This project uses a PIR sensor to turn a fan ON/oFF and an LM35 sensor to control the speed of the fan with PWM.
Things used in this project
Hardware components
Hand tools and fabrication machines
Soldering iron (generic)
Solder Wire, Lead Free
Wire Stripper & Cutter, 18-10 AWG / 0.75-4mm² Capacity Wires
Scissor, Electrician
Hot glue gun (generic)
Story
We make this project because we want to create an efficient fan so that when we use the fan there is no need to manually set speed and on / off, or when we forget to turn off the fan we don't need to fear more electricity costs.
How it works on this fan includes:
1. In here the PIR sensor as an on / off device with input detects human or human movement.
2. LM35 sensor as a fan switch with indoor temperature input, when the room temperature is getting higher then the speed of the fan is also getting higher, and vice versa.
3. We also use the IRF530N transistor because the supply voltage is 12 Volts.
Custom parts and enclosures
Schematics
Automatic Fan with PIR Sensor and Arduino Sensor
Code
Code
Arduino
#include <avr/io.h>
//the time when the sensor outputs a low impulse
long unsigned int lowIn;
//the amount of milliseconds the sensor has to be low
//before we assume all motion has stopped
long unsigned int pause = 500;
boolean lockLow = true;
boolean takeLowTime;
const int pSuhu = A0; //inisialisasi pin to control LM35 pin A0
float suhu, outputlm, adc; //tipe data yang memungkinkan memuat angka desimal
int pirPin = 12; //digital pin connected to the PIR's output
int pirPos = 13; //connects to the PIR's 5V pin
int kipas = 3;
void setup(){
Serial.begin(9600); //begins serial communication
pinMode(pirPin, INPUT);
pinMode(pirPos, OUTPUT);
pinMode(kipas,OUTPUT);
digitalWrite(pirPos, HIGH);
pinMode(pSuhu, INPUT); // set pSuhu(LM35) to be INPUT
while (digitalRead(pirPin) == HIGH) {
delay(500);
Serial.print(".");
}
Serial.println("SENSOR ACTIVE");
}
void lm35(){
adc = analogRead(pSuhu); //save nilai from LM35 to variabel nilaiLM35
suhu = adc / 2.0479;
outputlm=adc*4.883;
if (suhu >= 25)
{
analogWrite(kipas,50);
Serial.println("Kecepatan=50");
}
if (suhu >= 30)
{
analogWrite(kipas,100);
Serial.println("Kecepatan=100");
}
if (suhu >= 35)
{
analogWrite(kipas,150);
Serial.println("Kecepatan=150");
}
if (suhu >= 40)
{
analogWrite(kipas,200);
Serial.println("Kecepatan=200");
}
if (suhu >= 45)
{
analogWrite(kipas,255);
Serial.println("Kecepatan=255");
}
}
void loop(){
if(digitalRead(pirPin) == HIGH){ //if the PIR output is HIGH, turn servo
lm35();
if(lockLow){
//makes sure we wait for a transition to LOW before further output is made
lockLow = false;
Serial.println("---");
Serial.print("motion detected at ");
Serial.print(millis()/1000);
Serial.println(" sec");
delay(50);
}
takeLowTime = true;
}
if(digitalRead(pirPin) == LOW){
digitalWrite(kipas,LOW);
if(takeLowTime){
lowIn = millis(); //save the time of the transition from HIGH to LOW
takeLowTime = false; //make sure this is only done at the start of a LOW phase
}
//if the sensor is low for more than the given pause,
//we can assume the motion has stopped
if(!lockLow && millis() - lowIn > pause){
//makes sure this block of code is only executed again after
//a new motion sequence has been detected
lockLow = true;
Serial.print("motion ended at "); //output
Serial.print((millis() - pause)/1000);
Serial.println(" sec");
delay(50);
}
}
delay(1000);
Serial.print(", Suhu: ");
Serial.print(suhu);
Serial.println(); //send data ASCII + CR,LF (kode enter)
delay(1000);
Serial.print(", ADC: ");
Serial.print(adc);
Serial.println(); //send data ASCII + CR,LF (kode enter)
delay(1000);
}
The article was first published in hackster, May 15, 2019
cr: https://www.hackster.io/Jamaluddin/automatic-fan-with-pir-sensor-and-lm35-sensor-de596e
author: Jamaluddin
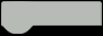