When the soil is dry, Arduino will command the water pump to run. Our plant is absolutely cheerful anytime!

Things used in this project
Hardware components
Story
This is my first project. I love plants, so I created an automatic watering system.
Our system measures the soil moisture and waters automatically according to it. A blue LED tells us when the bucket is empty. BME280 monitors and OLED displays the temperature and humidity in the room. Temporary, it's used indoors only because it needs electrical outlet. In the future, we want to use solar power to run it.
Step1 -Watering-
When the value obtained from the soil moiture sensor falls below 300, the water pump starts to run and waters for plants. "300" indicates that the soil is dry. An outlet supplies power to the pump and the relay module(JQC-3FF-S-Z) regulates the pump. A piezo speaker will let us know that watering is complete. The soil moisture is measured once every 60 seconds.





Step2 -Bucket check-
Use an ultrasonic sensor to know how much water is left in our watering bucket. When the height of the water in the bucket is less than 2cm, a blue LED will turn on. When there is enough water in the bucket, the LED are off.

The ultrasonic sensor measures the distance to the water. The serial monitor shows around 10 cm, which roughly matches the actual distance to water.

Step3 -the growing conditions-
Our system measures the temperature and humidity in the room, and displays these values on an OLED. It also shows the soil moisture.
In this project, I used an OLED with I2C connection. And I used the "I2CLiquidCrystal" library as a reference for that OLED. Download from here.→https://n.mtng.org/ele/arduino/i2c.html
According to the reference site, this library was created by the author with reference to the official Arduino "LiquidCrystal" library. →https://www.arduino.cc/en/Reference/LiquidCrystalConstructor
When using a display, please change the library and code according to the display you are using.


We wish to make more wonderful and useful devices in the future!
This time we used rosemary, which prefers dry conditions rather than wet. We should be careful not to over water it. It smells great. We're very happy.
Writing the sketches in one file makes it difficult to see the wiring, we created a separate file for each step. In practice, we used an Arduino and a breadboard.
Schematics
Step1
Step1. Measure the soil moisture and water with a pump if the soil is dry.

Step2
Step2. An LED tells us when the bucket is empty.

Step3
Step3. Temperature, humidity and soil moisture are measured and displayed on the OLED.

Code
AutoWatering.ino
C/C++
It says a lot, but I'm just measuring the soil humidity and watering it. It's so easy!
#include <Wire.h>
//BME280
#include <Adafruit_BME280.h>
//OLED
#include <I2CLiquidCrystal.h>
//------------------------------
//BME280
#define I2C_SCL 5
#define I2C_SDA 4
Adafruit_BME280 bme;
//OLED
#define I2C_ADDR 0x3c
#define BRIGHT 127
I2CLiquidCrystal oled(I2C_ADDR, (uint8_t)BRIGHT);
//Music
#define BEAT 300
#define PIN 10
#define DO 262
#define RE 294
#define MI 330
#define FA 349
#define SO 392
#define RA 440
#define SI 494
#define HDO 523
//----------------------
//Timer
int counter = 0;
//Ultrasonic Sensor
int Trig = 8;
int Echo = 9;
int Duration;
float Distance;
//LED
int led = 7;
//misture sensor
int water_count = 0;
//relay
int relay = 13;
void setup() {
//BME280
Serial.begin(9600);
Wire.begin();
if (!bme.begin(0x76)) {
Serial.println("Could not find a valid BME280 sensor, check wiring!");
while (1);
}
delay(100);
//OLED
oled.begin(16, 2);
oled.display();
oled.clear();
oled.noBlink();
oled.noCursor();
oled.home();
oled.print("Wait a minutes.");
//Ultrasonic Sensor
pinMode(Trig,OUTPUT);
pinMode(Echo,INPUT);
//LED
pinMode(led,OUTPUT);
//relay
pinMode(relay, OUTPUT);
}
void loop() {
//Check temperature, humidity, and soil humidity once a minute.
if(counter == 1){
ckeckBME280();
checkWater();
checkMoisture();
}
delay(10000);//10sec
counter++;
if(counter >= 6){
counter = 0;
}
checkWater();
}
//BME280
void ckeckBME280(){
// Get data from BME280 sensor
float t = bme.readTemperature(); // C
float h = bme.readHumidity(); // %
float p = bme.readPressure() / 100.0F; // hPa
Serial.print("Temperature:");
Serial.println(t);
Serial.print("Humidity:");
Serial.println(h);
Serial.print("Pressure:");
Serial.println(p);
Serial.println();
oled.setCursor(0, 0);
oled.print(String(t)+"C ");
oled.print(String(h)+"% ");
}
//Ultrasonic Sensor
void checkWater(){
//Check the water level in the bucket.
digitalWrite(Trig,LOW);
delayMicroseconds(1);
digitalWrite(Trig,HIGH);
delayMicroseconds(11);
digitalWrite(Trig,LOW);
Duration = pulseIn(Echo,HIGH);
if (Duration>0) {
Distance = Duration/2;
Distance = Distance*340*100/1000000; // ultrasonic speed is 340m/s = 34000cm/s = 0.034cm/us
Serial.print(Distance);
Serial.println(" cm");
if(Distance > 12){
digitalWrite(led, HIGH);
}else{
digitalWrite(led, LOW);
}
}
}
//moisture sensor
void checkMoisture(){
//Measure soil humidity
int moisture = analogRead(A0);
Serial.print("Moisture Sensor Value:");
Serial.println(moisture);
oled.setCursor(0, 1);
oled.print("Moisture: " + String(moisture) + " ");
if(moisture <= 300){
water_count++;
if(water_count == 5){//To wait for the water to go through the pot.
watering();
water_count = 0;
}
}
}
//Watering
void watering(){
digitalWrite(relay, HIGH);
delay(2000);
digitalWrite(relay, LOW);
delay(8000);
completeWatering();
counter++;
}
//Music
void completeWatering(){
//Let them know that watering is complete.
oled.setCursor(0, 0);
oled.print(" Thank you! ");
oled.setCursor(0, 1);
oled.print(" (^ O ^)/ ");
tone(PIN,DO,BEAT) ; // C
delay(BEAT) ;
tone(PIN,RE,BEAT) ; // D
delay(BEAT) ;
tone(PIN,MI,1200) ; // E
delay(BEAT) ;
delay(BEAT) ;
delay(BEAT) ;
tone(PIN,RE,BEAT) ; // D
delay(BEAT) ;
tone(PIN,DO,BEAT) ; // C
delay(BEAT) ;
delay(BEAT) ;
tone(PIN,DO,BEAT) ; // C
delay(BEAT) ;
tone(PIN,RE,BEAT) ; // D
delay(BEAT) ;
tone(PIN,MI,BEAT) ; // E
delay(BEAT) ;
tone(PIN,RE,BEAT) ; // D
delay(BEAT) ;
tone(PIN,DO,BEAT) ; // C
delay(BEAT) ;
tone(PIN,RE,1200) ; // D
delay(BEAT) ;
delay(BEAT) ;
delay(4400);
counter++;
oled.setCursor(0, 0);
oled.print(" ");
oled.setCursor(0, 1);
oled.print(" ");
ckeckBME280();
int moisture = analogRead(A0);
oled.setCursor(0, 1);
oled.print("Moisture: " + String(moisture) + " ");
}
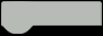