
In this project we will design a Ph Meter by Interfacing Analog Ph Sensor with Arduino.
Overview
In chemistry, pH is a scale used to specify how acidic or basic a water-based solution is. Acidic solutions have a lower pH, while basic solutions have a higher pH. Thus Ph sensor has the ability to determine the Ph of any solution, i.e it tells whether the substance is acidic, basic or neutral in nature. By knowing the Ph, we can monitor the water quality in Agricultural Farm and also in Fish Farming. Similarly, Ph Sensor has a wide range of applications like wastewater treatment, pharmaceuticals, chemicals & petrochemicals.
In this basic tutorial, we will learn how to interface Gravity Ph Sensor with Arduino. We will design a simple Ph Meter and display the Ph value on OLED/LCD Display. We will also learn about the construction & working of the Ph Sensor. Finally, we will learn the calibration method which will determine the correctness and accuracy of the sensor. The Ph Sensor can also be interfaced with other higher-level microcontrollers like NodeMCU ESP8266 & STM32 which I will discuss in future.
You can add a few more extra sensors to this project like TDS Sensor, Turbidity Sensor & Dissolved Oxygen Sensor for Water Quality Monitoring.
Bill of Materials
Following are the components required for making this project. All the components can be easily purchased from Amazon. The components purchased link is given.
What is Ph?
The term PH is a quantitative measure of the acidity or basicity of aqueous or other liquid solutions. The term, widely used in chemistry, biology, and agronomy, translates the values of the concentration of the hydrogen ion which ordinarily ranges between about 1 and 10−14 gram-equivalents per liter—into numbers between 0 and 14.

In pure water, which is neutral (neither acidic nor alkaline), the concentration of the hydrogen ion is 10−7 gram-equivalents per liter, which corresponds to a pH of 7. A solution with a pH less than 7 is considered acidic; a solution with a pH greater than 7 is considered basic, or alkaline.
What is Ph Meter?
A pH meter is a scientific instrument that measures the hydrogen-ion activity in water-based solutions, indicating its acidity or alkalinity expressed as pH. The pH meter measures the difference in electrical potential between a pH electrode and a reference electrode, and so the pH meter is sometimes referred to as a “potentiometric pH meter“. The difference in electrical potential relates to the acidity or pH of the solution.
Ph Meter Construction & Working
The Ph Sensor has a rod-like structure usually made of glass, with a bulb containing the sensor at the bottom. The glass electrode for measuring the pH has a glass bulb specifically designed to be selective to hydrogen-ion concentration. On immersion in the solution to be tested, hydrogen ions in the test solution exchange for other positively charged ions on the glass bulb, creating an electrochemical potential across the bulb. The electronic amplifier detects the difference in electrical potential between the two electrodes generated in the measurement and converts the potential difference to pH units. The magnitude of the electrochemical potential across the glass bulb is linearly related to the pH according to the Nernst equation.

The reference electrode is insensitive to the pH of the solution, being composed of a metallic conductor, which connects to the display. This conductor is immersed in an electrolyte solution, typically potassium chloride, which comes into contact with the test solution through a porous ceramic membrane. The display consists of a voltmeter, which displays voltage in units of pH.
Gravity Analog Ph Sensor/Meter Kit

