Nice looking and simple to build Retro style Numitron tubes clock.

Things used in this project
Hardware components
Software apps and online services
Hand tools and fabrication machines
Soldering iron (generic)
Solder Wire, Lead Free
Story
This time I will show you how to make a nice Retro style Numitron tubes clock. In addition to the correct time, the device briefly displays the Date, Year, and Current Temperature every 30 seconds.
I got the idea to make this watch from the given Github page: https://github.com/theremotheman/simple_numitron_clock_with_4_shift_registers_and_rtc3231.
I first made the project according to the instructions on the site and found that it contained many flaws.
Here is what the first prototype with 74HC595 looked like

For 74HC595 IC The total maximum current according to the datasheet is 70 milliamperes, which in this case is several times exceeded (about 160 milliamperes for digit 8), so that after a while the IC overheats and does not work properly. Another lack is that there are too many delays in the loop of code so the time is read only once in 60 seconds. In the picture you can see the finished clock made primarily according to the instructions on the page above. In the beginning, it works completely normally, but after a while, random segments are activated and IC-s, numitrons, or the microcontroller can burn very easily. In the first case, the problem was solved by using TPIC6C595 IC instead of 74HC595, which is provided for larger currents. Also should be taken care of that these two integrated circuits are not pin compatible.

----------------------------------------------------------------------------------
https://www.pcbgogo.com/promo/from_MirkoPavleskiMK
-----------------------------------------------------------------------------------
And the new code was created Using millis() Instead of delay() function so now the real-time clock is read constantly. I also added a switch that changes the light intensity of the numitrons, and thus the lifespan. As you can see, the device is relatively simple to build and I think this is the simplest way to make a tube clock. Numitrons are inexpensive, easy to obtain, and do not use an additional high voltage power supply.
Only a few components are needed to make this clock:
- Four Numitron tubes IV9
- Four Integrated circuits TPIC6C595
- Arduino microcontroller
- DS3231 Realtime clock module
- Two LEDs for seconds
- Switch
- and four decoupling capacitors

The real-time module also contains a thermometer, so for a more accurate display of the temperature, it is located outside the box, protected by a mesh. First, it is desirable to synchronize real-time clock with the PC clock, and we do that with the help of the DS1307RTC library. Then we upload the code and with that the device is ready. It remains to modify the code so that we can set the time with the help of buttons and it will be in the next period as a project update.
Finally, the clock is mounted in a suitable box and is a beautiful decoration in every showcase.
Schematics
Schematic diagram

