Introduction
This project aims to implement edge detection on UNIHIKER using OpenCV. This project interfaces a USB camera with UNIHIKER to detect objects and outline their edges.
Project Objectives
Learn how to implement Canny edge detection using the OpenCV library.
Software
Practical Process
1. Hardware Setup
Connect the camera to the USB port of UNIHIKER.

Connect the UNIHIKER board to the computer via USB cable.

2. Software Development
Step 1: Open Mind+, and remotely connect to UNIHIKER.

Step 2: Find a folder named "AI" in the "Files in UNIHIKER". And create a folder named "Edge Detection Using OpenCV on UNIHIKER" in this folder. Import the dependency files for this lesson.

Step3: Create a new project file in the same directory as the above file and name it "main.py".
Sample Program:
#!/usr/bin/env python
'''
This sample demonstrates Canny edge detection.
..
'''
# Python 2/3 compatibility
from __future__ import print_function
import cv2 as cv
import numpy as np
# relative module
import video
# built-in module
import sys
def main():
try:
fn = sys.argv[1]
except:
fn = 0
def nothing(*arg):
pass
cv.namedWindow('edge',cv.WND_PROP_FULLSCREEN) #Set the windows to be full screen.
cv.setWindowProperty('edge', cv.WND_PROP_FULLSCREEN, cv.WINDOW_FULLSCREEN) #Set the windows to be full screen.
cv.createTrackbar('thrs1', 'edge', 2000, 5000, nothing)
cv.createTrackbar('thrs2', 'edge', 4000, 5000, nothing)
cap = video.create_capture(fn)
while True:
_flag, img = cap.read()
gray = cv.cvtColor(img, cv.COLOR_BGR2GRAY)
thrs1 = cv.getTrackbarPos('thrs1', 'edge')
thrs2 = cv.getTrackbarPos('thrs2', 'edge')
edge = cv.Canny(gray, thrs1, thrs2, apertureSize=5)
vis = img.copy()
vis = np.uint8(vis/2.)
vis[edge != 0] = (0, 255, 0)
cv.imshow('edge', vis)
ch = cv.waitKey(5)
if ch == 27:
break
print('Done')
if __name__ == '__main__':
print(__doc__)
main()
cv.destroyAllWindows()
3. Run and Debug
Step 1: Run the Main Program
Run the "main.py" program. And then put Mind+ into the screen. The edges of the Mind+ will be promptly detected and outlined with green dots. And the edges of the table corners will also be detected.

4. Program Analysis
In the "main.py" file, we primarily use the OpenCV library to access the camera, capture real-time video streams, apply the Canny edge detection algorithm to identify edges, and finally display the edges as green dots in the window. Users can adjust the threshold of the Canny algorithm in real-time using sliders. The overall process is outlined as follows:
① Initialization: Upon program startup, command-line arguments are read to determine the video source. If no command-line argument is provided, the default camera is used. Then, a full-screen window named "edge" is created, along with two sliders within this window for adjusting the thresholds of the Canny algorithm.
② Main Loop: The program enters an infinite loop where, in each iteration, it reads a frame from the video source and then performs the following processing steps:
A: Convert the captured frame into grayscale.
B: Retrieve the current positions of the sliders, which serve as the thresholds for the Canny algorithm.
C: Apply the Canny algorithm to detect edges in the grayscale image, resulting in an edge image.
D: Create an image for display and set the pixels corresponding to edges in the edge image to green.
E: Display this image within the window.
③ User Interaction: The program will check keyboard input from the user. If you press 'ESC' , the main loop exits.
④ Termination: Upon exiting the main loop, the program destroys all windows and terminates.
The main objective of this program is to detect edges in real-time from the video stream and display them in a window. Users can dynamically adjust the edge detection threshold using sliders to observe the effects of edge detection under different thresholds.
Knowledge Corner - the Canny Algorithm
The Canny algorithm is a widely used edge detection technique introduced by John F. Canny in 1986. It is a multi-stage algorithm that involves the following steps:
1. Remove the Noise: Since edge detection is sensitive to noise, the first step involves smoothing the image using a Gaussian filter to remove noise.
2. Calculate Gradient Intensity and Direction: Next, the algorithm calculates the gradient of the image in both the horizontal and vertical directions using Sobel operators, thus obtaining the gradient intensity and direction for each pixel.
3. Non-Maximum Suppression(NMS): In this step, each pixel in the gradient magnitude image is compared with its neighbors along the gradient direction. If the gradient magnitude of the current pixel is not greater than either of its neighbors, its magnitude is set to zero. This step helps suppress pixels that are not part of edges.
4. Apply Double Thresholds: Two thresholds are determined: a high threshold and a low threshold, to further suppress weak edges. Pixels with intensity higher than the high threshold are considered "strong edges," those with intensity lower than the low threshold are immediately suppressed, and those with intensity between the two thresholds are labeled as "weak edges."
5. Track Edge by Hysteresis: The final step involves determining which edges are true edges. All strong edges are retained, and weak edges are kept if they are connected to strong edges.
The main advantage of the Canny algorithm is its excellent detection performance, accurately identifying edges in images, often with just one-pixel width. However, the algorithm's computational complexity may pose challenges for real-time applications.
Feel free to join our UNIHIKER Discord community! You can engage in more discussions and share your insights!
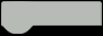