DFRobot Gravity: Analog pH meter V2 is specifically designed to measure the pH of the solution and reflect the acidity or alkalinity. As an upgraded version of pH meter V1, the sensor greatly improves the precision and user experience. The onboard voltage regulator chip supports the wide voltage supply of 3.3~5.5V. The output signal filtered by hardware has low jitter. With this Ph Sensor, you can quickly build the pH meter to measure the Ph value of the different aqueous solutions.
Features
-Input Voltage: 3.3~5.5V
-Hardware filtered output signal, low jitter
-Gravity connector and BNC connector
-Easy Calibration
-Uniform size of sensor & connector for easy Mechanical Fitting.
Specifications
The Ph Sensor Kit has Signal Conversion Board (Transmitter) V2 and also pH Probe. Both of them are connected with each other. The features of both of these parts are as follows.
Signal Conversion Board (Transmitter) V2
-Supply Voltage: 3.3~5.5V
-Output Voltage: 0~3.0V
-Probe Connector: BNC
-Signal Connector: PH2.0-3P
-Measurement Accuracy: ±0.1@25℃
-Dimension: 42mm32mm/1.661.26in
pH Probe
-Probe Type: Laboratory Grade
-Detection Range: 0~14
-Temperature Range: 5~60°C
-Zero Point: 7±0.5
-Response Time: <2min
-Internal Resistance: <250MΩ
-Probe Life: >0.5 years (depending on the frequency of use)
-Cable Length: 100cm
To learn more about the Ph Sensor you can check the DfRobot official article here: Gravity: Analog pH Sensor/Meter Kit V2
Interfacing Gravity Analog Ph Sensor with Arduino
Now let us interface Ph Sensor with Arduino using Simple Arduino Code. The connection is fairly simple and circuit/schematic is given below.

Ph Sensor has 3 pins that need to be connected to Arduino. So, connect the VCC pin to 5V of Arduino and GND to GND. Connect its analog pin to A0 of Arduino as shown in image above.

Source Code/Program
The source code for Interfacing DFRobot Gravity Ph Sensor with Arduino is given below. Simply copy the code and upload it to the Arduino Board.
#define SensorPin A0 // the pH meter Analog output is connected with the Arduino’s Analog
unsigned long int avgValue; //Store the average value of the sensor feedback
float b;
int buf[10],temp;
void setup()
{
pinMode(13,OUTPUT);
Serial.begin(9600);
Serial.println("Ready"); //Test the serial monitor
}
void loop()
{
for(int i=0;i<10;i++) //Get 10 sample value from the sensor for smooth the value
{
buf[i]=analogRead(SensorPin);
delay(10);
}
for(int i=0;i<9;i++) //sort the analog from small to large
{
for(int j=i+1;j<10;j++)
{
if(buf[i]>buf[j])
{
temp=buf[i];
buf[i]=buf[j];
buf[j]=temp;
}
}
}
avgValue=0;
for(int i=2;i<8;i++) //take the average value of 6 center sample
avgValue+=buf[i];
float phValue=(float)avgValue*5.0/1024/6; //convert the analog into millivolt
phValue=3.5*phValue; //convert the millivolt into pH value
Serial.print(" pH:");
Serial.print(phValue,2);
Serial.println(" ");
digitalWrite(13, HIGH);
delay(800);
digitalWrite(13, LOW);
}
Aqueous Solution for Testing the Ph of different Solutions
For testing the Ph meter designed above, I used 4 different solutions with different Ph value. I took the following solutions
1. Soap Water: Ph value is around 9-10
2. Milk: Ph Value is around 6.5 to 6.7
3. Tap Water: Ph Value is around 6.5 to 8.5
4. Lemon Juice: Ph Value is in between 2 to 3

Testing & Results
Once the code is uploaded to Arduino Board, you can open serial monitor and start testing the Ph Sensor. I tested the Ph Sensor with All 4 solutions given above and found the following results




Interfacing Ph Sensor with Arduino & OLED Display
Now Let us display the Ph Value on OLED Display instead of Serial Monitor. To do that we need to add a 0.96″ I2C OLED Display to Arduino Uno. Hence the connection diagram is shown below.

