In this tutorial we are going to interface the Huskylens with Raspberry Pi Pico and program the Pico.
Huskylens is an AI camera from dfrobot.com. It has many features like face recognition, object recognition, object tracking, color recognition, line tracking. Unlike other AI camera solutions Huskylens is very handy. It can be trained just by click of button or few lines of codes supported in python, micropython and Arduino language.
More about Huskylens: https://wiki.dfrobot.com/HUSKYLENS_V1.0_SKU_SEN0305_SEN0336
This tutorial and code is applicable for Face recognition mode, object recognition mode and color recognition mode of huskylens.
Note: Before getting started do know about the Raspberry Pi Pico and Thony IDE as we are going to use Thony IDE for programming the Pico.
Tutorial I referred: https://www.youtube.com/watch?v=zlKJ5hvfs6s&t=633s
Circuit Connection
* Make all the connections as shown in circuit diagram.

*Set the Huskylens to I2C protocol.
*Follow for detail instruction: https://wiki.dfrobot.com/HUSKYLENS_V1.0_SKU_SEN0305_SEN0336
Code
*Copy the code given below and save as huskylensPythonLibrary.py in the Raspberry Pi Pico directory
#Library credits: RRoy https://community.dfrobot.com/makelog-310469.html
import ubinascii
import time
from machine import UART,I2C,Pin
commandHeaderAndAddress = "55AA11"
algorthimsByteID = {
"ALGORITHM_OBJECT_TRACKING": "0100",
"ALGORITHM_FACE_RECOGNITION": "0000",
"ALGORITHM_OBJECT_RECOGNITION": "0200",
"ALGORITHM_LINE_TRACKING": "0300",
"ALGORITHM_COLOR_RECOGNITION": "0400",
"ALGORITHM_TAG_RECOGNITION": "0500",
"ALGORITHM_OBJECT_CLASSIFICATION": "0600"
}
COMMAND_REQUEST_CUSTOMNAMES= 0x2f
COMMAND_REQUEST_TAKE_PHOTO_TO_SD_CARD = 0x30
COMMAND_REQUEST_SAVE_MODEL_TO_SD_CARD = 0x32
COMMAND_REQUEST_LOAD_MODEL_FROM_SD_CARD = 0x33
COMMAND_REQUEST_CUSTOM_TEXT = 0x34
COMMAND_REQUEST_CLEAR_TEXT = 0x35
COMMAND_REQUEST_LEARN_ONECE = 0x36
COMMAND_REQUEST_FORGET = 0x37
COMMAND_REQUEST_SCREENSHOT_TO_SD_CARD = 0x39
COMMAND_REQUEST_FIRMWARE_VERSION = 0x3C
class HuskyLensLibrary:
def __init__(self, proto):
self.proto=proto
self.address=0x32
if(self.proto=="SERIAL"):
self.huskylensSer = UART(2,baudrate=9600,rx=33,tx=32,timeout=100)
else :
self.huskylensSer = I2C(0, scl=Pin(5), sda=Pin(4), freq=100000)
self.lastCmdSent = ""
def writeToHuskyLens(self, cmd):
self.lastCmdSent = cmd
if(self.proto=="SERIAL"):
self.huskylensSer.write(cmd)
else :
self.huskylensSer.writeto(self.address, cmd)
def calculateChecksum(self, hexStr):
total = 0
for i in range(0, len(hexStr), 2):
total += int(hexStr[i:i+2], 16)
hexStr = hex(total)[-2:]
return hexStr
def cmdToBytes(self, cmd):
return ubinascii.unhexlify(cmd)
def splitCommandToParts(self, str):
headers = str[0:4]
address = str[4:6]
data_length = int(str[6:8], 16)
command = str[8:10]
if(data_length > 0):
data = str[10:10+data_length*2]
else:
data = []
checkSum = str[2*(6+data_length-1):2*(6+data_length-1)+2]
return [headers, address, data_length, command, data, checkSum]
def getBlockOrArrowCommand(self):
if(self.proto=="SERIAL"):
byteString = self.huskylensSer.read(5)
byteString += self.huskylensSer.read(int(byteString[3]))
byteString += self.huskylensSer.read(1)
else:
byteString =self.huskylensSer.readfrom(self.address,5)
##print("______")
##print(byteString)
##print(byteString[3])
byteString +=self.huskylensSer.readfrom(self.address,byteString[3]+1)
##print("=======")
##print(byteString)
commandSplit = self.splitCommandToParts(''.join(['%02x' % b for b in byteString]))
return commandSplit[4]
def processReturnData(self):
inProduction = True
if(inProduction):
try:
if(self.proto=="SERIAL"):
byteString = self.huskylensSer.read(5)
byteString += self.huskylensSer.read(int(byteString[3]))
byteString += self.huskylensSer.read(1)
else:
byteString =self.huskylensSer.readfrom(self.address,5)
##print("______")
##print(byteString)
##print(byteString[3])
byteString +=self.huskylensSer.readfrom(self.address,byteString[3]+1)
##print("=======")
##print(byteString)
commandSplit = self.splitCommandToParts(''.join(['%02x' % b for b in byteString]))
if(commandSplit[3] == "2e"):
return "Knock Recieved"
else:
returnData = []
numberOfBlocksOrArrow = int(
commandSplit[4][2:4]+commandSplit[4][0:2], 16)
numberOfIDLearned = int(
commandSplit[4][6:8]+commandSplit[4][4:6], 16)
frameNumber = int(
commandSplit[4][10:12]+commandSplit[4][8:10], 16)
for i in range(numberOfBlocksOrArrow):
returnData.append(self.getBlockOrArrowCommand())
finalData=[]
tmp=[]
for i in returnData:
tmp=[]
for q in range(0,len(i),4):
tmp.append(int(i[q:q+2],16)+int(i[q+2:q+4],16))
finalData.append(tmp)
tmp=[]
return finalData
except:
print("Read error")
return []
def command_request_knock(self):
cmd = self.cmdToBytes(commandHeaderAndAddress+"002c3c")
self.writeToHuskyLens(cmd)
return self.processReturnData()
def command_request(self):
cmd = self.cmdToBytes(commandHeaderAndAddress+"002030")
self.writeToHuskyLens(cmd)
return self.processReturnData()
def command_request_blocks(self):
cmd = self.cmdToBytes(commandHeaderAndAddress+"002131")
self.writeToHuskyLens(cmd)
return self.processReturnData()
def command_request_arrows(self):
cmd = self.cmdToBytes(commandHeaderAndAddress+"002232")
self.writeToHuskyLens(cmd)
return self.processReturnData()
def command_request_learned(self):
cmd = self.cmdToBytes(commandHeaderAndAddress+"002333")
self.writeToHuskyLens(cmd)
return self.processReturnData()
def command_request_blocks_learned(self):
cmd = self.cmdToBytes(commandHeaderAndAddress+"002434")
self.writeToHuskyLens(cmd)
return self.processReturnData()
def command_request_arrows_learned(self):
cmd = self.cmdToBytes(commandHeaderAndAddress+"002535")
self.writeToHuskyLens(cmd)
return self.processReturnData()
def line_tracking_mode(self):
cmd = self.cmdToBytes(commandHeaderAndAddress+"022d030042")
self.writeToHuskyLens(cmd)
return self.processReturnData()
def face_recognition_mode(self):
cmd = self.cmdToBytes(commandHeaderAndAddress+"022d00003f")
self.writeToHuskyLens(cmd)
return self.processReturnData()
def object_tracking_mode(self):
cmd = self.cmdToBytes(commandHeaderAndAddress+"022d010040")
self.writeToHuskyLens(cmd)
return self.processReturnData()
def object_recognition_mode(self):
cmd = self.cmdToBytes(commandHeaderAndAddress+"022d020041")
self.writeToHuskyLens(cmd)
return self.processReturnData()
def color_recognition_mode(self):
cmd = self.cmdToBytes(commandHeaderAndAddress+"022d040043")
self.writeToHuskyLens(cmd)
return self.processReturnData()
def tag_recognition_mode(self):
cmd = self.cmdToBytes(commandHeaderAndAddress+"022d050044")
self.writeToHuskyLens(cmd)
return self.processReturnData()
def command_request_by_id(self, idVal):
idVal = "{:04x}".format(idVal)
idVal = idVal[2:]+idVal[0:2]
cmd = commandHeaderAndAddress+"0226"+idVal
cmd += self.calculateChecksum(cmd)
cmd = self.cmdToBytes(cmd)
self.writeToHuskyLens(cmd)
return self.processReturnData()
def command_request_blocks_by_id(self, idVal):
idVal = "{:04x}".format(idVal)
idVal = idVal[2:]+idVal[0:2]
cmd = commandHeaderAndAddress+"0227"+idVal
cmd += self.calculateChecksum(cmd)
cmd = self.cmdToBytes(cmd)
self.writeToHuskyLens(cmd)
return self.processReturnData()
def command_request_arrows_by_id(self, idVal):
idVal = "{:04x}".format(idVal)
idVal = idVal[2:]+idVal[0:2]
cmd = commandHeaderAndAddress+"0228"+idVal
cmd += self.calculateChecksum(cmd)
cmd = self.cmdToBytes(cmd)
self.writeToHuskyLens(cmd)
return self.processReturnData()
def command_request_algorthim(self, alg):
if alg in algorthimsByteID:
cmd = commandHeaderAndAddress+"022d"+algorthimsByteID[alg]
cmd += self.calculateChecksum(cmd)
cmd = self.cmdToBytes(cmd)
self.writeToHuskyLens(cmd)
return self.processReturnData()
else:
print("INCORRECT ALGORITHIM NAME")
#--------------------- 8.5 update new features
#在哈士奇的屏幕上显示文字
def command_request_custom_text(self, text,x,y):
textLength = len(text)
dataLength = textLength+4
cmd = commandHeaderAndAddress #[0x55,0xAA,0x11] [85, 170, 17]
cmd += "{:02x}".format(dataLength) #length of data [4+len(msg)=6]
#cmd += str(34)#COMMAND_REQUEST_CUSTOM_TEXT = 0x34,[52]
cmd += "{:02x}".format(COMMAND_REQUEST_CUSTOM_TEXT)
