
How to Create a Solar-Powered Balcony Plant Soil Moisture Monitoring System with ESP32-C6
This tutorial will guide you through creating a sustainable, low-power balcony plant monitoring system using the FireBeetle ESP32-C6, a soil sensor, a solar panel, and a lithium battery. The system collects real-time soil moisture data and wirelessly transmits it to the user for remote monitoring and alerts. This comprehensive guide covers everything from hardware selection and circuit design to software development and data analysis, helping urban gardeners efficiently care for their plants.
Hardware Connections
Data Collection and Processing
The ESP32 microcontroller handles data collection, processing, and wireless transmission. Key functions include:
1. Battery Voltage Collection: Use the IO0 pin to read battery voltage and convert it to a digital signal via ADC.
2. Soil Moisture Collection: Use the IO3 pin to read soil moisture sensor voltage and convert it to a digital signal via ADC.
3. Data Processing: Convert digital signals to actual voltage values and moisture percentages.
4. Data Reporting: Connect to a WiFi network and send data to a server via HTTP for remote monitoring.
The sample code:
#include <WiFi.h>
#include <HTTPClient.h>
// #define DATA_SEND_INTERVAL_MS 15 * 60 * 1000
#define DATA_SEND_INTERVAL_MS 60 * 1000
int lastDataSentTimestamp = 0;
unsigned long previousMillis = 0;
unsigned long interval = 30000;
// WiFi network information
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
// Server URL
const char* serverName = "http://192.168.1.236:5000/update";
const char* serverToken = "your_influx_token";
// Define ADC pins
const int batteryPin = 0; // IO0
const int soilMoisturePin = 3; // IO3
void sendData() {
// Collect battery voltage
int batteryVoltage = analogReadMilliVolts(batteryPin)*2;
Serial.print("BAT millivolts value = ");
Serial.print(batteryVoltage);
Serial.println("mV");
// Collect soil sensor voltage
int soilMoisturePercent = analogReadMilliVolts(soilMoisturePin);
Serial.print("Soil sensor voltage = ");
Serial.print(batteryVoltage);
Serial.println("mV");
Serial.println("--------------");
// Create HTTP client
if (WiFi.status() == WL_CONNECTED) {
HTTPClient http;
http.begin(serverName);
http.addHeader("Content-Type", "text/plain");
http.addHeader("authorization", serverToken);
// Create POST data
String httpRequestData = "batteryVoltage=" + String(batteryVoltage) + "&soilMoisture=" + String(soilMoisturePercent);
// Send HTTP POST request
int httpResponseCode = http.POST(httpRequestData);
// Print response result
if (httpResponseCode > 0) {
String response = http.getString();
Serial.println(httpResponseCode);
Serial.println(response);
} else {
Serial.print("Error on sending POST: ");
Serial.println(httpResponseCode);
}
// End HTTP request
http.end();
}
}
void setup() {
Serial.begin(115200);
// Set the resolution to 12 bits (0-4096)
analogReadResolution(12);
// Connect to WiFi
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
Serial.print("IP address: ");
Serial.println(WiFi.localIP());
sendData();
}
void loop() {
unsigned long currentMillis = millis();
// if WiFi is down, try reconnecting
if ((WiFi.status() != WL_CONNECTED) && (currentMillis - previousMillis >= interval)) {
Serial.print(millis());
Serial.println("Reconnecting to WiFi...");
WiFi.disconnect();
WiFi.reconnect();
previousMillis = currentMillis;
}
if (millis() > lastDataSentTimestamp + DATA_SEND_INTERVAL_MS) {
sendData();
Serial.print("Millis since last measurement: ");
Serial.println(millis() - lastDataSentTimestamp);
lastDataSentTimestamp = millis();
}
}
Data Viewing Methods Server Setup
On your computer, follow these steps:
1. Install Python 3.
2. Install Flask:
- Press Win+R, type cmd, and open the command prompt.
- Enter pip install flask to install Flask.
3. Run the Python code in the command line.
from flask import Flask, request, jsonify
app = Flask(__name__)
# Endpoint to handle data from Arduino
@app.route('/update', methods=['POST'])
def update():
auth_header = request.headers.get('authorization')
if auth_header == 'your_influx_token':
data = request.data.decode('utf-8')
print(f"Received data: {data}")
# Process the data sent from Arduino here, such as storing it in a database
return jsonify({"status": "success", "message": "Data received"}), 200
else:
return jsonify({"status": "error", "message": "Unauthorized"}), 401
@app.route('/')
def home():
return "Hello from Flask server!", 200
if __name__ == '__main__':
app.run(host='0.0.0.0', port=5000)
Now, you can check the data:

Debugging and Running
- Ensure Proper Hardware Connections: Connect the FireBeetle ESP32-C6, soil moisture sensor, solar panel, and lithium battery according to the circuit diagram.
- Set WiFi Information: Enter the correct WiFi name and password in the code.
- Server Configuration: Ensure the server-side code runs correctly and enter the correct server address and authorization token.
- Initial Run: Troubleshoot potential issues like unstable hardware connections or code errors. Check each step carefully to ensure solid connections and correct code.
- View Serial Monitor: Use the serial monitor to view ESP32-C6 outputs, aiding in debugging and troubleshooting.
Project Expansion
The onboard GDI interface allows for easy connection to screens, ensuring efficient data visualization. Detailed screen lists and configuration tutorials are provided to facilitate seamless integration of ESP32-C6 functionalities into any setup.
DFRobot's all Gravity and Fermion series sensors are compatible with the FireBeetle 2 ESP32-C6, offering a comprehensive selection for various projects.
Conclusion
This project provides a practical solution for monitoring balcony plants using the ESP32 microcontroller, soil moisture sensor, solar panel, and lithium battery. The system offers real-time environmental data and intelligent alerts, helping users efficiently care for their plants. The successful implementation shows the system's potential to improve balcony plant maintenance.
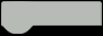