This project monitors temperature, humidity, and particulate matter concentrations in an industrial print room setting.

Things used in this project
Hardware components
Hand tools and fabrication machines
3D Printer (generic)
Soldering iron (generic)
Story
This project uses a 2 main sensors to monitor the environment within our print room. The particulate matter(PM2.5 HM3301) sensor, and the BME280 sensor which measure temperature and humidity. We added a blower fan right above the Micro-USB port to circulate air through the enclosure.
The HM-3301 Dust Sensor is based on the advanced Mie scattering theory. When light passes through particles with quantity same as or larger than wavelength of the light, it will produce light scattering. The scattered light is concentrated to a highly sensitive photodiode, which is then amplified and analyzed by a circuit. With specific mathematical model and algorithm, the count concentration and mass concentration of the dust particles is obtained.
This project is being recorded on a visual dashboard where charts show the information in an easy to read format.
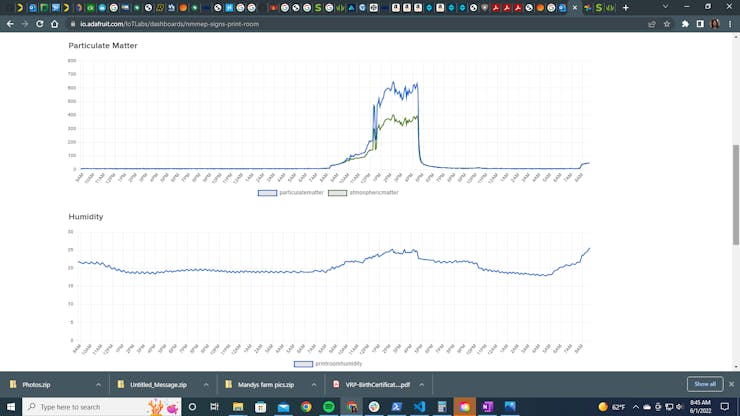
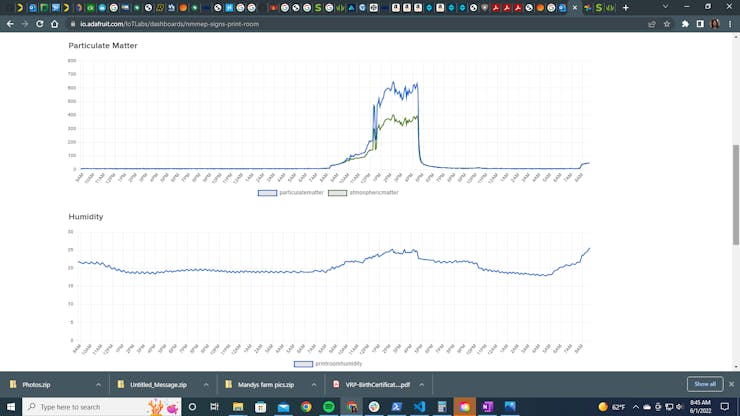
Custom parts and enclosures
Print Room Enclosure 1
Print Room Enclosure 2
Schematics
Print Room Monitor fritz

