IoT-based patient health monitoring system using Arduino and generic ESP8266. The proposed project can collect and send patientās health data
Things used in this project
Ā
Hardware components
Story
Ā
IoT based patient health monitoring system is a generic term given to any medical equipment that has internet capability and can measure one or more health data of a patient who is connected to the device such as heartbeat, body temperature, blood pressure, ECG, steps etc. The equipment can record, transmit and alert if there is any abrupt change in the patientās health.
Ā
By this definition, it includes devices such as smart-watches, fitness trackers, smart-phones to expensive hospital equipment which can connect to internet.
Ā
IoT based health monitoring system is used where the patient and heath expert(s) are at different locations. For example, a patient can stay at home and continue his/her routine life and a doctor can monitor patientās heath. Based on the received data the heath expert can prescribe a best treatment or take an immediate action in case of an emergency.
Ā
Ā
Ā
Ā
Schematics
Ā
IoT-based health monitoring system | Arduino Project
Ā
Ā
Code
Code for Arduino
Arduino
#include <LiquidCrystal.h>
#include <SoftwareSerial.h>
#include <OneWire.h>
#include <DallasTemperature.h>
#define USE_ARDUINO_INTERRUPTS true
#include <PulseSensorPlayground.h>
SoftwareSerial esp(10, 11);
LiquidCrystal lcd(7, 6, 5, 4, 3, 2);
#define ONE_WIRE_BUS 9
#define TEMPERATURE_PRECISION 12
OneWire oneWire(ONE_WIRE_BUS);
DallasTemperature sensors(&oneWire);
DeviceAddress tempDeviceAddress;
int numberOfDevices, temp, buzzer = 8;
const int PulseWire = A0;
int myBPM, Threshold = 550;
PulseSensorPlayground pulseSensor;
unsigned long previousMillis = 0;
const long interval = 5000;
void setup()
{
lcd.begin(16, 2);
Serial.begin(9600);
esp.begin(115200);
sensors.begin();
numberOfDevices = sensors.getDeviceCount();
pulseSensor.analogInput(PulseWire);
pulseSensor.setThreshold(Threshold);
pulseSensor.begin();
pinMode(buzzer, OUTPUT);
digitalWrite(buzzer, HIGH);
lcd.setCursor(0, 0);
lcd.print(" IoT Patient");
lcd.setCursor(0, 1);
lcd.print(" Monitor System");
delay(1500);
digitalWrite(buzzer, LOW);
lcd.clear();
}
void loop()
{
myBPM = pulseSensor.getBeatsPerMinute();
if (pulseSensor.sawStartOfBeat())
{
beep();
lcd.setCursor(0, 1);
lcd.print("HEART:");
lcd.print(myBPM);
lcd.setCursor(9, 1);
lcd.print(" BPM");
delay(20);
}
sensors.requestTemperatures();
for (int i = 0; i < numberOfDevices; i++)
{
if (sensors.getAddress(tempDeviceAddress, i))
{
temp = printTemperature(tempDeviceAddress);
lcd.setCursor(0, 0);
lcd.print("BODY:");
lcd.print(temp);
lcd.print(" *C");
}
}
upload();
}
int printTemperature(DeviceAddress deviceAddress)
{
int tempC = sensors.getTempC(deviceAddress);
return tempC;
}
void beep()
{
digitalWrite(buzzer, HIGH);
delay(150);
digitalWrite(buzzer, LOW);
}
void upload()
{
unsigned long currentMillis = millis();
if (currentMillis - previousMillis >= interval)
{
previousMillis = currentMillis;
esp.print('*');
esp.print(myBPM);
esp.print(temp);
esp.println('#');
}
}
Code for Esp 8266
Arduino
#include "ThingSpeak.h"
#include <ESP8266WiFi.h>
//------- WI-FI details ----------//
char ssid[] = "XXXXXXXXXXX"; // SSID here
char pass[] = "YYYYYYYYYYY"; // Passowrd here
//--------------------------------//
//----------- Channel details ----------------//
unsigned long Channel_ID = 123456; // Channel ID
const char * myWriteAPIKey = "ABCDEFG1234"; //Your write API key
//-------------------------------------------//
const int Field_Number_1 = 1;
const int Field_Number_2 = 2;
String value = "";
int value_1 = 0, value_2 = 0;
int x, y;
WiFiClient client;
void setup()
{
Serial.begin(115200);
WiFi.mode(WIFI_STA);
ThingSpeak.begin(client);
internet();
}
void loop()
{
internet();
if (Serial.available() > 0)
{
delay(100);
while (Serial.available() > 0)
{
value = Serial.readString();
if (value[0] == '*')
{
if (value[5] == '#')
{
value_1 = ((value[1] - 0x30) * 10 + (value[2] - 0x30));
value_2 = ((value[3] - 0x30) * 10 + (value[4] - 0x30));
}
else if (value[6] == '#')
{
value_1 = ((value[1] - 0x30) * 100 + (value[2] - 0x30) * 10 + (value[3] - 0x30));
value_2 = ((value[4] - 0x30) * 10 + (value[5] - 0x30));
}
}
}
}
upload();
}
void internet()
{
if (WiFi.status() != WL_CONNECTED)
{
while (WiFi.status() != WL_CONNECTED)
{
WiFi.begin(ssid, pass);
delay(5000);
}
}
}
void upload()
{
ThingSpeak.writeField(Channel_ID, Field_Number_1, value_1, myWriteAPIKey);
delay(15000);
ThingSpeak.writeField(Channel_ID, Field_Number_2, value_2, myWriteAPIKey);
delay(15000);
value = "";
}
The article was first published in hackster, September 4, 2022
cr: https://www.hackster.io/rajeshjiet/iot-based-health-monitoring-system-arduino-project-69056f
author: rajeshjiet
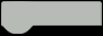