#first 4 digits, len,cor_x1,cor_x2,cor_y
#len
cmd += "{:02x}".format(dataLength)
#cor_x1,cor_x2
if x > 255:
data_1 = 0xff
cmd += "{:02x}".format(data_1)
data_2 = x % 256
cmd += "{:02x}".format(data_2)
else:
cmd += "{:02x}".format(0)
cmd += "{:02x}".format(x)
#cor_y
cmd += "{:02x}".format(y)
for char in text:
cmd += "{:02x}".format(ord(char))
cmd += self.calculateChecksum(cmd)
cmd = self.cmdToBytes(cmd)
self.writeToHuskyLens(cmd)
#print("cmd:",cmd)
return self.processReturnData()
#清屏
def command_request_clear_text(self):
cmd = commandHeaderAndAddress#[0x55,0xAA,0x11] [85, 170, 17]
dataLength = 0
cmd += "{:02x}".format(dataLength)
cmd += "{:02x}".format(COMMAND_REQUEST_CLEAR_TEXT)
cmd += self.calculateChecksum(cmd)
cmd = self.cmdToBytes(cmd)
self.writeToHuskyLens(cmd)
return self.processReturnData()
#存照片至SD卡
def command_request_photo(self):
cmd = commandHeaderAndAddress
dataLength = 0
cmd += "{:02x}".format(dataLength)
cmd += "{:02x}".format(COMMAND_REQUEST_TAKE_PHOTO_TO_SD_CARD)
cmd += self.calculateChecksum(cmd)
cmd = self.cmdToBytes(cmd)
self.writeToHuskyLens(cmd)
return self.processReturnData()
#忘记算法
def command_request_forget(self):
cmd = commandHeaderAndAddress
dataLength = 0
cmd += "{:02x}".format(dataLength)
cmd += "{:02x}".format(COMMAND_REQUEST_FORGET)
cmd += self.calculateChecksum(cmd)
cmd = self.cmdToBytes(cmd)
self.writeToHuskyLens(cmd)
return self.processReturnData()
#存截图至SD卡
def command_request_screenshot(self):
cmd = commandHeaderAndAddress
dataLength = 0
cmd += "{:02x}".format(dataLength)
cmd += "{:02x}".format(COMMAND_REQUEST_SCREENSHOT_TO_SD_CARD)
cmd += self.calculateChecksum(cmd)
cmd = self.cmdToBytes(cmd)
self.writeToHuskyLens(cmd)
return self.processReturnData()
#学习id一次
def command_request_learn_once(self,id):
cmd = commandHeaderAndAddress
dataLength = 2
cmd += "{:02x}".format(dataLength)
cmd += "{:02x}".format(COMMAND_REQUEST_LEARN_ONECE)
id = [id & 0xff, (id >> 8) & 0xff]
cmd += "{:02x}".format(id[0])
cmd += "{:02x}".format(id[1])
cmd += self.calculateChecksum(cmd)
cmd = self.cmdToBytes(cmd)
self.writeToHuskyLens(cmd)
return self.processReturnData()
# 自定名id名
def command_request_customnames(self, id, name):
nameLength = len(name)
dataLength = nameLength+3
cmd = commandHeaderAndAddress
cmd += "{:02x}".format(dataLength)
cmd += "{:02x}".format(COMMAND_REQUEST_CUSTOMNAMES)
cmd += "{:02x}".format(id)
cmd += "{:02x}".format(nameLength+1)
for char in name:
cmd += "{:02x}".format(ord(char))
cmd += "{:02x}".format(0)
cmd += self.calculateChecksum(cmd)
cmd = self.cmdToBytes(cmd)
self.writeToHuskyLens(cmd)
return self.processReturnData()
#保存模型到SD卡
def command_request_save_model_to_SD_card(self,index):
cmd = commandHeaderAndAddress
dataLength = 2
cmd += "{:02x}".format(dataLength)
cmd += "{:02x}".format(COMMAND_REQUEST_SAVE_MODEL_TO_SD_CARD)
index = [index & 0xff, (index >> 8) & 0xff]
cmd += "{:02x}".format(index[0])
cmd += "{:02x}".format(index[1])
cmd += self.calculateChecksum(cmd)
cmd = self.cmdToBytes(cmd)
self.writeToHuskyLens(cmd)
return self.processReturnData()
#读取模型到SD卡
def command_request_load_model_from_SD_card(self, index):
cmd = commandHeaderAndAddress
dataLength = 2
cmd += "{:02x}".format(dataLength)
cmd += "{:02x}".format(COMMAND_REQUEST_LOAD_MODEL_FROM_SD_CARD)
index = [index & 0xff, (index >> 8) & 0xff]
cmd += "{:02x}".format(index[0])
cmd += "{:02x}".format(index[1])
cmd += self.calculateChecksum(cmd)
cmd = self.cmdToBytes(cmd)
self.writeToHuskyLens(cmd)
return self.processReturnData()

*Copy the given code and save as st7789py.py in the Raspberry Pi Pico directory.
"""
st7789 tft driver in MicroPython based on devbis' st7789py_mpy module from
https://github.com/devbis/st7789py_mpy.
I added support for display rotation, scrolling and drawing text using 8 and 16
bit wide bitmap fonts with heights that are multiples of 8. Included are 12
bitmap fonts derived from classic pc text mode fonts.
"""
import time
from micropython import const
import ustruct as struct
# commands
ST7789_NOP = const(0x00)
ST7789_SWRESET = const(0x01)
ST7789_RDDID = const(0x04)
ST7789_RDDST = const(0x09)
ST7789_SLPIN = const(0x10)
ST7789_SLPOUT = const(0x11)
ST7789_PTLON = const(0x12)
ST7789_NORON = const(0x13)
ST7789_INVOFF = const(0x20)
ST7789_INVON = const(0x21)
ST7789_DISPOFF = const(0x28)
ST7789_DISPON = const(0x29)
ST7789_CASET = const(0x2A)
ST7789_RASET = const(0x2B)
ST7789_RAMWR = const(0x2C)
ST7789_RAMRD = const(0x2E)
ST7789_PTLAR = const(0x30)
ST7789_VSCRDEF = const(0x33)
ST7789_COLMOD = const(0x3A)
ST7789_MADCTL = const(0x36)
ST7789_VSCSAD = const(0x37)
ST7789_MADCTL_MY = const(0x80)
ST7789_MADCTL_MX = const(0x40)
ST7789_MADCTL_MV = const(0x20)
ST7789_MADCTL_ML = const(0x10)
ST7789_MADCTL_BGR = const(0x08)
ST7789_MADCTL_MH = const(0x04)
ST7789_MADCTL_RGB = const(0x00)
ST7789_RDID1 = const(0xDA)
ST7789_RDID2 = const(0xDB)
ST7789_RDID3 = const(0xDC)
ST7789_RDID4 = const(0xDD)
COLOR_MODE_65K = const(0x50)
COLOR_MODE_262K = const(0x60)
COLOR_MODE_12BIT = const(0x03)
COLOR_MODE_16BIT = const(0x05)
COLOR_MODE_18BIT = const(0x06)
COLOR_MODE_16M = const(0x07)
# Color definitions
BLACK = const(0x0000)
BLUE = const(0x001F)
RED = const(0xF800)
GREEN = const(0x07E0)
CYAN = const(0x07FF)
MAGENTA = const(0xF81F)
YELLOW = const(0xFFE0)
WHITE = const(0xFFFF)
_ENCODE_PIXEL = ">H"
_ENCODE_POS = ">HH"
_DECODE_PIXEL = ">BBB"
_BUFFER_SIZE = const(256)
_BIT7 = const(0x80)
_BIT6 = const(0x40)
_BIT5 = const(0x20)
_BIT4 = const(0x10)
_BIT3 = const(0x08)
_BIT2 = const(0x04)
_BIT1 = const(0x02)
_BIT0 = const(0x01)
def color565(red, green=0, blue=0):
"""
Convert red, green and blue values (0-255) into a 16-bit 565 encoding.
"""
try:
red, green, blue = red # see if the first var is a tuple/list
except TypeError:
pass
return (red & 0xf8) << 8 | (green & 0xfc) << 3 | blue >> 3
def _encode_pos(x, y):
"""Encode a postion into bytes."""
return struct.pack(_ENCODE_POS, x, y)
def _encode_pixel(color):
"""Encode a pixel color into bytes."""
return struct.pack(_ENCODE_PIXEL, color)
class ST7789():
"""
ST7789 driver class
Args:
spi (spi): spi object
width (int): display width
height (int): display height
reset (pin): reset pin
dc (pin): dc pin
cs (pin): cs pin
backlight(pin): backlight pin
xstart (int): display xstart offset
ystart (int): display ystart offset
rotation (int): display rotation
"""
def __init__(self, spi, width, height, reset, dc, cs=None, backlight=None,
xstart=-1, ystart=-1, rotation=0):
"""
Initialize display.
"""
if (width, height) != (240, 240) and (width, height) != (135, 240):
raise ValueError(
"Unsupported display. Only 240x240 and 135x240 are supported."
)
self._display_width = self.width = width
self._display_height = self.height = height
self.spi = spi
self.reset = reset
self.dc = dc
self.cs = cs
self.backlight = backlight
self._rotation = rotation % 4
self.xstart = xstart
self.ystart = ystart
self.hard_reset()
self.soft_reset()
self.sleep_mode(False)
self._set_color_mode(COLOR_MODE_65K|COLOR_MODE_16BIT)
time.sleep_ms(50)
self.rotation(self._rotation)
self.inversion_mode(True)
time.sleep_ms(10)
self.write(ST7789_NORON)
time.sleep_ms(10)
if backlight is not None:
backlight.value(1)
self.fill(0)
self.write(ST7789_DISPON)
time.sleep_ms(500)
def write(self, command=None, data=None):
"""SPI write to the device: commands and data."""
if self.cs:
self.cs.off()
if command is not None:
self.dc.off()
self.spi.write(bytes([command]))
if data is not None:
self.dc.on()
self.spi.write(data)
if self.cs:
self.cs.on()
def hard_reset(self):
"""
Hard reset display.
"""
if self.cs:
self.cs.off()
if self.reset:
self.reset.on()
time.sleep_ms(50)
if self.reset:
self.reset.off()
time.sleep_ms(50)
if self.reset:
self.reset.on()
time.sleep_ms(150)
if self.cs:
self.cs.on()
def soft_reset(self):
"""
Soft reset display.
"""
self.write(ST7789_SWRESET)
time.sleep_ms(150)
def sleep_mode(self, value):
"""
Enable or disable display sleep mode.
Args:
value (bool): if True enable sleep mode. if False disable sleep
mode
"""
if value:
self.write(ST7789_SLPIN)
else:
self.write(ST7789_SLPOUT)
def inversion_mode(self, value):
"""
Enable or disable display inversion mode.
