STEP 1
Coaster to care my hot coffee

CODE
#include <DHT.h>
#include <DHT_U.h>
#include <Arduino.h>
#include <TM1637Display.h> // 7SEG display library Include
// Module connection pins (Digital Pins)
#define CLK 2 // Arduino Nano Pin5(D2)
#define DIO 3 // Arduino Nano Pin6(D3)
const int pinLED = 4; // LED output D4
const int pinButton = 5; // Switch input D5
#define DHTPIN 6 // Temp Humidy sensor input D6
// Uncomment whatever type you're using!
//#define DHTTYPE DHT11 // DHT 11
#define DHTTYPE DHT22 // DHT 22 (AM2302), AM2321
//#define DHTTYPE DHT21 // DHT 21 (AM2301)
// Connect pin 1 (on the left) of the sensor to +5V
// NOTE: If using a board with 3.3V logic like an Arduino Due connect pin 1
// to 3.3V instead of 5V!
// Connect pin 2 of the sensor to whatever your DHTPIN is
// Connect pin 4 (on the right) of the sensor to GROUND
// Connect a 10K resistor from pin 2 (data) to pin 1 (power) of the sensor
// Initialize DHT sensor.
// Note that older versions of this library took an optional third parameter to
// tweak the timings for faster processors. This parameter is no longer needed
// as the current DHT reading algorithm adjusts itself to work on faster procs.
DHT dht(DHTPIN, DHTTYPE);
const uint8_t SEG_DONE[] = { //'donE'
SEG_B | SEG_C | SEG_D | SEG_E | SEG_G, // d
SEG_A | SEG_B | SEG_C | SEG_D | SEG_E | SEG_F, // O
SEG_C | SEG_E | SEG_G, // n
SEG_A | SEG_D | SEG_E | SEG_F | SEG_G // E
};
const uint8_t SEG_ON[] = { // '__on'
0, 0, // 2 banlks
SEG_C | SEG_D | SEG_E | SEG_G, // o
SEG_C | SEG_E | SEG_G // n
};
const uint8_t SEG_OFF[] = { // '_oFF'
0, // 1 blank
SEG_C | SEG_D | SEG_E | SEG_G, // o
SEG_A | SEG_E | SEG_F | SEG_G, // F
SEG_A | SEG_E | SEG_F | SEG_G // F
};
const uint8_t SEG_ERR[] = { // '_Err'
0, // 1 balnk
SEG_A | SEG_D | SEG_E | SEG_F | SEG_G, // E
SEG_E | SEG_G, // r
SEG_E | SEG_G // r
};
TM1637Display display(CLK, DIO);
// The amount of time (in milliseconds) between tests
#define TEST_DELAY 2000 // delay 2sec
void setup()
{
pinMode(pinLED, OUTPUT); // Init LED output
pinMode(pinButton, INPUT); // init SW input
Serial.begin(9600); // init serial port
Serial.println(F("Cup Temp!")); // send starting msg to pc
dht.begin(); // init temperature sensor
}
void loop() {
int k;
uint8_t data[] = { 0xff, 0xff, 0xff, 0xff }; // Data to turn on all segment
uint8_t blank[] = { 0x00, 0x00, 0x00, 0x00 }; // Data to turn off all segment
display.setBrightness(0x0f); // max brightness
int ih;
int it;
// All segments on
display.setSegments(data); // turn on all seg.
delay(TEST_DELAY); // dly
display.setSegments(blank); // turn off allseg.
delay(TEST_DELAY); // dly
while (1) { // endlerss loop
digitalWrite(pinLED, HIGH); // LED on
// Reading temperature or humidity takes about 250 milliseconds!
// Sensor readings may also be up to 2 seconds 'old' (its a very slow sensor)
float h = dht.readHumidity();
// Read temperature as Celsius (the default)
float t = dht.readTemperature();
// Read temperature as Fahrenheit (isFahrenheit = true)
float f = dht.readTemperature(true);
digitalWrite(pinLED, LOW); // LED off
// Check if any reads failed and exit early (to try again).
if (isnan(h) || isnan(t) || isnan(f)) { // on error
Serial.println(F("Failed to read from DHT sensor!")); // send error msg via serial port
display.setSegments(SEG_ERR); // display error msg on FND
//return;
}
else {
// Compute heat index in Fahrenheit (the default)
float hif = dht.computeHeatIndex(f, h);
// Compute heat index in Celsius (isFahreheit = false)
float hic = dht.computeHeatIndex(t, h, false);
Serial.print(F("Humidity: ")); // send Temp. Humidy. to PC
Serial.print(h);
Serial.print(F("% Temperature: "));
Serial.print(t);
Serial.print(F("°C "));
Serial.print(f);
Serial.print(F("°F Heat index: "));
Serial.print(hic);
Serial.print(F("°C "));
Serial.print(hif);
Serial.println(F("°F"));
ih = h * 10; // convert floating point value into interger
it = t * 10;
if(digitalRead(pinButton)) { // if sw pressed
display.showNumberDecEx(ih, 0x20, false); // then display humidity
delay(50); // 50ms dly
}
else { // nomaly
display.showNumberDecEx(it, 0x20, false); // display Temperature
delay(50); // 50ms dly
}
}
}
}
License 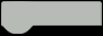
All Rights
Reserved
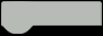
0
More from this category