In this article, I will show you how to use DF Robot’s all-new Light and Motor Driver Module for Python in your project.

Things used in this project
Hardware components
Hand tools and fabrication machines
Multitool, Screwdriver
Wire Stripper & Cutter, 32-20 AWG / 0.05-0.5mm² Solid & Stranded Wires
Story
In this article, I will show you how to use DF Robot’s all-new Light and Motor Driver Module for Python in your project. By using this module you can control the speed of a PMDC motor for the brightness of the LED strip.
I use PMDC most of the time in my small projects because it is cheap and very easy to control. I am going to drive my PMDC motor using this Motor Driver Module.
4 Control Modes
There are 4 Control modes of this module
1. Analogue control mode for potentiometer control mode
2. UART or serial communication control mode
3. Host PC software control mode
4. Python Programming control
The main advantage of this driver module is the driver can be controlled via PC using Python programming language. So, this board can be used very easily for those project which is based on Python programming.
Board Description
This module can be supplied from 5 volts to 24 volts DC. And the onboard motor driving MOSFET can handle 10 ampere current. For connecting the power supply and the motor there is a couple of 2 pin screw connector.
There is an onboard micro switch to start and stop the motor. Along with this switch, a male header is also present for connecting external NO-type push switch for the same purpose.
For controlling the speed of the motor manually here is a 3 pin connector where a potentiometer can be connected.
For your or serial communication purpose a 4 pin JST terminal is used. You will also get a 4 pin JST while purchasing this board. The four pins of this JST terminal are used for 5 volts, Ground, TX, and RX.
If you want to communicate with this motor driver via PC there is a USB C type connector on the board. By using a USB C-type data cable you can connect this board to the PC directly. For changing the driving mode is light switch is soldered on the right side of the JST terminal. To put this board into the analog control mode you have to slide the switch in the analog position and for the other three modes f to slide this switch in the communication position.
You can read the original article https://circuitician.com/light-and-motor-driver-for-python/
You can check the video tutorial here
Schematics
Circuit for analog mode

Circuit for UART mode

Code
Automatic Speed control code for arduino
C/C++
#include "SoftwareSerial.h"
#define PWM_ENABLE 0x01
#define PWM_DISENABLE 0x00
#define DEV_ADDR 0x32
#define DUTY_REG_ADDR 0x0006
#define FREQ_REG_ADDR 0x0007
#define PWM_EN_REG_ADDR 0x0008
SoftwareSerial mySoftwareSerial(10, 11); // RX_ARD --> TX LMD, TX_ARD --> RX_LMD
static uint16_t CheckCRC(uint8_t *data, uint8_t len){
uint16_t crc = 0xFFFF;
for(uint8_t pos = 0; pos < len; pos++){
crc ^= (uint16_t)data[pos];
for(uint8_t i = 8; i != 0; i-- ){
if((crc & 0x0001) != 0){
crc >>= 1;
crc ^= 0xA001;
}else{
crc >>= 1;
}
}
}
crc = ((crc & 0x00FF) << 8) | ((crc & 0xFF00) >> 8);
return crc;
}
static void WriteRegValue(uint16_t regAddr, uint16_t value){
uint8_t tempData[8];
uint16_t crc;
tempData[0] = DEV_ADDR;
tempData[1] = 0x06;
tempData[2] = (regAddr >> 8) & 0xFF;
tempData[3] = regAddr & 0xFF;
tempData[4] = (value >> 8) & 0xFF;
tempData[5] = value & 0xFF;
crc = CheckCRC(tempData, 6);
tempData[6] = (crc >> 8) & 0xFF;
tempData[7] = crc & 0xFF;
for(uint8_t i = 0 ;i < 8; i++){
mySoftwareSerial.print((char)tempData[i]);
}
mySoftwareSerial.flush();
}
static void setPwmDuty(uint8_t duty){
WriteRegValue(DUTY_REG_ADDR, (uint16_t)duty);
}
static void setPwmFreq(uint8_t freq){
WriteRegValue(FREQ_REG_ADDR, (uint16_t)freq);
}
static void setPwmEnable(uint8_t pwmStatus){
WriteRegValue(PWM_EN_REG_ADDR, (uint16_t)pwmStatus);
}
void setup() {
// put your setup code here, to run once:
Serial.begin(9600);
mySoftwareSerial.begin(9600);
delay(1000);
setPwmFreq(10);
delay(50);
setPwmDuty(0);
delay(50);
setPwmEnable(PWM_ENABLE);
delay(50);
}
void loop() {
for(int i=15; i<=255; i=i+1){
setPwmDuty(i);
Serial.println(i);
delay(30);
}
delay(5000);
for(int i=255; i>=15; i=i-1){
setPwmDuty(i);
Serial.println(i);
delay(30);
}
delay(5000);
}
pwmC.py
Python
import time
from pinpong.board import Board
from pinpong.libs.dfrobot_dri0050 import DRI0050 # Import DRI0050 library from libs
#Board("RPi").begin() #RPi Linux platform
Board("Win").begin() #windows platform
#pwmd = DRI0050(port="/dev/ttyUSB0") #RPi Linux platform
pwmd = DRI0050(port="COM3") #Windows platform
#print("version=0x{:x}, addr=0x{:x}".format(pwmd.get_version(), pwmd.get_addr()))
#print("pid=0x{:x}, vid=0x{:x}".format(pwmd.get_vid(), pwmd.get_pid()))
pwmres = 0.1
pwmD = 0
while True:
pwmres = 0.1
pwmD = 0
for i in range (0,10):
pwmd.set_freq(1000) #(183HZ-46875HZ)
pwmD = pwmD + pwmres
pwmd.set_duty(pwmD)#(0%-100%)
pwmd.set_enable(1)
time.sleep(0.5)
for i in range (0,10):
pwmd.set_freq(1000) #(183HZ-46875HZ)
pwmD = pwmD - pwmres
pwmd.set_duty(pwmD)#(0%-100%)
pwmd.set_enable(1)
time.sleep(0.5)
pwmd.set_enable(0)
time.sleep(1.5)
The article was first published in hackster, January 23, 2022
cr: https://www.hackster.io/circuiTician/light-motor-driver-module-for-python-9a4f93
author: circuiTician
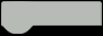