In this experiment, I am going to use DFRobot AS7341 Spectral Sensor to make a simple spectrophotometer, and carry out an experiment to measure substance concentration in different solutions by collecting data, fitting curve, etc.
Background
To begin with, let’s get to know the basics of spectrophotometer and AS7341 spectral sensor.
1. Spectrophotometer
A Spectrophotometer, also known as spectrometer, usually consists of five basic components: the light source, monochromator, sample cell, detector, and signal processing and display system. Since most matters absorb light and the absorbed light varies from different substances, by which we can use a spectrophotometer to measure the amount of the intensity of light absorbed after it passes through sample solution so as to determine the solution concentration.
Light source and monochrometer: tungsten lamps are used as the light sources of a spectrophotometer, and a prism can be used as a monochrometer to refract lights of tungsten lamp to generate continuous chromatography of rainbow colors(red, orange, yellow, green, blue, indigo, violet). But to simplify the experiment, I will use a red LED to simulate light source.
I get a cuvette as the sample cell since it is cheap and easily to be purchased online. The AS7341 Spectral Sensor will be the detector, and a UNO controller board is just right for the signal processing unit.
2. AS7341 Spectral Sensor
The AS7341 Visible Light Sensor employs the new generation of AS7341 spectral sensor IC launched by the well-known AMS company. The sensor features eight channels for the visible light, one channel for near-IR, and one channel without a filter. Also it integrates a dedicated channel to detect ambient light flicker. Besides that, this sensor comes with 6 independent 16-bit ADC channels for data processing in parallel. The sensor can detect visible light in 8 wavelength ranges: F1(405-425nm), F2(435-455nm), F3(470-490nm), F4(505-525nm), F5(545-565nm), F6(580-600nm), F7(620-640nm), F8(670-690nm).
3. Assembly
Preparation:
● DFRduino UNO R3 ×1
● DFRobot I/O Expansion Board ×1
● AS7341 Visible Light Sensor ×1
● Red LED ×1
● Cuvette ×1
● Display ×1
● 3D Printed Case ×1
● Beaker ×6
● Dye(or other soluble substance)
The assembly is pretty easy and will be omitted here.


Spectral Sensor Test
Connect the LED and sensor to the board as the diagram below shows, burn the code into the UNO board.

/*!
* @file getData.ino
* @brief Read the values of 10 optical channels of the AS7341 spectral sensor, the more light of a certain wavelength of the light source,
* the greater the corresponding channel value.
*
* @copyright Copyright (c) 2010 DFRobot Co.Ltd (http://www.dfrobot.com)
* @licence The MIT License (MIT)
* @author [fengli]([email protected])
* @version V1.0
* @date 2020-07-16
* @get from https://www.dfrobot.com
* @url https://github.com/DFRobot/DFRobot_AS7341
*/
#include "DFRobot_AS7341.h"
/*!
* @brief Construct the function
* @param pWire IC bus pointer object and construction device, can both pass or not pass parameters, Wire in default.
*/
DFRobot_AS7341 as7341;
void setup(void)
{
Serial.begin(115200);
//Detect if IIC can communicate properly
while (as7341.begin() != 0) {
Serial.println("IIC init failed, please check if the wire connection is correct");
delay(1000);
}
// //Integration time = (ATIME + 1) x (ASTEP + 1) x 2.78µs
// //Set the value of register ATIME, through which the value of Integration time can be calculated. The value represents the time that must be spent during data reading.
// as7341.setAtime(29);
// //Set the value of register ASTEP, through which the value of Integration time can be calculated. The value represents the time that must be spent during data reading.
// as7341.setAstep(599);
// //Set gain value(0~10 corresponds to X0.5,X1,X2,X4,X8,X16,X32,X64,X128,X256,X512)
// as7341.setAGAIN(7);
// //Enable LED
// //as7341.enableLed(true);
// //Set pin current to control brightness (1~20 corresponds to current 4mA,6mA,8mA,10mA,12mA,......,42mA)
// //as7341.controlLed(10);
}
void loop(void)
{
DFRobot_AS7341::sModeOneData_t data1;
DFRobot_AS7341::sModeTwoData_t data2;
//Start spectrum measurement
//Channel mapping mode: 1.eF1F4ClearNIR,2.eF5F8ClearNIR
as7341.startMeasure(as7341.eF1F4ClearNIR);
//Read the value of sensor data channel 0~5, under eF1F4ClearNIR
data1 = as7341.readSpectralDataOne();
Serial.print("F1(405-425nm):");
Serial.println(data1.ADF1);
Serial.print("F2(435-455nm):");
Serial.println(data1.ADF2);
Serial.print("F3(470-490nm):");
Serial.println(data1.ADF3);
Serial.print("F4(505-525nm):");
Serial.println(data1.ADF4);
//Serial.print("Clear:");
//Serial.println(data1.ADCLEAR);
//Serial.print("NIR:");
//Serial.println(data1.ADNIR);
as7341.startMeasure(as7341.eF5F8ClearNIR);
//Read the value of sensor data channel 0~5, under eF5F8ClearNIR
data2 = as7341.readSpectralDataTwo();
Serial.print("F5(545-565nm):");
Serial.println(data2.ADF5);
Serial.print("F6(580-600nm):");
Serial.println(data2.ADF6);
Serial.print("F7(620-640nm):");
Serial.println(data2.ADF7);
Serial.print("F8(670-690nm):");
Serial.println(data2.ADF8);
Serial.print("Clear:");
Serial.println(data2.ADCLEAR);
Serial.print("NIR:");
Serial.println(data2.ADNIR);
delay(1000);
}
When there is nothing in the Cuvette, open the serial monitor, we can see that the data in F7 wavelength is the highest. So we use the light intensity of F7 as a reference.