Args:
value (bool): if True enable inversion mode. if False disable
inversion mode
"""
if value:
self.write(ST7789_INVON)
else:
self.write(ST7789_INVOFF)
def _set_color_mode(self, mode):
"""
Set display color mode.
Args:
mode (int): color mode
COLOR_MODE_65K, COLOR_MODE_262K, COLOR_MODE_12BIT,
COLOR_MODE_16BIT, COLOR_MODE_18BIT, COLOR_MODE_16M
"""
self.write(ST7789_COLMOD, bytes([mode & 0x77]))
def rotation(self, rotation):
"""
Set display rotation.
Args:
rotation (int): 0-Portrait, 1-Landscape, 2-Inverted Portrait,
3-Inverted Landscape
"""
self._rotation = rotation % 4
if self._rotation == 0: # Portrait
madctl = ST7789_MADCTL_RGB
self.width = self._display_width
self.height = self._display_height
if self._display_width == 135:
self.xstart = 52
self.ystart = 40
elif self._rotation == 1: # Landscape
madctl = ST7789_MADCTL_MX | ST7789_MADCTL_MV | ST7789_MADCTL_RGB
self.width = self._display_height
self.height = self._display_width
if self._display_width == 135:
self.xstart = 40
self.ystart = 53
elif self._rotation == 2: # Inverted Portrait
madctl = ST7789_MADCTL_MX | ST7789_MADCTL_MY | ST7789_MADCTL_RGB
self.width = self._display_width
self.height = self._display_height
if self._display_width == 135:
self.xstart = 53
self.ystart = 40
else: # Inverted Landscape
madctl = ST7789_MADCTL_MV | ST7789_MADCTL_MY | ST7789_MADCTL_RGB
self.width = self._display_height
self.height = self._display_width
if self._display_width == 135:
self.xstart = 40
self.ystart = 52
self.write(ST7789_MADCTL, bytes([madctl]))
def _set_columns(self, start, end):
"""
Send CASET (column address set) command to display.
Args:
start (int): column start address
end (int): column end address
"""
if start <= end <= self.width:
self.write(ST7789_CASET, _encode_pos(
start+self.xstart, end + self.xstart))
def _set_rows(self, start, end):
"""
Send RASET (row address set) command to display.
Args:
start (int): row start address
end (int): row end address
"""
if start <= end <= self.height:
self.write(ST7789_RASET, _encode_pos(
start+self.ystart, end+self.ystart))
def set_window(self, x0, y0, x1, y1):
"""
Set window to column and row address.
Args:
x0 (int): column start address
y0 (int): row start address
x1 (int): column end address
y1 (int): row end address
"""
self._set_columns(x0, x1)
self._set_rows(y0, y1)
self.write(ST7789_RAMWR)
def vline(self, x, y, length, color):
"""
Draw vertical line at the given location and color.
Args:
x (int): x coordinate
Y (int): y coordinate
length (int): length of line
color (int): 565 encoded color
"""
self.fill_rect(x, y, 1, length, color)
def hline(self, x, y, length, color):
"""
Draw horizontal line at the given location and color.
Args:
x (int): x coordinate
Y (int): y coordinate
length (int): length of line
color (int): 565 encoded color
"""
self.fill_rect(x, y, length, 1, color)
def pixel(self, x, y, color):
"""
Draw a pixel at the given location and color.
Args:
x (int): x coordinate
Y (int): y coordinate
color (int): 565 encoded color
"""
self.set_window(x, y, x, y)
self.write(None, _encode_pixel(color))
def blit_buffer(self, buffer, x, y, width, height):
"""
Copy buffer to display at the given location.
Args:
buffer (bytes): Data to copy to display
x (int): Top left corner x coordinate
Y (int): Top left corner y coordinate
width (int): Width
height (int): Height
"""
self.set_window(x, y, x + width - 1, y + height - 1)
self.write(None, buffer)
def rect(self, x, y, w, h, color):
"""
Draw a rectangle at the given location, size and color.
Args:
x (int): Top left corner x coordinate
y (int): Top left corner y coordinate
width (int): Width in pixels
height (int): Height in pixels
color (int): 565 encoded color
"""
self.hline(x, y, w, color)
self.vline(x, y, h, color)
self.vline(x + w - 1, y, h, color)
self.hline(x, y + h - 1, w, color)
def fill_rect(self, x, y, width, height, color):
"""
Draw a rectangle at the given location, size and filled with color.
Args:
x (int): Top left corner x coordinate
y (int): Top left corner y coordinate
width (int): Width in pixels
height (int): Height in pixels
color (int): 565 encoded color
"""
self.set_window(x, y, x + width - 1, y + height - 1)
chunks, rest = divmod(width * height, _BUFFER_SIZE)
pixel = _encode_pixel(color)
self.dc.on()
if chunks:
data = pixel * _BUFFER_SIZE
for _ in range(chunks):
self.write(None, data)
if rest:
self.write(None, pixel * rest)
def fill(self, color):
"""
Fill the entire FrameBuffer with the specified color.
Args:
color (int): 565 encoded color
"""
self.fill_rect(0, 0, self.width, self.height, color)
def line(self, x0, y0, x1, y1, color):
"""
Draw a single pixel wide line starting at x0, y0 and ending at x1, y1.
Args:
x0 (int): Start point x coordinate
y0 (int): Start point y coordinate
x1 (int): End point x coordinate
y1 (int): End point y coordinate
color (int): 565 encoded color
"""
steep = abs(y1 - y0) > abs(x1 - x0)
if steep:
x0, y0 = y0, x0
x1, y1 = y1, x1
if x0 > x1:
x0, x1 = x1, x0
y0, y1 = y1, y0
dx = x1 - x0
dy = abs(y1 - y0)
err = dx // 2
if y0 < y1:
ystep = 1
else:
ystep = -1
while x0 <= x1:
if steep:
self.pixel(y0, x0, color)
else:
self.pixel(x0, y0, color)
err -= dy
if err < 0:
y0 += ystep
err += dx
x0 += 1
def vscrdef(self, tfa, vsa, bfa):
"""
Set Vertical Scrolling Definition.
To scroll a 135x240 display these values should be 40, 240, 40.
There are 40 lines above the display that are not shown followed by
240 lines that are shown followed by 40 more lines that are not shown.
You could write to these areas off display and scroll them into view by
changing the TFA, VSA and BFA values.
Args:
tfa (int): Top Fixed Area
vsa (int): Vertical Scrolling Area
bfa (int): Bottom Fixed Area
"""
struct.pack(">HHH", tfa, vsa, bfa)
self.write(ST7789_VSCRDEF, struct.pack(">HHH", tfa, vsa, bfa))
def vscsad(self, vssa):
"""
Set Vertical Scroll Start Address of RAM.
Defines which line in the Frame Memory will be written as the first
line after the last line of the Top Fixed Area on the display
Example:
for line in range(40, 280, 1):
tft.vscsad(line)
utime.sleep(0.01)
Args:
vssa (int): Vertical Scrolling Start Address
"""
self.write(ST7789_VSCSAD, struct.pack(">H", vssa))
def _text8(self, font, text, x0, y0, color=WHITE, background=BLACK):
"""
Internal method to write characters with width of 8 and
heights of 8 or 16.
Args:
font (module): font module to use
text (str): text to write
x0 (int): column to start drawing at
y0 (int): row to start drawing at
color (int): 565 encoded color to use for characters
background (int): 565 encoded color to use for background
"""
for char in text:
ch = ord(char)
if (font.FIRST <= ch < font.LAST
and x0+font.WIDTH <= self.width
and y0+font.HEIGHT <= self.height):
if font.HEIGHT == 8:
passes = 1
size = 8
each = 0
else:
passes = 2
size = 16
each = 8
for line in range(passes):
idx = (ch-font.FIRST)*size+(each*line)
buffer = struct.pack('>64H',
color if font.FONT[idx] & _BIT7 else background,
color if font.FONT[idx] & _BIT6 else background,
color if font.FONT[idx] & _BIT5 else background,
color if font.FONT[idx] & _BIT4 else background,
color if font.FONT[idx] & _BIT3 else background,
color if font.FONT[idx] & _BIT2 else background,
color if font.FONT[idx] & _BIT1 else background,
color if font.FONT[idx] & _BIT0 else background,
color if font.FONT[idx+1] & _BIT7 else background,
color if font.FONT[idx+1] & _BIT6 else background,
color if font.FONT[idx+1] & _BIT5 else background,
color if font.FONT[idx+1] & _BIT4 else background,
color if font.FONT[idx+1] & _BIT3 else background,
color if font.FONT[idx+1] & _BIT2 else background,
color if font.FONT[idx+1] & _BIT1 else background,
color if font.FONT[idx+1] & _BIT0 else background,
color if font.FONT[idx+2] & _BIT7 else background,
color if font.FONT[idx+2] & _BIT6 else background,
color if font.FONT[idx+2] & _BIT5 else background,
color if font.FONT[idx+2] & _BIT4 else background,
color if font.FONT[idx+2] & _BIT3 else background,
color if font.FONT[idx+2] & _BIT2 else background,
color if font.FONT[idx+2] & _BIT1 else background,
color if font.FONT[idx+2] & _BIT0 else background,
color if font.FONT[idx+3] & _BIT7 else background,
color if font.FONT[idx+3] & _BIT6 else background,
color if font.FONT[idx+3] & _BIT5 else background,
color if font.FONT[idx+3] & _BIT4 else background,
color if font.FONT[idx+3] & _BIT3 else background,
color if font.FONT[idx+3] & _BIT2 else background,
color if font.FONT[idx+3] & _BIT1 else background,
color if font.FONT[idx+3] & _BIT0 else background,
color if font.FONT[idx+4] & _BIT7 else background,
color if font.FONT[idx+4] & _BIT6 else background,
color if font.FONT[idx+4] & _BIT5 else background,
color if font.FONT[idx+4] & _BIT4 else background,
color if font.FONT[idx+4] & _BIT3 else background,
color if font.FONT[idx+4] & _BIT2 else background,
color if font.FONT[idx+4] & _BIT1 else background,
color if font.FONT[idx+4] & _BIT0 else background,
color if font.FONT[idx+5] & _BIT7 else background,
color if font.FONT[idx+5] & _BIT6 else background,
color if font.FONT[idx+5] & _BIT5 else background,
color if font.FONT[idx+5] & _BIT4 else background,
color if font.FONT[idx+5] & _BIT3 else background,
color if font.FONT[idx+5] & _BIT2 else background,
color if font.FONT[idx+5] & _BIT1 else background,
color if font.FONT[idx+5] & _BIT0 else background,
color if font.FONT[idx+6] & _BIT7 else background,
color if font.FONT[idx+6] & _BIT6 else background,
color if font.FONT[idx+6] & _BIT5 else background,
color if font.FONT[idx+6] & _BIT4 else background,
color if font.FONT[idx+6] & _BIT3 else background,
color if font.FONT[idx+6] & _BIT2 else background,
color if font.FONT[idx+6] & _BIT1 else background,
color if font.FONT[idx+6] & _BIT0 else background,
color if font.FONT[idx+7] & _BIT7 else background,
color if font.FONT[idx+7] & _BIT6 else background,
color if font.FONT[idx+7] & _BIT5 else background,
color if font.FONT[idx+7] & _BIT4 else background,
color if font.FONT[idx+7] & _BIT3 else background,
color if font.FONT[idx+7] & _BIT2 else background,
color if font.FONT[idx+7] & _BIT1 else background,
color if font.FONT[idx+7] & _BIT0 else background
)
self.blit_buffer(buffer, x0, y0+8*line, 8, 8)
x0 += 8
def _text16(self, font, text, x0, y0, color=WHITE, background=BLACK):
"""
Internal method to draw characters with width of 16 and heights of 16
or 32.
