Introduction
This project aims to implement QR Code detection on UNIHIKER using OpenCV. This project interfaces a USB camera with UNIHIKER to detect QR codes in real-time using the camera and display the decoded results on screen.
Project Objectives
Learn how to decode QR Codes using OpenCV and the pyzbar Library
Software
Practical Process
1. Hardware Setup
Connect the camera to the USB port of UNIHIKER.

Connect the UNIHIKER board to the computer via USB cable.

2. Software Development
Step 1: Open Mind+, and remotely connect to UNIHIKER.

Step 2: Create a new file named "main.py" in "Files in Project".

Step3: Write Program
Double-click to open the "main.py" file.
Sample Program:
import cv2
import time
import numpy as np
from pyzbar.pyzbar import decode
def main():
cap = cv2.VideoCapture(0)
while not cap.isOpened():
continue
cap_w, cap_h = 240, 320
cv2.namedWindow("qrwindows", cv2.WND_PROP_FULLSCREEN)
cv2.setWindowProperty("qrwindows",cv2.WND_PROP_FULLSCREEN, cv2.WINDOW_FULLSCREEN)
while True:
qrimg_success, qrimg_src = cap.read()
xmin, ymin, w, h = 250, 100, cap_w, cap_h
qrimg_src = qrimg_src[ymin:ymin+h, xmin:xmin+w]
qrcode_val = decode(qrimg_src)
if len(qrcode_val) > 0:
for i in range(len(qrcode_val)):
qrcode_str = qrcode_val[i][0].decode()
print(qrcode_str)
point_x = qrcode_val[i][2][0]
point_y = qrcode_val[i][2][1]
point_w = qrcode_val[i][2][2]
point_h = qrcode_val[i][2][3]
point_1_x = qrcode_val[i][3][0][0]
point_1_y = qrcode_val[i][3][0][1]
point_2_x = qrcode_val[i][3][1][0]
point_2_y = qrcode_val[i][3][1][1]
point_3_x = qrcode_val[i][3][2][0]
point_3_y = qrcode_val[i][3][2][1]
point_4_x = qrcode_val[i][3][3][0]
point_4_y = qrcode_val[i][3][3][1]
cv2.rectangle(qrimg_src, (point_x, point_y), (point_x+point_w, point_y+point_h), (255, 0, 255), 2)
cv2.line(qrimg_src, (point_1_x, point_1_y), (point_2_x, point_2_y), (255, 0, 0), 2, cv2.FILLED)
cv2.line(qrimg_src, (point_2_x, point_2_y), (point_3_x, point_3_y), (255, 0, 0), 2, cv2.FILLED)
cv2.line(qrimg_src, (point_3_x, point_3_y), (point_4_x, point_4_y), (255, 0, 0), 2, cv2.FILLED)
cv2.line(qrimg_src, (point_4_x, point_4_y), (point_1_x, point_1_y), (255, 0, 0), 2, cv2.FILLED)
cv2.circle(qrimg_src, (point_1_x, point_1_y), 5, (255, 0, 0), 2)
cv2.circle(qrimg_src, (point_2_x, point_2_y), 5, (0, 255, 0), 2)
cv2.circle(qrimg_src, (point_3_x, point_3_y), 5, (0, 0, 255), 2)
cv2.circle(qrimg_src, (point_4_x, point_4_y), 5, (255, 255, 0), 2)
cv2.putText(qrimg_src,qrcode_str,(point_x,point_y-15), cv2.FONT_HERSHEY_COMPLEX, 0.4, (0, 0, 255), 1)
cv2.imshow('qrwindows', qrimg_src)
cv2.waitKey(10)
time.sleep(1)
else:
cv2.imshow('qrwindows', qrimg_src)
cv2.waitKey(10)
if __name__ == '__main__':
main()
3. Run and Debug
Run the Main Program, move the QR code into the camera's view. The screen of the UNIHIKER will display a QR code detection frame and its decoded content.

4. Program Analysis
In the above "main.py" file, we use the OpenCV library to access the camera and obtain real-time video streams. We then use the decode method from the pyzbar library to detect and parse strings represented by QR codes. Subsequently, we retrieve the coordinates of recognized QR codes, draw recognition boxes, and display the recognized content in real-time. The overall flow is as follows:
1. Camera Initialization:
- Initialize the camera using cv2.VideoCapture(0), where the parameter 0 denotes the default camera device.
- Wait for the camera to start: Use cap.isOpened() to verify if the camera has successfully opened. If not, continue waiting.
2. Create Display Window:
- Create a window named qrwindows using cv2.namedWindow and set it to full-screen display mode.
3. Main Loop:
- Enter an infinite loop to continuously capture images from the camera and process them for QR code detection.
- Read image: Use cap.read() to capture a frame from the camera.
- Cropping: Crop a region from the captured image based on predefined coordinates and size (adjusted for screen dimensions) for QR code detection.
- Decode QR code: Apply the decode function to decode QR codes within the cropped image area.
- Process detection results:
- If a QR code is detected, iterate through all detected QR codes.
- For each QR code, parse its content and print it to the console.
- Calculate the coordinates of QR code positioning points and draw recognition boxes, connecting lines, and positioning points on the image.
- Display the decoded QR code text above the recognition box and pause for viewing.
- If no QR code is detected, display the camera image and continue with the next cycle.
Knowledge Corner - QR code and QR code scanning & processing
QR codes are a fast and convenient way to transmit information, widely used in our daily lives. For example, people can make payments to merchants without cash by scanning QR codes; consumers can scan QR codes on products to obtain detailed information and trace the origin of goods. In practice, the various uses of QR codes rely on encoding and decoding information. QR codes store encoded information, which is captured by device cameras such as smartphones when scanned. The device then decodes the QR code, ultimately restoring the encoded information to its original form.

A QR code consists of a matrix of black and white squares, which are recognized and decoded by scanning devices. When identifying a QR code, the process includes image acquisition and preprocessing, positioning, segmentation, decoding, and parsing, as illustrated below.

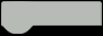