UNIHIKER is equipped with a variety of on-board hardware and expansion interfaces, making it ideal for controlling a wide range of sensors and actuators.
In this tutorial, we connect UNIHIKER to various Gravity modules and utilize Python programming for hardware control, showcasing the implementation of an intelligent car system
The project showcases the following functions:
1.Control Panel: Use the UNIHIKER Screen as an interface that allows users to monitor various car conditions.
2.Traffic Light Recognition: Employ an RGB color sensor to detect the color of a color wheel and regulate the motor's speed, simulating the traffic light recognition of a self-driving vehicle.
3.Light-Sensitive Headlights: Activate the headlights automatically when ambient light is insufficient, ensuring optimal visibility and energy efficiency.
4.Parking Assistant: Utilize an ultrasonic sensor installed on the rear of the car to detect obstacles. It alerts the driver and pedestrians by producing sound and lighting up LEDs.
5.Automatic Fan: Automatically activate the fan when the temperature rises, maintaining a comfortable interior climate.
UNIHIKER Overview:
UNIHIKER comes equipped with built-in sensors, such as a microphone, light sensor, accelerometer, gyroscope, and more, allowing for easy hardware learning without the need for additional modules. It also integrates a touch screen that enables projects with interactive displays. This feature-rich platform provides a convenient starting point for exploring and experimenting with various hardware capabilities.
UNIHIKER has multiple interfaces on its side. Furthermore, it shares the same edge connector as micro:bit, making it compatible with micro:bit expansion boards. In this project, UNIHIKER is connected to a driver expansion board to provide additional pins and connectors for modules and motors.
Hardware Connection:
Ultrasonic Sensor - P0
Temperature Sensor - P1
Fan - P8
RGB LED[2] - RGB LED[1] - RGB LED[0] - P12
*The RGB LED can be connected in cascade, enabling control of an RGB LED Strip with just one signal pin. In this setup, the 1st and 2nd RGB LEDs serve as the Headlight, while the 3rd one functions as the color recognition indicator. (Please note that the 5 LED module depicted in the picture is not available for purchase; instead, a single RGB LED can be used in its place)
LED - P14
LED - P15
Buzzer - P16
Color Recognition Sensor - IIC
Interface Design:
The control panel user interface mimics a real car's control panel. It displays essential information such as the current time, motor status, signal indicators, and the overall condition of the vehicle. Each element of the interface has been designed using image design software and saved in the PNG format. This means that you don't need to worry about complicated layout design through coding. Instead, the focus is on retrieving real-time data and displaying it in the corresponding areas of the interface.
Please find the image and code in the attached file for download.
import time, datetime
from unihiker import GUI # Import package
from pinpong.board import *
from pinpong.extension.unihiker import *
from pinpong.libs.dfrobot_tcs34725 import TCS34725
from pinpong.libs.dfrobot_dualuse_shield import DFRobot_DualuseShield
'''Hardware Initialization'''
Board().begin() # Initialize
adc0 = ADC(Pin(Pin.P0)) # Ultrasonic
adc1 = ADC(Pin(Pin.P1)) # Temperature
tcs = TCS34725() # Instantiate TCS34725 class, create tcs object (color sensor)
# Fan
fan = Pin(Pin.P8, Pin.OUT) # Initialize pin as output level
# LED Strip
np_12 = NeoPixel(Pin(Pin.P12), 3) # Pin 9, 1+2 lights
np_12.clear() # Clear display
# LED 1/LED 2
led1 = Pin(Pin.P14, Pin.OUT) # Initialize pin as output level
led2 = Pin(Pin.P15, Pin.OUT) # Initialize pin as output level
# Buzzer
tone = Tone(Pin(Pin.P16)) # Pass Pin to Tone for analog output
tone.freq(200) # Play at the set frequency
# Motor
p_mbt0014_motor = DFRobot_DualuseShield()
# Display different colors of light for LED strip and RGB light
def lamp_bright(): # Bright - White light
np_12[0] = (255, 255, 255) # Set RGB brightness for the first light, 1st white light
np_12[1] = (255, 255, 255) # Set RGB brightness for the second light, 2nd white light
def lamp_close(): # Off - Black
np_12[0] = (0, 0, 0) # Set RGB brightness for the first light, 1st white light
np_12[1] = (0, 0, 0) # Set RGB brightness for the second light, 2nd white light
def lamp_red(): # Red light on
np_12[2] = (255, 0, 0) # Set RGB brightness for the third light, red light
def lamp_yellow(): # Yellow light on
np_12[2] = (255, 255, 0)
def lamp_green(): # Green light on
np_12[2] = (0, 255, 0) # Set RGB brightness for the third light, green light
def lamp_off(): # Turn off lights
np_12[2] = (0, 0, 0) # Set RGB brightness for the third light, white light