Args:
font (module): font module to use
text (str): text to write
x0 (int): column to start drawing at
y0 (int): row to start drawing at
color (int): 565 encoded color to use for characters
background (int): 565 encoded color to use for background
"""
for char in text:
ch = ord(char)
if (font.FIRST <= ch < font.LAST
and x0+font.WIDTH <= self.width
and y0+font.HEIGHT <= self.height):
if font.HEIGHT == 16:
passes = 2
size = 32
each = 16
else:
passes = 4
size = 64
each = 16
for line in range(passes):
idx = (ch-font.FIRST)*size+(each*line)
buffer = struct.pack('>128H',
color if font.FONT[idx] & _BIT7 else background,
color if font.FONT[idx] & _BIT6 else background,
color if font.FONT[idx] & _BIT5 else background,
color if font.FONT[idx] & _BIT4 else background,
color if font.FONT[idx] & _BIT3 else background,
color if font.FONT[idx] & _BIT2 else background,
color if font.FONT[idx] & _BIT1 else background,
color if font.FONT[idx] & _BIT0 else background,
color if font.FONT[idx+1] & _BIT7 else background,
color if font.FONT[idx+1] & _BIT6 else background,
color if font.FONT[idx+1] & _BIT5 else background,
color if font.FONT[idx+1] & _BIT4 else background,
color if font.FONT[idx+1] & _BIT3 else background,
color if font.FONT[idx+1] & _BIT2 else background,
color if font.FONT[idx+1] & _BIT1 else background,
color if font.FONT[idx+1] & _BIT0 else background,
color if font.FONT[idx+2] & _BIT7 else background,
color if font.FONT[idx+2] & _BIT6 else background,
color if font.FONT[idx+2] & _BIT5 else background,
color if font.FONT[idx+2] & _BIT4 else background,
color if font.FONT[idx+2] & _BIT3 else background,
color if font.FONT[idx+2] & _BIT2 else background,
color if font.FONT[idx+2] & _BIT1 else background,
color if font.FONT[idx+2] & _BIT0 else background,
color if font.FONT[idx+3] & _BIT7 else background,
color if font.FONT[idx+3] & _BIT6 else background,
color if font.FONT[idx+3] & _BIT5 else background,
color if font.FONT[idx+3] & _BIT4 else background,
color if font.FONT[idx+3] & _BIT3 else background,
color if font.FONT[idx+3] & _BIT2 else background,
color if font.FONT[idx+3] & _BIT1 else background,
color if font.FONT[idx+3] & _BIT0 else background,
color if font.FONT[idx+4] & _BIT7 else background,
color if font.FONT[idx+4] & _BIT6 else background,
color if font.FONT[idx+4] & _BIT5 else background,
color if font.FONT[idx+4] & _BIT4 else background,
color if font.FONT[idx+4] & _BIT3 else background,
color if font.FONT[idx+4] & _BIT2 else background,
color if font.FONT[idx+4] & _BIT1 else background,
color if font.FONT[idx+4] & _BIT0 else background,
color if font.FONT[idx+5] & _BIT7 else background,
color if font.FONT[idx+5] & _BIT6 else background,
color if font.FONT[idx+5] & _BIT5 else background,
color if font.FONT[idx+5] & _BIT4 else background,
color if font.FONT[idx+5] & _BIT3 else background,
color if font.FONT[idx+5] & _BIT2 else background,
color if font.FONT[idx+5] & _BIT1 else background,
color if font.FONT[idx+5] & _BIT0 else background,
color if font.FONT[idx+6] & _BIT7 else background,
color if font.FONT[idx+6] & _BIT6 else background,
color if font.FONT[idx+6] & _BIT5 else background,
color if font.FONT[idx+6] & _BIT4 else background,
color if font.FONT[idx+6] & _BIT3 else background,
color if font.FONT[idx+6] & _BIT2 else background,
color if font.FONT[idx+6] & _BIT1 else background,
color if font.FONT[idx+6] & _BIT0 else background,
color if font.FONT[idx+7] & _BIT7 else background,
color if font.FONT[idx+7] & _BIT6 else background,
color if font.FONT[idx+7] & _BIT5 else background,
color if font.FONT[idx+7] & _BIT4 else background,
color if font.FONT[idx+7] & _BIT3 else background,
color if font.FONT[idx+7] & _BIT2 else background,
color if font.FONT[idx+7] & _BIT1 else background,
color if font.FONT[idx+7] & _BIT0 else background,
color if font.FONT[idx+8] & _BIT7 else background,
color if font.FONT[idx+8] & _BIT6 else background,
color if font.FONT[idx+8] & _BIT5 else background,
color if font.FONT[idx+8] & _BIT4 else background,
color if font.FONT[idx+8] & _BIT3 else background,
color if font.FONT[idx+8] & _BIT2 else background,
color if font.FONT[idx+8] & _BIT1 else background,
color if font.FONT[idx+8] & _BIT0 else background,
color if font.FONT[idx+9] & _BIT7 else background,
color if font.FONT[idx+9] & _BIT6 else background,
color if font.FONT[idx+9] & _BIT5 else background,
color if font.FONT[idx+9] & _BIT4 else background,
color if font.FONT[idx+9] & _BIT3 else background,
color if font.FONT[idx+9] & _BIT2 else background,
color if font.FONT[idx+9] & _BIT1 else background,
color if font.FONT[idx+9] & _BIT0 else background,
color if font.FONT[idx+10] & _BIT7 else background,
color if font.FONT[idx+10] & _BIT6 else background,
color if font.FONT[idx+10] & _BIT5 else background,
color if font.FONT[idx+10] & _BIT4 else background,
color if font.FONT[idx+10] & _BIT3 else background,
color if font.FONT[idx+10] & _BIT2 else background,
color if font.FONT[idx+10] & _BIT1 else background,
color if font.FONT[idx+10] & _BIT0 else background,
color if font.FONT[idx+11] & _BIT7 else background,
color if font.FONT[idx+11] & _BIT6 else background,
color if font.FONT[idx+11] & _BIT5 else background,
color if font.FONT[idx+11] & _BIT4 else background,
color if font.FONT[idx+11] & _BIT3 else background,
color if font.FONT[idx+11] & _BIT2 else background,
color if font.FONT[idx+11] & _BIT1 else background,
color if font.FONT[idx+11] & _BIT0 else background,
color if font.FONT[idx+12] & _BIT7 else background,
color if font.FONT[idx+12] & _BIT6 else background,
color if font.FONT[idx+12] & _BIT5 else background,
color if font.FONT[idx+12] & _BIT4 else background,
color if font.FONT[idx+12] & _BIT3 else background,
color if font.FONT[idx+12] & _BIT2 else background,
color if font.FONT[idx+12] & _BIT1 else background,
color if font.FONT[idx+12] & _BIT0 else background,
color if font.FONT[idx+13] & _BIT7 else background,
color if font.FONT[idx+13] & _BIT6 else background,
color if font.FONT[idx+13] & _BIT5 else background,
color if font.FONT[idx+13] & _BIT4 else background,
color if font.FONT[idx+13] & _BIT3 else background,
color if font.FONT[idx+13] & _BIT2 else background,
color if font.FONT[idx+13] & _BIT1 else background,
color if font.FONT[idx+13] & _BIT0 else background,
color if font.FONT[idx+14] & _BIT7 else background,
color if font.FONT[idx+14] & _BIT6 else background,
color if font.FONT[idx+14] & _BIT5 else background,
color if font.FONT[idx+14] & _BIT4 else background,
color if font.FONT[idx+14] & _BIT3 else background,
color if font.FONT[idx+14] & _BIT2 else background,
color if font.FONT[idx+14] & _BIT1 else background,
color if font.FONT[idx+14] & _BIT0 else background,
color if font.FONT[idx+15] & _BIT7 else background,
color if font.FONT[idx+15] & _BIT6 else background,
color if font.FONT[idx+15] & _BIT5 else background,
color if font.FONT[idx+15] & _BIT4 else background,
color if font.FONT[idx+15] & _BIT3 else background,
color if font.FONT[idx+15] & _BIT2 else background,
color if font.FONT[idx+15] & _BIT1 else background,
color if font.FONT[idx+15] & _BIT0 else background
)
self.blit_buffer(buffer, x0, y0+8*line, 16, 8)
x0 += font.WIDTH
def text(self, font, text, x0, y0, color=WHITE, background=BLACK):
"""
Draw text on display in specified font and colors. 8 and 16 bit wide
fonts are supported.
Args:
font (module): font module to use.
text (str): text to write
x0 (int): column to start drawing at
y0 (int): row to start drawing at
color (int): 565 encoded color to use for characters
background (int): 565 encoded color to use for background
"""
if font.WIDTH == 8:
self._text8(font, text, x0, y0, color, background)
else:
self._text16(font, text, x0, y0, color, background)
* First create a folder with name fonts in the Raspberry Pi Pico directory.
*Now copy the given code and save as vga1_16x32.py in the Raspberry Pi Pico→fonts directory.