Experiment
Then we will collect several groups of data in the experiment. Prepare 6 beakers and some dyes.
Step 1. Pour 100ml water into the six beakers.

Step 2. Add dyes into the beakers: not add to the first cup, one drop for the second cup, two drops for the third cup, and so on.

Step 3. Stir the solutions, and then pour them into the Cuvette for measurement. To get accurate data, clean the Cuvette every time we change the solution. Record the collected data in Excel.


Data Analysis
From the data we can see that as the dye concentration rises, the intensity of F7 becomes lower. If we assume that each drop of dye will increase the concentration by 15%, so the data of the 6 solutions can be fitted into a concentration curve of 0% - 75%. Next, we will fit this curve in Excel.
1) Select the data in rows of concentration and F7, and create a scatter diagram based on these data.

2) Right-click any point in the scatter chart, select “Add trend line”, and select the curve that best fits the data points. Finally, display the formula. So the final formula can be obtained. My formula is: y = 2e-06x2 - 0.0257x + 70.525.

Display Unknown Concentration
Add the formula above into the code: outdata=(0.000002data2.ADF7data2.ADF7-0.0257data2.ADF7+70.525);
Add the library of the display and the revised code. All the codes and necessary libraries are attached to the end of the article.
#include "DFRobot_AS7341.h"
#include <DFRobot_SSD1306_I2C.h>
const chCode chBuf[] = {
{0x6d53,0xe6b593,0xC5A8,{0x00,0x40,0x20,0x40,0x10,0x40,0x17,0xfc,0x84,0x84,0x48,0x88,0x41,0x40,0x11,0x44,0x13,0x48,0x25,0x30,0xe9,0x20,0x21,0x10,0x21,0x08,0x21,0x44,0x21,0x82,0x01,0x00}},
{0x5ea6,0xe5baa6,0xB6C8,{0x01,0x00,0x00,0x80,0x3f,0xfe,0x22,0x20,0x22,0x20,0x3f,0xfc,0x22,0x20,0x22,0x20,0x23,0xe0,0x20,0x00,0x2f,0xf0,0x24,0x10,0x42,0x20,0x41,0xc0,0x86,0x30,0x38,0x0e}}
};
DFRobot_AS7341 as7341;
DFRobot_SSD1306_I2C oled12864;
double outdata;
void setup(void)
{
Serial.begin(115200);
oled12864.begin(0x3c);
//Detect if IIC can communicate properly
while (as7341.begin() != 0) {
Serial.println("IIC init failed, please check if the wire connection is correct");
delay(1000);
}
}
void loop(void)
{
DFRobot_AS7341::sModeOneData_t data1;
DFRobot_AS7341::sModeTwoData_t data2;
//Start spectrum measurement
//Channel mapping mode: 1.eF1F4ClearNIR,2.eF5F8ClearNIR
as7341.startMeasure(as7341.eF1F4ClearNIR);
//Read the value of sensor data channel 0~5 under eF1F4ClearNIR mode
data1 = as7341.readSpectralDataOne();
Serial.print("F1(405-425nm):");
Serial.println(data1.ADF1);
Serial.print("F2(435-455nm):");
Serial.println(data1.ADF2);
Serial.print("F3(470-490nm):");
Serial.println(data1.ADF3);
Serial.print("F4(505-525nm):");
Serial.println(data1.ADF4);
//Serial.print("Clear:");
//Serial.println(data1.ADCLEAR);
//Serial.print("NIR:");
//Serial.println(data1.ADNIR);
as7341.startMeasure(as7341.eF5F8ClearNIR);
//Read the value of sensor data channel 0~5 under eF5F8ClearNIR mode
data2 = as7341.readSpectralDataTwo();
Serial.print("F5(545-565nm):");
Serial.println(data2.ADF5);
Serial.print("F6(580-600nm):");
Serial.println(data2.ADF6);
Serial.print("F7(620-640nm):");
Serial.println(data2.ADF7);
Serial.print("F8(670-690nm):");
Serial.println(data2.ADF8);
Serial.print("Clear:");
Serial.println(data2.ADCLEAR);
Serial.print("NIR:");
Serial.println(data2.ADNIR);
delay(1000);
outdata=(0.000002*data2.ADF7*data2.ADF7-0.0257*data2.ADF7+70.525);
Serial.print("Concentration:");
Serial.println(outdata);
oled12864.setChCode(chBuf);
oled12864.setCursor(1, 1);
oled12864.print("Concentration");
oled12864.setCursor(110, 1);
oled12864.print("%");
oled12864.setCursor(40, 1);
oled12864.print(outdata);
}
Randomly mix a solution and pour it into the cuvette.

Put the cuvette into our device, then the solution concentration will be displayed on the screen.

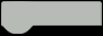