Hi,
I've been tinkering with the DF2301Q Voice Recognition Module (VRM) and want to use it to control a basic LED circuit. For me, the variable "CMDID" is supposed to equal the command ID number listed on the product website (this is what allows the arduino to identify and respond to various command phrases).
So far, it has only ever equal to 0, meaning that my verbal commands are being processed as invalid despite the VRM audibly responding to them.
The module verbally responds to all my commands, but it isn't communicating with my ARDUINO UNO R3 by changing the command ID to be 103 or 104 (lights off) or anything else for that matter.
I need help; why isn't my Arduino repsonding to the VRM?
Other notes:
-Voice Module connections
(Voice module connection point) >> (ARDUINO UNO R3 connection point)
D/T >> A5
C/R >> A4
GND >> GND
VCC >> 5V pin
-Sorry, I'm still kind of new to coding so I don't know the etiquette; hopefully I provided enough context. I would be more than happy to answer questions.
#include "DFRobot_DF2301Q.h"
#define Led 8
// Not sure what these do
#if (defined(ARDUINO_AVR_UNO) || defined(ESP8266)) // Use software serial
SoftwareSerial softSerial(/*rx =*/4, /*tx =*/5);
DFRobot_DF2301Q_UART asr(/*softSerial =*/&softSerial);
#elif defined(ESP32) // Use the hardware serial with remappable pin: Serial1
DFRobot_DF2301Q_UART asr(/*hardSerial =*/&Serial1, /*rx =*/D3, /*tx =*/D2);
#else // Use hardware serial: Serial1
DFRobot_DF2301Q_UART asr(/*hardSerial =*/&Serial1);
#endif
void setup() {
Serial.begin(115200);
pinMode(Led, OUTPUT);
digitalWrite(Led, LOW);
// Init the sensor
while (!(asr.begin())) {
Serial.println("Communication with device failed, please check connection");
delay(3000);
}
Serial.println("Begin ok!");
//Volume and wakeup settings
asr.settingCMD(DF2301Q_UART_MSG_CMD_SET_MUTE, 0);
asr.settingCMD(DF2301Q_UART_MSG_CMD_SET_VOLUME, 7);
asr.settingCMD(DF2301Q_UART_MSG_CMD_SET_WAKE_TIME, 20);
//asr.settingCMD(DF2301Q_UART_MSG_CMD_SET_ENTERWAKEUP, 0);
}
void loop() {
//set CMDID to equal the voice command ID number
uint8_t CMDID = asr.getCMDID();
switch (CMDID){
case 103:
digitalWrite(Led, HIGH);
Serial.print("Lights on; Command ID = ");
Serial.println(CMDID);
break;
case 104:
digitalWrite(Led, LOW);
Serial.print("Lights off; Command ID = ");
Serial.println(CMDID);
break;
default:
Serial.print("CMDID = ");
Serial.println(CMDID);
break;
}
delay(300);
}
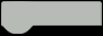