WIDTH = 16
HEIGHT = 32
FIRST = 0x20
LAST = 0x7f
_FONT = \
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x00\x00\x00\x00\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x7f\xfe\x7f\xfe\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x7f\xfe\x7f\xfe\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x01\x80\x01\x80\x0f\xf0\x0f\xf0\x39\x9c\x39\x9c\x71\x8e\x71\x8e\x71\x80\x71\x80\x39\x80\x39\x80\x0f\xf0\x0f\xf0\x01\x9c\x01\x9c\x01\x8e\x01\x8e\x71\x8e\x71\x8e\x39\x9c\x39\x9c\x0f\xf0\x0f\xf0\x01\x80\x01\x80\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x1e\x1c\x1e\x1c\x1e\x38\x1e\x38\x00\x70\x00\x70\x00\xe0\x00\xe0\x01\xc0\x01\xc0\x03\x80\x03\x80\x07\x00\x07\x00\x0e\x3c\x0e\x3c\x1c\x3c\x1c\x3c\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x07\xc0\x07\xc0\x1c\x70\x1c\x70\x38\x38\x38\x38\x1c\x70\x1c\x70\x07\xc0\x07\xc0\x0f\xce\x0f\xce\x38\xfc\x38\xfc\x70\x78\x70\x78\x70\x78\x70\x78\x38\xfc\x38\xfc\x0f\xce\x0f\xce\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\xe0\x00\xe0\x01\xc0\x01\xc0\x03\x80\x03\x80\x07\x00\x07\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\xe0\x00\xe0\x01\xc0\x01\xc0\x03\x80\x03\x80\x07\x00\x07\x00\x07\x00\x07\x00\x07\x00\x07\x00\x07\x00\x07\x00\x07\x00\x07\x00\x03\x80\x03\x80\x01\xc0\x01\xc0\x00\xe0\x00\xe0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x07\x00\x07\x00\x03\x80\x03\x80\x01\xc0\x01\xc0\x00\xe0\x00\xe0\x00\xe0\x00\xe0\x00\xe0\x00\xe0\x00\xe0\x00\xe0\x00\xe0\x00\xe0\x01\xc0\x01\xc0\x03\x80\x03\x80\x07\x00\x07\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x0e\x38\x0e\x38\x03\xe0\x03\xe0\x3f\xfe\x3f\xfe\x03\xe0\x03\xe0\x0e\x38\x0e\x38\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x3f\xfe\x3f\xfe\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x03\xc0\x03\xc0\x03\xc0\x03\xc0\x03\x80\x03\x80\x07\x00\x07\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x3f\xfe\x3f\xfe\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x03\xc0\x03\xc0\x03\xc0\x03\xc0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x1c\x00\x1c\x00\x38\x00\x38\x00\x70\x00\x70\x00\xe0\x00\xe0\x01\xc0\x01\xc0\x03\x80\x03\x80\x07\x00\x07\x00\x0e\x00\x0e\x00\x1c\x00\x1c\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x07\xe0\x07\xe0\x1c\x38\x1c\x38\x38\x3c\x38\x3c\x38\x7c\x38\x7c\x38\xdc\x38\xdc\x39\x9c\x39\x9c\x3b\x1c\x3b\x1c\x3e\x1c\x3e\x1c\x3c\x1c\x3c\x1c\x1c\x38\x1c\x38\x07\xe0\x07\xe0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x01\xc0\x01\xc0\x03\xc0\x03\xc0\x0f\xc0\x0f\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x0f\xf0\x0f\xf0\x38\x1c\x38\x1c\x00\x0e\x00\x0e\x00\x0e\x00\x0e\x00\x1c\x00\x1c\x00\x70\x00\x70\x01\xc0\x01\xc0\x07\x00\x07\x00\x1c\x00\x1c\x00\x38\x00\x38\x00\x3f\xfe\x3f\xfe\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x0f\xf0\x0f\xf0\x38\x1c\x38\x1c\x00\x0e\x00\x0e\x00\x0e\x00\x0e\x00\x1c\x00\x1c\x01\xf0\x01\xf0\x00\x1c\x00\x1c\x00\x0e\x00\x0e\x00\x0e\x00\x0e\x38\x1c\x38\x1c\x0f\xf0\x0f\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x01\xf0\x01\xf0\x03\xf0\x03\xf0\x07\x70\x07\x70\x0e\x70\x0e\x70\x1c\x70\x1c\x70\x38\x70\x38\x70\x3f\xfc\x3f\xfc\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x3f\xfe\x3f\xfe\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x3f\xf0\x3f\xf0\x00\x1c\x00\x1c\x00\x0e\x00\x0e\x00\x0e\x00\x0e\x00\x0e\x00\x0e\x38\x1c\x38\x1c\x0f\xf0\x0f\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x07\xf0\x07\xf0\x1c\x00\x1c\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x3f\xf0\x3f\xf0\x38\x1c\x38\x1c\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x1c\x1c\x1c\x1c\x07\xf0\x07\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x3f\xf8\x3f\xf8\x00\x38\x00\x38\x00\x38\x00\x38\x00\x70\x00\x70\x00\xe0\x00\xe0\x01\xc0\x01\xc0\x03\x80\x03\x80\x03\x80\x03\x80\x03\x80\x03\x80\x03\x80\x03\x80\x03\x80\x03\x80\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x07\xf0\x07\xf0\x1c\x1c\x1c\x1c\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x1c\x1c\x1c\x1c\x07\xf0\x07\xf0\x1c\x1c\x1c\x1c\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x1c\x1c\x1c\x1c\x07\xf0\x07\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x07\xf0\x07\xf0\x1c\x1c\x1c\x1c\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x1c\x0e\x1c\x0e\x07\xfe\x07\xfe\x00\x0e\x00\x0e\x00\x0e\x00\x0e\x00\x0e\x00\x0e\x00\x1c\x00\x1c\x0f\xf0\x0f\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x03\x80\x03\x80\x03\x80\x03\x80\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x03\x80\x03\x80\x03\x80\x03\x80\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x03\x80\x03\x80\x03\x80\x03\x80\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x03\x80\x03\x80\x03\x80\x03\x80\x07\x00\x07\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\xe0\x00\xe0\x01\xc0\x01\xc0\x03\x80\x03\x80\x07\x00\x07\x00\x0e\x00\x0e\x00\x1c\x00\x1c\x00\x0e\x00\x0e\x00\x07\x00\x07\x00\x03\x80\x03\x80\x01\xc0\x01\xc0\x00\xe0\x00\xe0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x3f\xfc\x3f\xfc\x00\x00\x00\x00\x00\x00\x00\x00\x3f\xfc\x3f\xfc\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x07\x00\x07\x00\x03\x80\x03\x80\x01\xc0\x01\xc0\x00\xe0\x00\xe0\x00\x70\x00\x70\x00\x38\x00\x38\x00\x70\x00\x70\x00\xe0\x00\xe0\x01\xc0\x01\xc0\x03\x80\x03\x80\x07\x00\x07\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x07\xe0\x07\xe0\x1c\x38\x1c\x38\x38\x1c\x38\x1c\x00\x38\x00\x38\x00\x70\x00\x70\x00\xe0\x00\xe0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x00\x00\x00\x00\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x0f\xf0\x0f\xf0\x38\x1c\x38\x1c\x70\x0e\x70\x0e\x71\xfe\x71\xfe\x73\x8e\x73\x8e\x73\x8e\x73\x8e\x73\x8e\x73\x8e\x71\xfc\x71\xfc\x70\x00\x70\x00\x38\x00\x38\x00\x0f\xfc\x0f\xfc\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x03\xc0\x03\xc0\x07\xe0\x07\xe0\x0e\x70\x0e\x70\x1c\x38\x1c\x38\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x3f\xfc\x3f\xfc\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x3f\xf0\x3f\xf0\x38\x1c\x38\x1c\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x1c\x38\x1c\x3f\xf0\x3f\xf0\x38\x1c\x38\x1c\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x1c\x38\x1c\x3f\xf0\x3f\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x07\xf0\x07\xf0\x1c\x1c\x1c\x1c\x38\x0e\x38\x0e\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x0e\x38\x0e\x1c\x1c\x1c\x1c\x07\xf0\x07\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x3f\xf0\x3f\xf0\x38\x1c\x38\x1c\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x1c\x38\x1c\x3f\xf0\x3f\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x3f\xfc\x3f\xfc\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x3f\xe0\x3f\xe0\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x3f\xfc\x3f\xfc\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x3f\xfc\x3f\xfc\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x3f\xe0\x3f\xe0\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x07\xf0\x07\xf0\x1c\x1c\x1c\x1c\x38\x0e\x38\x0e\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x3e\x38\x3e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x1c\x1c\x1c\x1c\x07\xf0\x07\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x3f\xfe\x3f\xfe\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x1c\x00\x1c\x00\x1c\x00\x1c\x00\x1c\x00\x1c\x00\x1c\x00\x1c\x00\x1c\x00\x1c\x00\x1c\x00\x1c\x00\x1c\x00\x1c\x00\x1c\x00\x1c\x1c\x1c\x1c\x1c\x0e\x38\x0e\x38\x03\xe0\x03\xe0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x1c\x38\x1c\x38\x1c\x70\x1c\x70\x1c\xe0\x1c\xe0\x1d\xc0\x1d\xc0\x1f\x80\x1f\x80\x1f\x80\x1f\x80\x1d\xc0\x1d\xc0\x1c\xe0\x1c\xe0\x1c\x70\x1c\x70\x1c\x38\x1c\x38\x1c\x1c\x1c\x1c\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x3f\xfc\x3f\xfc\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x78\x1e\x78\x1e\x7c\x3e\x7c\x3e\x7e\x7e\x7e\x7e\x77\xee\x77\xee\x73\xce\x73\xce\x71\x8e\x71\x8e\x70\x0e\x70\x0e\x70\x0e\x70\x0e\x70\x0e\x70\x0e\x70\x0e\x70\x0e\x70\x0e\x70\x0e\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