# Initialize color sensor to confirm successful connection
while True:
if tcs.begin(): # Initialize color sensor, return True if successful
print("Found sensor") # Print "Found sensor"
break # Exit loop
else: # Otherwise
print("No TCS34725 found ... check your connections") # Print "No TCS34725 found ... check your connections"
time.sleep(1)
'''Screen Initialization'''
gui = GUI() # Instantiate GUI class
image_background = gui.draw_image(x=0, y=0, image='image/行空板车UI_画板.png') # Background
text_time = gui.draw_text(x=100, y=5, text='', color=(255, 255, 255), font_size=10) # Time 08:00
text_status = gui.draw_text(x=50, y=60, text='', color=(255, 255, 255), font_size=34) # Status (FAST, SLOW, STOP)
image_signal = gui.draw_image(x=300, y=160, image='image/行空板车UI-04.png') # Signal light (GREEN, YELLOW, RED) x=0
text_temp = gui.draw_text(x=25, y=222, text='', color=(255, 255, 255), font_size=13) # Temperature 30
text_temp_unit = gui.draw_text(x=65, y=222, text='', color=(255, 255, 255), font_size=13) # Temperature unit (℃)
text_fan = gui.draw_text(x=130, y=222, text='', color=(255, 255, 255), font_size=13) # ON/OFF
text_daylight = gui.draw_text(x=25, y=272, text='', color=(255, 255, 255), font_size=13) # 300
text_headlight = gui.draw_text(x=130, y=272, text='', color=(255, 255, 255), font_size=13) # ON/OFF
s = 0
for i in range(8):
v = adc1.read() # Get temperature analog value
v = round(v * 3.5 * 100 / 4096, 1) # Convert to temperature
print(v)
s = s + v
time.sleep(1)
print(s)
agv = round(s / 8, 1)
print("Average temperature is", agv)
text_temp.config(text=agv)
text_temp_unit.config(text='℃')
time.sleep(2)
# Ultrasonic thread
def start1():
while True:
data_dis = adc0.read() # Get distance
print("Distance", data_dis)
'''Ultrasonic control LED and buzzer'''
if data_dis < 50:
led1.write_digital(1) # Set high level output for LED1
led2.write_digital(1) # Set high level output for LED2
tone.on() # Turn off the buzzer
else:
led1.write_digital(0) # Set low level output for LED1
led2.write_digital(0) # Set low level output for LED2
tone.off() # Turn off the buzzer
time.sleep(0.5)
# Light thread
def start2():
while True:
data_light = light.read() # Get light intensity
print("Light", data_light)
text_daylight.config(text=data_light)
'''Light control lights'''
if data_light < 200:
lamp_bright()
text_headlight.config(text='ON')
else:
lamp_close()
text_headlight.config(text='OFF')
time.sleep(0.5)
# Temperature thread
def start3():
while True:
data_temp0 = adc1.read() # Get temperature analog value
data_temp1 = round(data_temp0 * 3.5 * 100 / 4096, 1) # Convert to temperature
print("Temperature", data_temp1)
text_temp.config(text=data_temp1)
text_temp_unit.config(text='℃')
'''Temperature control fan'''
if data_temp1 - agv > 3:
fan.write_digital(1) # Set high level output for fan
text_fan.config(text='ON')
else:
fan.write_digital(0) # Set low level output for fan
text_fan.config(text='OFF')
time.sleep(0.5)
# Color thread
def start4():
while True:
# Get color
r, g, b, c = tcs.get_rgbc() # Get rgbc data
# Data conversion
if c:
r /= c
g /= c
b /= c
r *= 256
g *= 256
b *= 256
print(c, "Red value:", r, "Green value:", g, "Blue value:", b) # Print color values
'''Color control motor'''
if r > 120: # Red light, stop
print("Red light, stop")
lamp_red()
p_mbt0014_motor.stop(p_mbt0014_motor.ALL)
text_status.config(text='STOP')
image_signal.config(image='image/行空板车UI-06.png', x=0)
elif g > 110 and r > 70: # Yellow light, slow down
print("Yellow light, slow down")
lamp_yellow()
p_mbt0014_motor.run(p_mbt0014_motor.ALL, p_mbt0014_motor.CW, 20)
text_status.config(text='SLOW')
image_signal.config(image='image/行空板车UI-05.png', x=0)
elif g > 110 and r < 70: # Green light, full speed
print("Green light, full speed")
lamp_green()
p_mbt0014_motor.run(p_mbt0014_motor.ALL, p_mbt0014_motor.CW, 200)
text_status.config(text='FAST')
image_signal.config(image='image/行空板车UI-04.png', x=0)
time.sleep(0.5)
# Start thread 1
thread1 = gui.start_thread(start1) # Ultrasonic
# Start thread 2
thread2 = gui.start_thread(start2) # Light
# Start thread 3
thread3 = gui.start_thread(start3) # Temperature
# Start thread 4
thread4 = gui.start_thread(start4) # Color
# Start the main program
while True:
now_time = datetime.datetime.now().strftime('%R')
print("------------------")
text_time.config(text=now_time)
time.sleep(0.5)
3D Structure:
For the traffic signal, we have designed a hexagon color wheel where the colors on the diagonal sides are identical. This means that the recognized color will be the same as the one displayed on the wheel.
Additionally, we have included 3D printed structures for the motors and fan, enhancing the overall functionality and design of the intelligent car system.

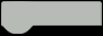