代码
#include <RTClib.h> //rtc with temperature for ds3231
RTC_DS3231 rtc; //setting which rtc module is used
#include <Wire.h> //wiring
#include <TimeLib.h> //time lib
#include <Time.h> //time function
#include <DS1307RTC.h> //rtc
#include <SPI.h>
#define led 7
const int latchPin = 13; //latch
const int clockPin = 10; //clock
const int dataPin = 11; //data
unsigned long previousMillis = 0; // stores last time Led blinked
long interval = 30000; // interval at which to blink (milliseconds)
unsigned long previousMillisDiode = 0; // stores last time Led blinked
long intervalDiode = 500; // interval at which to blink (milliseconds)
const int nums[12] = { //setting display array - according to docs: pin1 is common, pin2 is dot(unused in sketch), rest should be connected to shift registers one by one
0b10111110, //0
0b00000110, //1
0b01111010, //2
0b01101110, //3
0b11000110, //4
0b11101100, //5
0b11111100, //6
0b00001110, //7
0b11111110, //8
0b11101110, //9
0b11001010, //st.
0b10111000 //celz.
};
int hour1; //hour first number
int hour2; //hour second number
int minute1; //minutes first number
int minute2; //minutes second number
int day1; //day first number
int day2; //day second number
int month1; //month first number
int month2; //month second number
int year1; //year first number - constant 2
int year2; //year second number - constant 0 (you wanna live that long to change it?)
int year3; //year third number
int year4; //year fourth number
int hourDecimal; //decimal parsing of hour
int minuteDecimal; //decimal parsing of minute
int dayDecimal; //decimal parsing of day
int monthDecimal; //decimal parsing of month
int year70; //year after unix epoch
int temp1; //first temperature number
int temp2; //second temperature number
int tempDecimal; //decimal parsing of temperature(first two numbers)
void setup() {
pinMode (led,OUTPUT);
pinMode(latchPin, OUTPUT); //set pins to output so you can control the shift register
pinMode(clockPin, OUTPUT); //set pins to output so you can control the shift register
pinMode(dataPin, OUTPUT); //set pins to output so you can control the shift register
Serial.begin(9600);
// initialize SPI:
SPI.begin();
// take the SS pin low to select the chip:
digitalWrite(clockPin,LOW);
}
void loop() {
tmElements_t tm; //naming from DS1307RTC library
RTC.read(tm); // read rtc time/date/year
minuteDecimal = tm.Minute / 10; //parse output to be readable(shorter) by dividing by ten
hourDecimal = tm.Hour / 10; //parse output to be readable(shorter) by dividing by ten
dayDecimal = tm.Day / 10; //parse output to be readable(shorter) by dividing by ten
monthDecimal = tm.Month / 10; //parse output to be readable(shorter) by dividing by ten
year70 = tm.Year - 30; //display real year by subtracting from unix epoch(1970)
hour1 = hourDecimal; //simple as that
hour2 = tm.Hour - 10 * hourDecimal; //make calculations to display only second number from two digits string
minute1 = minuteDecimal; //simple
minute2 = tm.Minute - 10 * minuteDecimal; //make calculations to display only second number from two digits string
day1 = dayDecimal; //simple
day2 = tm.Day - 10 * dayDecimal; //make calculations to display only second number from two digit string
month1 = monthDecimal; //simple
month2 = tm.Month - 10 * monthDecimal; //make calculations to display only second number from two digit string
year1 = 2; //first year number, do you really need to change that? you got flying cars and etc?
year2 = 0; //second year number, if you need to change that you should be playing with grandkids instead
year3 = year70 / 10; //parse output to be readable(shorter) by dividing by ten
year4 = year70 - 10 * year3; //make calculations to display only second number from two digit string
tempDecimal = rtc.getTemperature()/10; //parse output to be readable(shorter) by dividing by ten
temp1 = tempDecimal; //simple
temp2 = rtc.getTemperature() - 10 * tempDecimal; //make calculations to display only second number from two digit string
if (millis() - previousMillisDiode >= intervalDiode) {
previousMillisDiode = millis();
digitalWrite(led, !digitalRead(led)); //change led state
}
if (millis() - previousMillis >= interval) {
previousMillis = millis();
digitalWrite (clockPin, LOW);
SPI.transfer (0b00000000);
SPI.transfer (0b00000000);
SPI.transfer (0b00000000);
SPI.transfer (0b00000000);
digitalWrite (clockPin, HIGH);
delay(500); //numitron tured off for 0.5 sec to make 'breathing' effect
digitalWrite (clockPin, LOW);
SPI.transfer (nums[month2]);
SPI.transfer (nums[month1]);
SPI.transfer (nums[day2]);
SPI.transfer (nums[day1]);
digitalWrite (clockPin, HIGH);
delay(1500);
digitalWrite (clockPin, LOW);
SPI.transfer (0b00000000);
SPI.transfer (0b00000000);
SPI.transfer (0b00000000);
SPI.transfer (0b00000000);
digitalWrite (clockPin, HIGH);
delay(500); //numitron tured off for 0.5 sec to make 'breathing' effect
digitalWrite (clockPin, LOW);
SPI.transfer (nums[year4]);
SPI.transfer (nums[year3]);
SPI.transfer (nums[year2]);
SPI.transfer (nums[year1]);
digitalWrite (clockPin, HIGH);
delay(1500);
digitalWrite (clockPin, LOW);
SPI.transfer (0b00000000);
SPI.transfer (0b00000000);
SPI.transfer (0b00000000);
SPI.transfer (0b00000000);
digitalWrite (clockPin, HIGH);
delay(500); //numitron tured off for 0.5 sec to make 'breathing' effect
digitalWrite (clockPin, LOW);
SPI.transfer (0b10111000);
SPI.transfer (0b11001010);
SPI.transfer (nums[temp2]);
SPI.transfer (nums[temp1]);
digitalWrite (clockPin, HIGH);
delay(1500);
digitalWrite (clockPin, LOW);
SPI.transfer (0b00000000);
SPI.transfer (0b00000000);
SPI.transfer (0b00000000);
SPI.transfer (0b00000000);
digitalWrite (clockPin, HIGH);
delay(500); //numitron tured off for 0.5 sec to make 'breathing' effect
}
else
{
digitalWrite (clockPin, LOW);
SPI.transfer (nums[minute2]);
SPI.transfer (nums[minute1]);
SPI.transfer (nums[hour2]);
SPI.transfer (nums[hour1]);
digitalWrite (clockPin, HIGH);
}
}
The article was first published in hackster, November 20, 2021
cr: https://www.hackster.io/mircemk/diy-simplest-iv9-numitron-clock-with-arduino-5fd16c
author: Mirko Pavleski
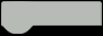