleClick(doubleClick);
button.attachLongPressStart(longPressStart);
tm1637.clearScreen();
tm1637.colonOn();
tm1637.display("0000");
tm1637.colonOn();
delay(100);
}
void loop() {
button.tick();
delay (10);
}
void singleClick()
{
Serial.println("x1");
minutes++;
Serial.println(minutes);
sprintf(tmp, "%d", minutes);
ss.speak((tmp));
delay (100);
ss.speak(F("minutes set"));
if (minutes < 10)
tm1637.display(minutes,1,0,1);
else
tm1637.display(minutes);
Serial.println(tmp);
delay(100);
} // singleClick
void doubleClick()
{
Serial.println("x2");
if (minutes == 0) return;
ss.speak(F("Starting countdown"));
minutes--;
for (minutes ; minutes >= 0; minutes--) {
for (int seconds = 59; seconds >= 0; seconds--) {
if (minutes < 10 && minutes >0)
tm1637.display(seconds + minutes*100,1,0,1);
else if (minutes == 0 && seconds >=10)
tm1637.display(seconds + minutes*100,1,0,2);
else if (minutes == 0 && seconds < 10)
tm1637.display(seconds + minutes*100,1,0,3);
else
tm1637.display(seconds + minutes*100);
tm1637.switchColon();
if (seconds == 0)
{
sprintf(tmp,"%d", minutes);
ss.speak(tmp);
delay(100);
ss.speak(F("minutes left"));
}
delay(1000);
}
}
ss.speak(F("Time finished"));
delay (1000);
ss.speak(F("you can setup a new timer now"));
tm1637.colonOn();
tm1637.display("0000");
minutes = 0;
tm1637.colonOn();
delay(100);
} // doubleClick
void longPressStart()
{
Serial.println("long Press");
minutes = 0;
ss.speak(F("Counter reset"));
tm1637.colonOn();
tm1637.display("0000");
tm1637.colonOn();
delay(100);
} // longPressStart
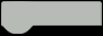