Print Room Monitor Schem

Code
Print Room Monitor
C/C++
#include <Adafruit_BME280.h>
#include <Adafruit_MQTT.h>
#include "Adafruit_MQTT/Adafruit_MQTT.h"
#include "Adafruit_MQTT/Adafruit_MQTT_SPARK.h"
#include "credentials.h"
#include <Wire.h>
TCPClient TheClient;
Adafruit_MQTT_SPARK mqtt(&TheClient,AIO_SERVER,AIO_SERVERPORT,AIO_USERNAME,AIO_KEY);
Adafruit_MQTT_Publish ParticulateMatter = Adafruit_MQTT_Publish(&mqtt, AIO_USERNAME "/feeds/ParticulateMatter");
Adafruit_MQTT_Publish PrintRoomTemperature = Adafruit_MQTT_Publish(&mqtt, AIO_USERNAME "/feeds/PrintRoomTemperature");
Adafruit_MQTT_Publish PrintRoomHumidity = Adafruit_MQTT_Publish(&mqtt, AIO_USERNAME "/feeds/PrintRoomHumidity");
Adafruit_MQTT_Publish AtmosphericMatter = Adafruit_MQTT_Publish(&mqtt, AIO_USERNAME "/feeds/AtmosphericMatter");
Adafruit_BME280 bme;
float tempC;
float tempF;
float humidRH;
bool status;
unsigned long lastTime, last;
int PM25;
uint8_t buf[30];
const char* str[] = {"sensor num: ", "PM1.0 concentration(CF=1,Standard particulate matter,unit:ug/m3): ",
"PM2.5 concentration(CF=1,Standard particulate matter,unit:ug/m3): ",
"PM10 concentration(CF=1,Standard particulate matter,unit:ug/m3): ",
"PM1.0 concentration(Atmospheric environment,unit:ug/m3): ",
"PM2.5 concentration(Atmospheric environment,unit:ug/m3): ",
"PM10 concentration(Atmospheric environment,unit:ug/m3): ",
};
void setup() {
Serial.begin(9600);
Wire.begin();
Wire.beginTransmission(0x40);
Wire.write(0x88);
Wire.endTransmission(false);
status = bme.begin(0x76);
if (status == false) {
Serial.printf("BME280 at address 0x%02X failed to start", 0x76);
}
}
void loop() {
MQTT_connect();
pingBroker();
if((millis()-lastTime) >60000){
read_sensor_value(buf,29);
parse_result_value(buf);
standardMatterRead(buf);
atmosphericMatterRead(buf);
readTempF();
readHumidity();
lastTime = millis();
}
}
void MQTT_connect() {
int8_t ret;
if (mqtt.connected()) {
return;
}
Serial.print("Connecting to MQTT... ");
while ((ret = mqtt.connect()) != 0) { // connect will return 0 for connected
Serial.printf("%s\n",(char *)mqtt.connectErrorString(ret));
Serial.printf("Retrying MQTT connection in 5 seconds..\n");
mqtt.disconnect();
delay(5000); // wait 5 seconds
}
Serial.printf("MQTT Connected!\n");
}
void pingBroker(){
if ((millis()-last)>120000) {
Serial.printf("Pinging MQTT \n");
if(! mqtt.ping()) {
Serial.printf("Disconnecting \n");
mqtt.disconnect();
}
last = millis();
}
}
void read_sensor_value(uint8_t* data, uint32_t data_len){
Wire.requestFrom(0x40, 29);
for (int i = 0; i < data_len; i++){
data[i] = Wire.read();
}
}
void parse_result_value(uint8_t* data){
for (int i = 0; i < 28; i++){
Serial.println(data[i], HEX);
}
uint8_t sum = 0;
for (int i = 0; i < 28; i++){
sum += data[i];
}
if (sum != data[28]){
Serial.printf("wrong checkSum!! \n");
}
}
void standardMatterRead(uint8_t* data){
uint16_t value = 0;
int i = 4;
value = (uint16_t) data[i * 2] << 8 | data[i * 2 + 1];
print_result(str[i - 1], value);
if(mqtt.Update()){
ParticulateMatter.publish(value);
}
}
void atmosphericMatterRead(uint8_t* data){
uint16_t value2 = 0;
int i = 7;
value2 = (uint16_t) data[i * 2] << 8 | data[i * 2 + 1];
print_result(str[i - 1], value2);
if(mqtt.Update()){
AtmosphericMatter.publish(value2);
}
}
void print_result(const char* str, uint16_t value){
Serial.printf(str);
Serial.println(value);
}
void readTempF() {
tempC = bme.readTemperature();
tempF = map(tempC, 0.0, 100.0, 32.0, 212.0);
Serial.printf("Temperature %.01f \n", tempF);
if(mqtt.Update()){
PrintRoomTemperature.publish(tempF);
}
}
void readHumidity() {
humidRH = bme.readHumidity();
Serial.printf("Humidity %0.1f \n", humidRH);
if(mqtt.Update()){
PrintRoomHumidity.publish(humidRH);
}
}
The article was first published in hackster, June 1, 2022
cr: https://www.hackster.io/user2152977/print-room-monitor-978e4c
author: user2152977, Brian Rashap
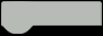