x38\x0e\x38\x0e\x3c\x0e\x3c\x0e\x3e\x0e\x3e\x0e\x3f\x0e\x3f\x0e\x3b\x8e\x3b\x8e\x39\xce\x39\xce\x38\xee\x38\xee\x38\x7e\x38\x7e\x38\x3e\x38\x3e\x38\x1e\x38\x1e\x38\x0e\x38\x0e\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x07\xf0\x07\xf0\x1c\x1c\x1c\x1c\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x1c\x1c\x1c\x1c\x07\xf0\x07\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x3f\xf0\x3f\xf0\x38\x1c\x38\x1c\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x1c\x38\x1c\x3f\xf0\x3f\xf0\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x07\xf0\x07\xf0\x1c\x1c\x1c\x1c\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\xee\x38\xee\x1c\x7c\x1c\x7c\x07\xf8\x07\xf8\x00\x1c\x00\x1c\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x3f\xf0\x3f\xf0\x38\x1c\x38\x1c\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x1c\x38\x1c\x3f\xf0\x3f\xf0\x38\xe0\x38\xe0\x38\x70\x38\x70\x38\x38\x38\x38\x38\x1c\x38\x1c\x38\x0e\x38\x0e\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x0f\xf0\x0f\xf0\x38\x1c\x38\x1c\x70\x0e\x70\x0e\x70\x00\x70\x00\x38\x00\x38\x00\x0f\xf0\x0f\xf0\x00\x1c\x00\x1c\x00\x0e\x00\x0e\x70\x0e\x70\x0e\x38\x1c\x38\x1c\x0f\xf0\x0f\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x3f\xfe\x3f\xfe\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x1c\x1c\x1c\x1c\x07\xf0\x07\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x1c\x38\x1c\x38\x0e\x70\x0e\x70\x07\xe0\x07\xe0\x03\xc0\x03\xc0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x70\x0e\x70\x0e\x70\x0e\x70\x0e\x70\x0e\x70\x0e\x70\x0e\x70\x0e\x70\x0e\x70\x0e\x70\x0e\x70\x0e\x71\x8e\x71\x8e\x73\xce\x73\xce\x77\xee\x77\xee\x3e\x7c\x3e\x7c\x1c\x38\x1c\x38\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x1c\x38\x1c\x38\x0e\x70\x0e\x70\x07\xe0\x07\xe0\x03\xc0\x03\xc0\x07\xe0\x07\xe0\x0e\x70\x0e\x70\x1c\x38\x1c\x38\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x38\x0e\x38\x0e\x1c\x1c\x1c\x1c\x0e\x38\x0e\x38\x07\x70\x07\x70\x03\xe0\x03\xe0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x3f\xfe\x3f\xfe\x00\x1c\x00\x1c\x00\x38\x00\x38\x00\x70\x00\x70\x00\xe0\x00\xe0\x01\xc0\x01\xc0\x03\x80\x03\x80\x07\x00\x07\x00\x0e\x00\x0e\x00\x1c\x00\x1c\x00\x3f\xfe\x3f\xfe\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x07\xf0\x07\xf0\x07\x00\x07\x00\x07\x00\x07\x00\x07\x00\x07\x00\x07\x00\x07\x00\x07\x00\x07\x00\x07\x00\x07\x00\x07\x00\x07\x00\x07\x00\x07\x00\x07\x00\x07\x00\x07\xf0\x07\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x1c\x00\x1c\x00\x0e\x00\x0e\x00\x07\x00\x07\x00\x03\x80\x03\x80\x01\xc0\x01\xc0\x00\xe0\x00\xe0\x00\x70\x00\x70\x00\x38\x00\x38\x00\x1c\x00\x1c\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x07\xf0\x07\xf0\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x07\xf0\x07\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x03\xc0\x03\xc0\x07\xe0\x07\xe0\x0e\x70\x0e\x70\x1c\x38\x1c\x38\x38\x1c\x38\x1c\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\xff\xff\xff\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x07\x00\x07\x00\x03\x80\x03\x80\x01\xc0\x01\xc0\x00\xe0\x00\xe0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x0f\xf8\x0f\xf8\x00\x0e\x00\x0e\x0f\xfe\x0f\xfe\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x0f\xfe\x0f\xfe\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x3f\xf0\x3f\xf0\x38\x1c\x38\x1c\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x3f\xf8\x3f\xf8\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x0f\xf8\x0f\xf8\x38\x0e\x38\x0e\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x0e\x38\x0e\x0f\xf8\x0f\xf8\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x0e\x00\x0e\x00\x0e\x00\x0e\x00\x0e\x00\x0e\x00\x0e\x00\x0e\x07\xfe\x07\xfe\x1c\x0e\x1c\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x0f\xfe\x0f\xfe\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x0f\xf8\x0f\xf8\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x3f\xfe\x3f\xfe\x38\x00\x38\x00\x38\x00\x38\x00\x0f\xfc\x0f\xfc\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\xf8\x00\xf8\x03\x80\x03\x80\x03\x80\x03\x80\x03\x80\x03\x80\x03\x80\x03\x80\x0f\xf0\x0f\xf0\x03\x80\x03\x80\x03\x80\x03\x80\x03\x80\x03\x80\x03\x80\x03\x80\x03\x80\x03\x80\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x0f\xf8\x0f\xf8\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x0f\xfe\x0f\xfe\x00\x0e\x00\x0e\x00\x0e\x00\x0e\x1f\xf8\x1f\xf8\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x3b\xf8\x3b\xf8\x3c\x0e\x3c\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x00\x00\x00\x00\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x70\x00\x70\x00\x70\x00\x70\x00\x00\x00\x00\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\x70\x00\xe0\x00\xe0\x0f\x80\x0f\x80\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x0e\x00\x0e\x00\x0e\x00\x0e\x00\x0e\x00\x0e\x00\x0e\x00\x0e\x00\x0e\x38\x0e\x38\x0e\x70\x0e\x70\x0e\xe0\x0e\xe0\x0f\xc0\x0f\xc0\x0e\xe0\x0e\xe0\x0e\x70\x0e\x70\x0e\x38\x0e\x38\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x3e\x78\x3e\x78\x39\xce\x39\xce\x39\xce\x39\xce\x39\xce\x39\xce\x39\xce\x39\xce\x39\xce\x39\xce\x39\xce\x39\xce\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x3f\xe0\x3f\xe0\x38\x38\x38\x38\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x07\xf0\x07\xf0\x1c\x1c\x1c\x1c\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x1c\x1c\x1c\x1c\x07\xf0\x07\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x3f\xf0\x3f\xf0\x38\x1c\x38\x1c\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x1c\x38\x1c\x3f\xf0\x3f\xf0\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x07\xfe\x07\xfe\x1c\x0e\x1c\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x1c\x0e\x1c\x0e\x07\xfe\x07\xfe\x00\x0e\x00\x0e\x00\x0e\x00\x0e\x00\x0e\x00\x0e\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x3f\xf0\x3f\xf0\x38\x1c\x38\x1c\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x38\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x0f\xfc\x0f\xfc\x38\x00\x38\x00\x38\x00\x38\x00\x0f\xf8\x0f\xf8\x00\x0e\x00\x0e\x00\x0e\x00\x0e\x1f\xf8\x1f\xf8\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x1f\xfc\x1f\xfc\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x38\x1c\x0f\xfc\x0f\xfc\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x70\x0e\x70\x0e\x38\x1c\x38\x1c\x1c\x38\x1c\x38\x0e\x70\x0e\x70\x07\xe0\x07\xe0\x03\xc0\x03\xc0\x01\x80\x01\x80\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x38\x0e\x39\xce\x39\xce\x3b\xee\x3b\xee\x1f\x7c\x1f\x7c\x0e\x38\x0e\x38\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x1c\x38\x1c\x38\x0e\x70\x0e\x70\x07\xe0\x07\xe0\x03\xc0\x03\xc0\x07\xe0\x07\xe0\x0e\x70\x0e\x70\x1c\x38\x1c\x38\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x38\x0e\x38\x0e\x1c\x1c\x1c\x1c\x0e\x38\x0e\x38\x07\x70\x07\x70\x03\xe0\x03\xe0\x01\xc0\x01\xc0\x03\x80\x03\x80\x07\x00\x07\x00\x0e\x00\x0e\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x3f\xfe\x3f\xfe\x00\x1c\x00\x1c\x00\x70\x00\x70\x01\xc0\x01\xc0\x07\x00\x07\x00\x1c\x00\x1c\x00\x3f\xfe\x3f\xfe\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\xf8\x00\xf8\x01\xc0\x01\xc0\x03\x80\x03\x80\x03\x80\x03\x80\x03\x80\x03\x80\x1e\x00\x1e\x00\x03\x80\x03\x80\x03\x80\x03\x80\x03\x80\x03\x80\x01\xc0\x01\xc0\x00\xf8\x00\xf8\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x00\x00\x00\x00\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x1f\x00\x1f\x00\x03\x80\x03\x80\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x00\x78\x00\x78\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x01\xc0\x03\x80\x03\x80\x1f\x00\x1f\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x07\x9e\x07\x9e\x3c\xf0\x3c\xf0\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\xc0\x01\xc0\x07\x70\x07\x70\x1c\x1c\x1c\x1c\x70\x07\x70\x07\x70\x07\x70\x07\x7f\xff\x7f\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'\
FONT = memoryview(_FONT)
*Copy the given code and save as vga2_8x8.py in the Raspberry Pi Pico→fonts directory.
