Project Introduction
In this project, we will demonstrate the line tracking function of HuskyLens. We will install HuskyLens to a Devastator tank mobile robot, then HuskyLens will control the robot to perform line tracking.
Press the learning button of HuskyLens, then it can learn and remember the features of an object line. There is no need to adjust parameters again and again, it is easier to use and more powerful than other ordinary sensors.
Other Romeo board and tank robot also can be used, such as follows:
Romeo BLE - Arduino Robot Control Board with Bluetooth 4.0
Devastator Tank Mobile Robot Platform (Metal DC Gear Motor)
Assemble Devastator Tank Mobile Robot
Firstly, we need to assemble the devastator tank, during this process, we need to install ROMEO board to the inner part and connect it to the tank.
Please refer to links below for more details of product, assembling, installation and connection.
Devastator Tank Mobile Robot(Metal DC Gear Motor)
You can find the video, manual and wiki on the product page. ^_^
Wiring

NOTE:
1. We would better connect 4pin sensor cable to ROMEO and reserve the other end, meanwhile, fixing the brackets of HuskyLens with screws and nuts during the process of devastator tank assembly. This can avoid opening the covering of an assembled devastator tank, which is really complex after the assembly.
2. In this project, ROMEO board will use the I2C port to communicate with HuskyLens. Please check the next part "Install HuskyLens" for more details.
Test the Devastator Tank Mobile Robot
Once assembled, we need to test whether the devastator tank is working properly.
Please use the sample code below to test whether the motor is running properly.
Please remove the lithium battery and connect ROMEO board via USB to upload code to avoid contingency.
Please download the example package. Click here to download it.
1. Unpack the Motor Test, enter into library folder, then copy and paste the DFMobile folder (contains DFmobile.h and DFmobile.cpp) to the folder Libraries of your Arduino IDE, then start your Arduino IDE.

2. Use the code below.
/****************************************************
MotorTest
*****************************************************
This example is aimed to control DFMobile basic motion -- Forward and Back
Created 2015-3-2
By Gavin
******************************************************/
#include <DFMobile.h>
DFMobile Robot (4, 5, 7, 6); // initiate the Motor pin
void setup () {
Robot.Direction (LOW, HIGH); // initiate the positive direction
}
void loop () {
Robot.Speed (255, 255); //Forward
delay (1000);
Robot.Speed (-255, -255); //Back
delay (2000);
}
3. Select Arduino board type and corresponding port to upload the code. The board type corresponds to the main control board type. For example, please select Arduino Uno if you are using ROMEO BLE; and for ROMEO V2, you need to select Arduino Leonardo.
4. Once the uploading is accomplished, please unplug the USB cable and plug in a lithium battery to test it. This time, the devastator tank robot will run back and forth. If the motor does not rotate when turning, please check whether the assembly and wiring are correctly achieved.

Install HuskyLens
The position of HuskyLens brackets can be adjusted in real situations.

I2C Communication between ROMEO and HuskyLens

Line Tracking
Please remove the lithium battery and connect ROMEO via USB to upload code to avoid contingency.
1. Download and unpack HUSKYLENS Arduino Library.
Then copy and paste the HuskyLens folder to the folder libraries of your Arduino IDE.
You can also refer to wiki 8.1 to check how to install the library.
2. Start Arduino IDE, open File->Examples->HUSKYLENS->HUSKYLENS_LINE_TRACKING, or just use the sample code below.
/***************************************************
HUSKYLENS An Easy-to-use AI Machine Vision Sensor
<https://www.dfrobot.com/product-1922.html>
***************************************************
This example shows how to play with line tracking.
Created 2020-03-13
By [Angelo qiao]([email protected])
GNU Lesser General Public License.
See <http://www.gnu.org/licenses/> for details.
All above must be included in any redistribution
****************************************************/
/***********Notice and Trouble shooting***************
1.Connection and Diagram can be found here
<https://wiki.dfrobot.com/HUSKYLENS_V1.0_SKU_SEN0305_SEN0336#target_23>
2.This code is tested on Arduino Uno, Leonardo, Mega boards.
****************************************************/
#include "HUSKYLENS.h"
#include "SoftwareSerial.h"
#include "PIDLoop.h"
#include "DFMobile.h"
#define ZUMO_FAST 255
DFMobile Robot (7,6,4,5); // initiate the Motor pin
PIDLoop headingLoop(2000, 0, 0, false);
HUSKYLENS huskylens;
//HUSKYLENS green line >> SDA; blue line >> SCL
int ID1 = 1;
void printResult(HUSKYLENSResult result);
void setup() {
Serial.begin(115200);
Robot.Direction (HIGH, LOW); // initiate the positive direction
Wire.begin();
while (!huskylens.begin(Wire))
{
Serial.println(F("Begin failed!"));
Serial.println(F("1.Please recheck the \"Protocol Type\" in HUSKYLENS (General Settings>>Protol Type>>I2C)"));
Serial.println(F("2.Please recheck the connection."));
delay(100);
}
huskylens.writeAlgorithm(ALGORITHM_LINE_TRACKING); //Switch the algorithm to line tracking.
}
int left = 0, right = 0;
void loop() {
int32_t error;
if (!huskylens.request(ID1)) {Serial.println(F("Fail to request data from HUSKYLENS, recheck the connection!"));left = 0; right = 0;}
else if(!huskylens.isLearned()) {Serial.println(F("Nothing learned, press learn button on HUSKYLENS to learn one!"));left = 0; right = 0;}
else if(!huskylens.available()) Serial.println(F("No block or arrow appears on the screen!"));
else
{
HUSKYLENSResult result = huskylens.read();
printResult(result);
// Calculate the error:
error = (int32_t)result.xTarget - (int32_t)160;
// Perform PID algorithm.
headingLoop.update(error);
// separate heading into left and right wheel velocities.
left = headingLoop.m_command;
right = -headingLoop.m_command;
left += ZUMO_FAST;
right += ZUMO_FAST;
}
Serial.println(String()+left+","+right);
Robot.Speed (left,right);
}
void printResult(HUSKYLENSResult result){
if (result.command == COMMAND_RETURN_BLOCK){
Serial.println(String()+F("Block:xCenter=")+result.xCenter+F(",yCenter=")+result.yCenter+F(",width=")+result.width+F(",height=")+result.height+F(",ID=")+result.ID);
}
else if (result.command == COMMAND_RETURN_ARROW){
Serial.println(String()+F("Arrow:xOrigin=")+result.xOrigin+F(",yOrigin=")+result.yOrigin+F(",xTarget=")+result.xTarget+F(",yTarget=")+result.yTarget+F(",ID=")+result.ID);
}
else{
Serial.println("Object unknown!");
}
}
3. Connect the USB port of ROMEO board to upload the code, then disconnect the USB wire and plug-in a lithium battery, and you will find that devastator tank robot does not move, do not worry, because HuskyLens has not learned the line.
4. Switch HuskyLens to line-tracking mode, then point the “+” at the object line, then short press the "learning button" to complete the learning process. Then release the learning button once the line is learned.
You can also refer to wiki 7.4 for more details of the line-tracking.
5. When HuskyLens detects the line which has been learned, a blue arrow will appear automatically on the screen. The direction of the arrow indicates the predicted direction of the line. Then the devastator tank will move forward and adjust direction according to the predicted direction from HuskyLens, therefore an automatic line-tracking is realized.

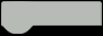