The connection is fairly simple again. Connect the Analog pin of Ph Sensor to Arduino A0 pin and supply 5V to it. Also, connect the SDA SCL pin of OLED Display to Arduino A4 & A5 Pin respectively.
Source Code/Program
The source code for Interfacing DFRobot Gravity Ph Sensor with Arduino and OLED Display is given below. Simply copy the code and upload it to the Arduino Board.
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#define SensorPin 0 // the pH meter Analog output is connected with the Arduino’s Analog
unsigned long int avgValue; //Store the average value of the sensor feedback
float b;
int buf[10],temp;
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
#define OLED_RESET -1 // Reset pin # (or -1 if sharing reset pin)
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET);
void setup()
{
pinMode(13,OUTPUT);
Serial.begin(9600);
Serial.println("Ready"); //Test the serial monitor
if(!display.begin(SSD1306_SWITCHCAPVCC, 0x3C))
{
Serial.println(F("SSD1306 allocation failed"));
for(;;); // Don't proceed, loop forever
}
display.display();
delay(2);
display.clearDisplay();
display.clearDisplay();
display.setTextColor(WHITE);
display.setTextSize(2);
display.setCursor(0,5);
display.print("PH Sensor");
display.display();
delay(3000);
}
void loop()
{
for(int i=0;i<10;i++) //Get 10 sample value from the sensor for smooth the value
{
buf[i]=analogRead(SensorPin);
delay(10);
}
for(int i=0;i<9;i++) //sort the analog from small to large
{
for(int j=i+1;j<10;j++)
{
if(buf[i]>buf[j])
{
temp=buf[i];
buf[i]=buf[j];
buf[j]=temp;
}
}
}
avgValue=0;
for(int i=2;i<8;i++) //take the average value of 6 center sample
avgValue+=buf[i];
float phValue=(float)avgValue*5.0/1024/6; //convert the analog into millivolt
phValue=3.5*phValue; //convert the millivolt into pH value
Serial.print(" pH:");
Serial.print(phValue,2);
Serial.println(" ");
display.clearDisplay();
display.setTextSize(2);
display.setCursor(20,0);
display.println("Ph Value");
display.setTextSize(3);
display.setCursor(30,30);
display.print(phValue);
display.display();
digitalWrite(13, HIGH);
delay(800);
digitalWrite(13, LOW);
}
Testing & Results
Once the code is uploaded the OLED display will start showing the value of Ph. Dipping the Ph electrode on different solutions will give different value as shown in the images below.



Ph Sensor Calibration
If you worked with PH metering before you will know that PH values range from 0-14. Where PH 0 is very acidic, PH 7 will be neutral and PH 14 very alkaline. The biggest problem while using the Ph Sensor is about the calibration. Since the Ph sensor is an analog sensor so there is a need for calibration as the output is dependent upon the voltage. So we need a solution whose Ph Strength is known to us. There are various buffer solutions available in the market whose PH is fixed.

The simplest method to calibrate the Ph sensor is to dip the ph electrode on the solution of known strength and observe the reading. For example, when the electrode is dipped in a solution whose Ph Value is 7, the reading should show 7. In case it doesn’t show the correct reading, you need to rotate the potentiometer placed on Signal Conversion Board (Transmitter) V2. Once the reading matches, you can stop rotating and hence the sensor is calibrated.

Precautions & Things to Follow
-The BNC connector and the signal conversion board must be kept dry and clean, otherwise, it will affect the input impedance, resulting in an inaccurate measurement. If it is damp, it needs to be dried.
-The signal conversion board cannot be directly placed on a wet or semiconductor surface, otherwise, it will affect the input impedance, resulting in an inaccurate measurement. It is recommended to use the nylon pillar to fix the signal conversion board, allow a certain distance between the signal conversion board and the surface.
-The sensitive glass bubble in the head of the pH probe should avoid touching with the hard material. Any damage or scratches will cause the electrode to fail.
-After completing the measurement, disconnect the pH probe from the signal conversion board. The pH probe should not be connected to the signal conversion board without the power supply for a long time.
Video Tutorial & Explanation
If you want an IoT Based Ph Meter, you can follow the IoT pH Meter Tutorial and make it work with ESP32 & Thingspeak Server.
The article was first published in how2electronics, January 21, 2022
cr: https://how2electronics.com/ph-meter-using-ph-sensor-arduino-oled/
author: Admin
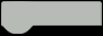