"""converted from vga_8x8.bin """
WIDTH = 8
HEIGHT = 8
FIRST = 0x00
LAST = 0xff
_FONT =\
b'\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x7e\x81\xa5\x81\xbd\x99\x81\x7e'\
b'\x7e\xff\xdb\xff\xc3\xe7\xff\x7e'\
b'\x6c\xfe\xfe\xfe\x7c\x38\x10\x00'\
b'\x10\x38\x7c\xfe\x7c\x38\x10\x00'\
b'\x38\x7c\x38\xfe\xfe\xd6\x10\x38'\
b'\x10\x38\x7c\xfe\xfe\x7c\x10\x38'\
b'\x00\x00\x18\x3c\x3c\x18\x00\x00'\
b'\xff\xff\xe7\xc3\xc3\xe7\xff\xff'\
b'\x00\x3c\x66\x42\x42\x66\x3c\x00'\
b'\xff\xc3\x99\xbd\xbd\x99\xc3\xff'\
b'\x0f\x07\x0f\x7d\xcc\xcc\xcc\x78'\
b'\x3c\x66\x66\x66\x3c\x18\x7e\x18'\
b'\x3f\x33\x3f\x30\x30\x70\xf0\xe0'\
b'\x7f\x63\x7f\x63\x63\x67\xe6\xc0'\
b'\x18\xdb\x3c\xe7\xe7\x3c\xdb\x18'\
b'\x80\xe0\xf8\xfe\xf8\xe0\x80\x00'\
b'\x02\x0e\x3e\xfe\x3e\x0e\x02\x00'\
b'\x18\x3c\x7e\x18\x18\x7e\x3c\x18'\
b'\x66\x66\x66\x66\x66\x00\x66\x00'\
b'\x7f\xdb\xdb\x7b\x1b\x1b\x1b\x00'\
b'\x3e\x61\x3c\x66\x66\x3c\x86\x7c'\
b'\x00\x00\x00\x00\x7e\x7e\x7e\x00'\
b'\x18\x3c\x7e\x18\x7e\x3c\x18\xff'\
b'\x18\x3c\x7e\x18\x18\x18\x18\x00'\
b'\x18\x18\x18\x18\x7e\x3c\x18\x00'\
b'\x00\x18\x0c\xfe\x0c\x18\x00\x00'\
b'\x00\x30\x60\xfe\x60\x30\x00\x00'\
b'\x00\x00\xc0\xc0\xc0\xfe\x00\x00'\
b'\x00\x24\x66\xff\x66\x24\x00\x00'\
b'\x00\x18\x3c\x7e\xff\xff\x00\x00'\
b'\x00\xff\xff\x7e\x3c\x18\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00'\
b'\x18\x3c\x3c\x18\x18\x00\x18\x00'\
b'\x66\x66\x24\x00\x00\x00\x00\x00'\
b'\x6c\x6c\xfe\x6c\xfe\x6c\x6c\x00'\
b'\x18\x3e\x60\x3c\x06\x7c\x18\x00'\
b'\x00\xc6\xcc\x18\x30\x66\xc6\x00'\
b'\x38\x6c\x38\x76\xdc\xcc\x76\x00'\
b'\x18\x18\x30\x00\x00\x00\x00\x00'\
b'\x0c\x18\x30\x30\x30\x18\x0c\x00'\
b'\x30\x18\x0c\x0c\x0c\x18\x30\x00'\
b'\x00\x66\x3c\xff\x3c\x66\x00\x00'\
b'\x00\x18\x18\x7e\x18\x18\x00\x00'\
b'\x00\x00\x00\x00\x00\x18\x18\x30'\
b'\x00\x00\x00\x7e\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x18\x18\x00'\
b'\x06\x0c\x18\x30\x60\xc0\x80\x00'\
b'\x38\x6c\xc6\xd6\xc6\x6c\x38\x00'\
b'\x18\x38\x18\x18\x18\x18\x7e\x00'\
b'\x7c\xc6\x06\x1c\x30\x66\xfe\x00'\
b'\x7c\xc6\x06\x3c\x06\xc6\x7c\x00'\
b'\x1c\x3c\x6c\xcc\xfe\x0c\x1e\x00'\
b'\xfe\xc0\xc0\xfc\x06\xc6\x7c\x00'\
b'\x38\x60\xc0\xfc\xc6\xc6\x7c\x00'\
b'\xfe\xc6\x0c\x18\x30\x30\x30\x00'\
b'\x7c\xc6\xc6\x7c\xc6\xc6\x7c\x00'\
b'\x7c\xc6\xc6\x7e\x06\x0c\x78\x00'\
b'\x00\x18\x18\x00\x00\x18\x18\x00'\
b'\x00\x18\x18\x00\x00\x18\x18\x30'\
b'\x06\x0c\x18\x30\x18\x0c\x06\x00'\
b'\x00\x00\x7e\x00\x00\x7e\x00\x00'\
b'\x60\x30\x18\x0c\x18\x30\x60\x00'\
b'\x7c\xc6\x0c\x18\x18\x00\x18\x00'\
b'\x7c\xc6\xde\xde\xde\xc0\x78\x00'\
b'\x38\x6c\xc6\xfe\xc6\xc6\xc6\x00'\
b'\xfc\x66\x66\x7c\x66\x66\xfc\x00'\
b'\x3c\x66\xc0\xc0\xc0\x66\x3c\x00'\
b'\xf8\x6c\x66\x66\x66\x6c\xf8\x00'\
b'\xfe\x62\x68\x78\x68\x62\xfe\x00'\
b'\xfe\x62\x68\x78\x68\x60\xf0\x00'\
b'\x3c\x66\xc0\xc0\xce\x66\x3a\x00'\
b'\xc6\xc6\xc6\xfe\xc6\xc6\xc6\x00'\
b'\x3c\x18\x18\x18\x18\x18\x3c\x00'\
b'\x1e\x0c\x0c\x0c\xcc\xcc\x78\x00'\
b'\xe6\x66\x6c\x78\x6c\x66\xe6\x00'\
b'\xf0\x60\x60\x60\x62\x66\xfe\x00'\
b'\xc6\xee\xfe\xfe\xd6\xc6\xc6\x00'\
b'\xc6\xe6\xf6\xde\xce\xc6\xc6\x00'\
b'\x7c\xc6\xc6\xc6\xc6\xc6\x7c\x00'\
b'\xfc\x66\x66\x7c\x60\x60\xf0\x00'\
b'\x7c\xc6\xc6\xc6\xc6\xce\x7c\x0e'\
b'\xfc\x66\x66\x7c\x6c\x66\xe6\x00'\
b'\x3c\x66\x30\x18\x0c\x66\x3c\x00'\
b'\x7e\x7e\x5a\x18\x18\x18\x3c\x00'\
b'\xc6\xc6\xc6\xc6\xc6\xc6\x7c\x00'\
b'\xc6\xc6\xc6\xc6\xc6\x6c\x38\x00'\
b'\xc6\xc6\xc6\xd6\xd6\xfe\x6c\x00'\
b'\xc6\xc6\x6c\x38\x6c\xc6\xc6\x00'\
b'\x66\x66\x66\x3c\x18\x18\x3c\x00'\
b'\xfe\xc6\x8c\x18\x32\x66\xfe\x00'\
b'\x3c\x30\x30\x30\x30\x30\x3c\x00'\
b'\xc0\x60\x30\x18\x0c\x06\x02\x00'\
b'\x3c\x0c\x0c\x0c\x0c\x0c\x3c\x00'\
b'\x10\x38\x6c\xc6\x00\x00\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\xff'\
b'\x30\x18\x0c\x00\x00\x00\x00\x00'\
b'\x00\x00\x78\x0c\x7c\xcc\x76\x00'\
b'\xe0\x60\x7c\x66\x66\x66\xdc\x00'\
b'\x00\x00\x7c\xc6\xc0\xc6\x7c\x00'\
b'\x1c\x0c\x7c\xcc\xcc\xcc\x76\x00'\
b'\x00\x00\x7c\xc6\xfe\xc0\x7c\x00'\
b'\x3c\x66\x60\xf8\x60\x60\xf0\x00'\
b'\x00\x00\x76\xcc\xcc\x7c\x0c\xf8'\
b'\xe0\x60\x6c\x76\x66\x66\xe6\x00'\
b'\x18\x00\x38\x18\x18\x18\x3c\x00'\
b'\x06\x00\x06\x06\x06\x66\x66\x3c'\
b'\xe0\x60\x66\x6c\x78\x6c\xe6\x00'\
b'\x38\x18\x18\x18\x18\x18\x3c\x00'\
b'\x00\x00\xec\xfe\xd6\xd6\xd6\x00'\
b'\x00\x00\xdc\x66\x66\x66\x66\x00'\
b'\x00\x00\x7c\xc6\xc6\xc6\x7c\x00'\
b'\x00\x00\xdc\x66\x66\x7c\x60\xf0'\
b'\x00\x00\x76\xcc\xcc\x7c\x0c\x1e'\
b'\x00\x00\xdc\x76\x60\x60\xf0\x00'\
b'\x00\x00\x7e\xc0\x7c\x06\xfc\x00'\
b'\x30\x30\xfc\x30\x30\x36\x1c\x00'\
b'\x00\x00\xcc\xcc\xcc\xcc\x76\x00'\
b'\x00\x00\xc6\xc6\xc6\x6c\x38\x00'\
b'\x00\x00\xc6\xd6\xd6\xfe\x6c\x00'\
b'\x00\x00\xc6\x6c\x38\x6c\xc6\x00'\
b'\x00\x00\xc6\xc6\xc6\x7e\x06\xfc'\
b'\x00\x00\x7e\x4c\x18\x32\x7e\x00'\
b'\x0e\x18\x18\x70\x18\x18\x0e\x00'\
b'\x18\x18\x18\x18\x18\x18\x18\x00'\
b'\x70\x18\x18\x0e\x18\x18\x70\x00'\
b'\x76\xdc\x00\x00\x00\x00\x00\x00'\
b'\x00\x10\x38\x6c\xc6\xc6\xfe\x00'\
b'\x7c\xc6\xc0\xc0\xc6\x7c\x0c\x78'\
b'\xcc\x00\xcc\xcc\xcc\xcc\x76\x00'\
b'\x0c\x18\x7c\xc6\xfe\xc0\x7c\x00'\
b'\x7c\x82\x78\x0c\x7c\xcc\x76\x00'\
b'\xc6\x00\x78\x0c\x7c\xcc\x76\x00'\
b'\x30\x18\x78\x0c\x7c\xcc\x76\x00'\
b'\x30\x30\x78\x0c\x7c\xcc\x76\x00'\
b'\x00\x00\x7e\xc0\xc0\x7e\x0c\x38'\
b'\x7c\x82\x7c\xc6\xfe\xc0\x7c\x00'\
b'\xc6\x00\x7c\xc6\xfe\xc0\x7c\x00'\
b'\x30\x18\x7c\xc6\xfe\xc0\x7c\x00'\
b'\x66\x00\x38\x18\x18\x18\x3c\x00'\
b'\x7c\x82\x38\x18\x18\x18\x3c\x00'\
b'\x30\x18\x00\x38\x18\x18\x3c\x00'\
b'\xc6\x38\x6c\xc6\xfe\xc6\xc6\x00'\
b'\x38\x6c\x7c\xc6\xfe\xc6\xc6\x00'\
b'\x18\x30\xfe\xc0\xf8\xc0\xfe\x00'\
b'\x00\x00\x7e\x18\x7e\xd8\x7e\x00'\
b'\x3e\x6c\xcc\xfe\xcc\xcc\xce\x00'\
b'\x7c\x82\x7c\xc6\xc6\xc6\x7c\x00'\
b'\xc6\x00\x7c\xc6\xc6\xc6\x7c\x00'\
b'\x30\x18\x7c\xc6\xc6\xc6\x7c\x00'\
b'\x78\x84\x00\xcc\xcc\xcc\x76\x00'\
b'\x60\x30\xcc\xcc\xcc\xcc\x76\x00'\
b'\xc6\x00\xc6\xc6\xc6\x7e\x06\xfc'\
b'\xc6\x38\x6c\xc6\xc6\x6c\x38\x00'\
b'\xc6\x00\xc6\xc6\xc6\xc6\x7c\x00'\
b'\x18\x18\x7e\xc0\xc0\x7e\x18\x18'\
b'\x38\x6c\x64\xf0\x60\x66\xfc\x00'\
b'\x66\x66\x3c\x7e\x18\x7e\x18\x18'\
b'\xf8\xcc\xcc\xfa\xc6\xcf\xc6\xc7'\
b'\x0e\x1b\x18\x3c\x18\xd8\x70\x00'\
b'\x18\x30\x78\x0c\x7c\xcc\x76\x00'\
