Extend the functionality of your current keyboard with custom capacitive touch keys.

Things used in this project
Hardware components
Story
Introduction
The Arduino Leonardo is a wonderful microcontroller. In addition to maintaining the same form factor as the venerable Uno, it also has the ability to serve as a USB keyboard. This means that you can program your Leonardo to press specific keys or print strings in response to various stimuli.
Pair it with a few capacitive touch pads and you can produce a device that essentially adds four fully-programmable keys to your workspace! Use each key to type commonly-used phrases, perform specific macros, press hard-to-use key combinations, and so much more! Let's get started.
Step 1: Assembly
This part is exceedingly simple. All you need to do is insert the included JST cables into the sockets and begin wiring. All the black wires should be connected to ground, the red wires to 5V, and the green wires to pins 2, 3, 4, and 5. The Leonardo only has a few power pins so it's helpful to connect the ground and VCC wires together using a breadboard. For simplicity's sake I recommend that you arrange the pads in numerical order, starting with the pad connected to pin 2 on the left and ending with pin 5 on the right.
I also used some double-sided tape to hold the pads in place. You could accomplish this with a whole number of different household products.
Step 2: Example Sketch
#include <Keyboard.h>
void setup(){
pinMode(2, INPUT);
pinMode(3, INPUT);
pinMode(4, INPUT);
pinMode(5, INPUT);
Keyboard.begin();
}
void loop(){
checkPress(2, 'd');
checkPress(3, 'f');
checkPress(4, 'g');
checkPress(5, 'h');
}
void checkPress(int pin, char key) {
if (digitalRead(pin)) {
Keyboard.press(key);
}
else {
Keyboard.release(key);
}
}
Try uploading the above software to your Leonardo. Click on an empty text box and try pressing the pads to ensure that each one outputs a character. Go to http://www.apronus.com/music/flashpiano.htm and you can use each of the keys to play chords!

Step 3: Creating Hotkeys
#include <Keyboard.h>
const int delayValue = 100;
const char cmdKey = KEY_LEFT_GUI;
void setup(){
pinMode(2, INPUT);
pinMode(3, INPUT);
pinMode(4, INPUT);
pinMode(5, INPUT);
Keyboard.begin();
}
void loop(){
if (digitalRead(2)) {
Keyboard.press(cmdKey);
Keyboard.press('c');
delay(delayValue);
Keyboard.releaseAll();
while(digitalRead(2));
}
if (digitalRead(3)) {
Keyboard.press(cmdKey);
Keyboard.press('v');
delay(delayValue);
Keyboard.releaseAll();
while(digitalRead(3));
}
if (digitalRead(4)) {
Keyboard.press(cmdKey);
Keyboard.press('n');
delay(delayValue);
Keyboard.releaseAll();
while(digitalRead(4));
}
if (digitalRead(5)) {
Keyboard.press(cmdKey);
Keyboard.press('z');
delay(delayValue);
Keyboard.releaseAll();
while(digitalRead(5));
}
}
The above sketch allows Mac users to use their pads for some common keyboard combinations. You can find the full list of all key definitions at https://www.arduino.cc/en/Reference/KeyboardModifiers.
Step 4: Going Further
Of course, simple key combinations and strings are not the only uses for such a system. Coupled with a program such as Keyboard Maestro, you could get your keys to perform extremely complex tasks. I set up some of my keys to lock my computer, turn sound on/off, and open specific programs! Consider it a fully-programmable computer interface that you can design for any purpose.
That's it! I'd love to hear what you used this project for, so post a comment below. If you want to see more of my work you can visit www.AlexWulff.com
Code
PrintChars
Arduino
#include <Keyboard.h>
void setup(){
pinMode(2, INPUT);
pinMode(3, INPUT);
pinMode(4, INPUT);
pinMode(5, INPUT);
Keyboard.begin();
}
void loop(){
checkPress(2, 'd');
checkPress(3, 'f');
checkPress(4, 'g');
checkPress(5, 'h');
}
void checkPress(int pin, char key) {
if (digitalRead(pin)) {
Keyboard.press(key);
}
else {
Keyboard.release(key);
}
}
Hotkeys
Arduino
#include <Keyboard.h>
const int delayValue = 100;
const char cmdKey = KEY_LEFT_GUI;
void setup(){
pinMode(2, INPUT);
pinMode(3, INPUT);
pinMode(4, INPUT);
pinMode(5, INPUT);
Keyboard.begin();
}
void loop(){
if (digitalRead(2)) {
Keyboard.press(cmdKey);
Keyboard.press('c');
delay(delayValue);
Keyboard.releaseAll();
while(digitalRead(2));
}
if (digitalRead(3)) {
Keyboard.press(cmdKey);
Keyboard.press('v');
delay(delayValue);
Keyboard.releaseAll();
while(digitalRead(3));
}
if (digitalRead(4)) {
Keyboard.press(cmdKey);
Keyboard.press('n');
delay(delayValue);
Keyboard.releaseAll();
while(digitalRead(4));
}
if (digitalRead(5)) {
Keyboard.press(cmdKey);
Keyboard.press('z');
delay(delayValue);
Keyboard.releaseAll();
while(digitalRead(5));
}
}
The article was first published in hackster, August 31, 2017
cr: https://www.hackster.io/AlexWulff/capacitive-touch-keyboard-extension-with-leonardo-13a387
author: Alex Wulff
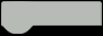