b'\x0c\x18\x00\x38\x18\x18\x3c\x00'\
b'\x0c\x18\x7c\xc6\xc6\xc6\x7c\x00'\
b'\x18\x30\xcc\xcc\xcc\xcc\x76\x00'\
b'\x76\xdc\x00\xdc\x66\x66\x66\x00'\
b'\x76\xdc\x00\xe6\xf6\xde\xce\x00'\
b'\x3c\x6c\x6c\x3e\x00\x7e\x00\x00'\
b'\x38\x6c\x6c\x38\x00\x7c\x00\x00'\
b'\x18\x00\x18\x18\x30\x63\x3e\x00'\
b'\x00\x00\x00\xfe\xc0\xc0\x00\x00'\
b'\x00\x00\x00\xfe\x06\x06\x00\x00'\
b'\x63\xe6\x6c\x7e\x33\x66\xcc\x0f'\
b'\x63\xe6\x6c\x7a\x36\x6a\xdf\x06'\
b'\x18\x00\x18\x18\x3c\x3c\x18\x00'\
b'\x00\x33\x66\xcc\x66\x33\x00\x00'\
b'\x00\xcc\x66\x33\x66\xcc\x00\x00'\
b'\x22\x88\x22\x88\x22\x88\x22\x88'\
b'\x55\xaa\x55\xaa\x55\xaa\x55\xaa'\
b'\x77\xdd\x77\xdd\x77\xdd\x77\xdd'\
b'\x18\x18\x18\x18\x18\x18\x18\x18'\
b'\x18\x18\x18\x18\xf8\x18\x18\x18'\
b'\x18\x18\xf8\x18\xf8\x18\x18\x18'\
b'\x36\x36\x36\x36\xf6\x36\x36\x36'\
b'\x00\x00\x00\x00\xfe\x36\x36\x36'\
b'\x00\x00\xf8\x18\xf8\x18\x18\x18'\
b'\x36\x36\xf6\x06\xf6\x36\x36\x36'\
b'\x36\x36\x36\x36\x36\x36\x36\x36'\
b'\x00\x00\xfe\x06\xf6\x36\x36\x36'\
b'\x36\x36\xf6\x06\xfe\x00\x00\x00'\
b'\x36\x36\x36\x36\xfe\x00\x00\x00'\
b'\x18\x18\xf8\x18\xf8\x00\x00\x00'\
b'\x00\x00\x00\x00\xf8\x18\x18\x18'\
b'\x18\x18\x18\x18\x1f\x00\x00\x00'\
b'\x18\x18\x18\x18\xff\x00\x00\x00'\
b'\x00\x00\x00\x00\xff\x18\x18\x18'\
b'\x18\x18\x18\x18\x1f\x18\x18\x18'\
b'\x00\x00\x00\x00\xff\x00\x00\x00'\
b'\x18\x18\x18\x18\xff\x18\x18\x18'\
b'\x18\x18\x1f\x18\x1f\x18\x18\x18'\
b'\x36\x36\x36\x36\x37\x36\x36\x36'\
b'\x36\x36\x37\x30\x3f\x00\x00\x00'\
b'\x00\x00\x3f\x30\x37\x36\x36\x36'\
b'\x36\x36\xf7\x00\xff\x00\x00\x00'\
b'\x00\x00\xff\x00\xf7\x36\x36\x36'\
b'\x36\x36\x37\x30\x37\x36\x36\x36'\
b'\x00\x00\xff\x00\xff\x00\x00\x00'\
b'\x36\x36\xf7\x00\xf7\x36\x36\x36'\
b'\x18\x18\xff\x00\xff\x00\x00\x00'\
b'\x36\x36\x36\x36\xff\x00\x00\x00'\
b'\x00\x00\xff\x00\xff\x18\x18\x18'\
b'\x00\x00\x00\x00\xff\x36\x36\x36'\
b'\x36\x36\x36\x36\x3f\x00\x00\x00'\
b'\x18\x18\x1f\x18\x1f\x00\x00\x00'\
b'\x00\x00\x1f\x18\x1f\x18\x18\x18'\
b'\x00\x00\x00\x00\x3f\x36\x36\x36'\
b'\x36\x36\x36\x36\xff\x36\x36\x36'\
b'\x18\x18\xff\x18\xff\x18\x18\x18'\
b'\x18\x18\x18\x18\xf8\x00\x00\x00'\
b'\x00\x00\x00\x00\x1f\x18\x18\x18'\
b'\xff\xff\xff\xff\xff\xff\xff\xff'\
b'\x00\x00\x00\x00\xff\xff\xff\xff'\
b'\xf0\xf0\xf0\xf0\xf0\xf0\xf0\xf0'\
b'\x0f\x0f\x0f\x0f\x0f\x0f\x0f\x0f'\
b'\xff\xff\xff\xff\x00\x00\x00\x00'\
b'\x00\x00\x76\xdc\xc8\xdc\x76\x00'\
b'\x78\xcc\xcc\xd8\xcc\xc6\xcc\x00'\
b'\xfe\xc6\xc0\xc0\xc0\xc0\xc0\x00'\
b'\x00\x00\xfe\x6c\x6c\x6c\x6c\x00'\
b'\xfe\xc6\x60\x30\x60\xc6\xfe\x00'\
b'\x00\x00\x7e\xd8\xd8\xd8\x70\x00'\
b'\x00\x00\x66\x66\x66\x66\x7c\xc0'\
b'\x00\x76\xdc\x18\x18\x18\x18\x00'\
b'\x7e\x18\x3c\x66\x66\x3c\x18\x7e'\
b'\x38\x6c\xc6\xfe\xc6\x6c\x38\x00'\
b'\x38\x6c\xc6\xc6\x6c\x6c\xee\x00'\
b'\x0e\x18\x0c\x3e\x66\x66\x3c\x00'\
b'\x00\x00\x7e\xdb\xdb\x7e\x00\x00'\
b'\x06\x0c\x7e\xdb\xdb\x7e\x60\xc0'\
b'\x1e\x30\x60\x7e\x60\x30\x1e\x00'\
b'\x00\x7c\xc6\xc6\xc6\xc6\xc6\x00'\
b'\x00\xfe\x00\xfe\x00\xfe\x00\x00'\
b'\x18\x18\x7e\x18\x18\x00\x7e\x00'\
b'\x30\x18\x0c\x18\x30\x00\x7e\x00'\
b'\x0c\x18\x30\x18\x0c\x00\x7e\x00'\
b'\x0e\x1b\x1b\x18\x18\x18\x18\x18'\
b'\x18\x18\x18\x18\x18\xd8\xd8\x70'\
b'\x00\x18\x00\x7e\x00\x18\x00\x00'\
b'\x00\x76\xdc\x00\x76\xdc\x00\x00'\
b'\x38\x6c\x6c\x38\x00\x00\x00\x00'\
b'\x00\x00\x00\x18\x18\x00\x00\x00'\
b'\x00\x00\x00\x18\x00\x00\x00\x00'\
b'\x0f\x0c\x0c\x0c\xec\x6c\x3c\x1c'\
b'\x6c\x36\x36\x36\x36\x00\x00\x00'\
b'\x78\x0c\x18\x30\x7c\x00\x00\x00'\
b'\x00\x00\x3c\x3c\x3c\x3c\x00\x00'\
b'\x00\x00\x00\x00\x00\x00\x00\x00'\
FONT = memoryview(_FONT)
*It should look something like this


*This code is for training the Huskylens.
*Copy the given code and save with your desired name with .py extension, I saved as learn.py in Raspberry Pi Pico directory.
from huskylensPythonLibrary import HuskyLensLibrary
import time
husky = HuskyLensLibrary("I2C")
for i in range(1000):
husky.command_request_learn_once(1)
time.sleep(0.05)
*In the line husky.command_request_learn_once(1) digit 1 is the ID number(Face ID/Object ID). It should be changed for every other face or object else it will rewrite the existing face/object with new face/object.

*Copy the given code and save in the Raspberry Pi Pico directory as main.py
from huskylensPythonLibrary import HuskyLensLibrary
import time
import uos
import machine
import st7789py as st7789
from fonts import vga2_8x8 as font1
from fonts import vga1_16x32 as font2
import random
# oled display height
husky = HuskyLensLibrary("I2C") ## SERIAL OR I2C
#SPI(1) default pins
spi1_sck=10
spi1_mosi=11
spi1_miso=8 #not use
st7789_res = 12
st7789_dc = 13
st7789_cs = 9
disp_width = 240
disp_height = 240
CENTER_Y = int(disp_width/2)
CENTER_X = int(disp_height/2)
print(uos.uname())
spi1 = machine.SPI(1, baudrate=40000000, polarity=1)
print(spi1)
display = st7789.ST7789(spi1, disp_width, disp_width,
reset=machine.Pin(st7789_res, machine.Pin.OUT),
dc=machine.Pin(st7789_dc, machine.Pin.OUT),
xstart=0, ystart=0, rotation=0)
while True:
result = husky.command_request()
value = ""
for i in range(1):
if result[i][4] == 10:
result[i][4] = 0
elif result[i][4] == 0:
result[i][4] = "?"
value += str(result[i][4])
print(value)
if int(value) == 1:
display.fill(st7789.BLACK)
print("Mr.Bean")
display.text(font2, "Mr.Bean", 10, 100,st7789.GREEN)
time.sleep(1)
print(" ")
if int(value) == 2:
display.fill(st7789.BLACK)
print("Champak Chacha")
display.text(font2, "Champak Chacha", 10, 100,st7789.YELLOW)
time.sleep(1)
print(" ")
if int(value) == 3:
display.fill(st7789.BLACK)
print("Jethiya")
display.text(font2, "Jethiya", 10, 100,st7789.BLUE)
time.sleep(1)
print(" ")
*In this code the face/object will be recognized and it returns the ID numbers.
*Using this ID numbers we can perform different tasks based on our desired requirements.
*In my case I am printing and displaying the names of the persons based on their face ID.

*You can modify the blue highlighted code part as per your requirements.
Note: Before running the main.py make sure that the face/object is in front of the Huskylens or else you will get an error and code wont run.
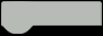