A few simple Arduino examples of using this low-cost compact esp32 + tft touch display module.
These days I received a shipment from Elecrow that contains several components that I ordered a week ago. My shipment arrived unexpectedly quickly, and is well packed and protected in a branded box. It consists of Crow Panel 2.8" HMI 320x240 display SPI TFT LCD Touch screen compatible with Arduino, LVGL Library, and ESP microcontroller onboard with all necessary cables and touch pen.

These are laser machined housing parts for this ESP32 Display, then a waterproof temperature sensor and a BME 280 sensor board. The sensors contain appropriate cables that are compatible with the Display board, so that when making a project there is no need for soldering, and there is also no possibility of wrong connection. When the board is turned on for the first time, a demo application appears on the display to demonstrate some of the capabilities of this small device. After assembling the case, the display looks tidy and is protected from damage.

On Elecrow's site you can find a lot of detailed information and tutorials about this display module using the LVGL library and Square Line Studio software, which drastically simplify the creation of the graphical environment without writing code. This time, my idea was to explain to you how to use this module to create simple code yourself using the TFT-Espi library, which is very often represented in DIY projects.
I will only use Arduino IDE to write code and upload it to the board. I will also explain how to control external components, specifically an LED as the simplest example.
First we need to enable display module in the Arduino environment. For this purpose we go to Arduino IDE - File - Preferences - where we add the ESP32 URL to "Board Manager URLs" as follows:
(https://raw.githubusercontent.com/espressif/arduino-esp32/gh-pages/package_esp32_index.json)

Now click "Tool-->Board-->Board Manager", and search for "esp32". It is recommended to
install version 2.0.3 ESP32 package.

Next we go to Sketch-> Include Library-> Manage Libraries -> TFT_eSPI -> Install


After that "User_Setup.h" in "" library folder needs to be modified according to their screen drive. For this purpose, we copy the "User-Setup.h" file given below, to the "TFT_eSPI" library folder, replacing the original file.

Now, on Arduino IDE -> Tools -> Boards manager -> ESP32 Arduino we choose: ESP32-WROOM-DA Module, and set the parameters as given in the image

With this, the procedure for entering support for the specific Display Мodule in the Arduino IDE is completed.
Since TFT_eSPI library is customized for this display module, we can now successfully upload code from some previous projects that use it. Of course, a huge advantage is that there is no need for any soldering, especially if we use Elecrow sensors and modules, and the display together with the microcontroller is small, compact, and housed in a nice case. First I will test the device with test examples that are provided in the library itself. For this purpose I go to Examples - TFT_eSPI - and select an example. Then select the appropriate port and upload the code.

and now, another example

As I mentioned above in the text, next I will describe to you a simple example of how to control an LED. For this purpose, on the two input-output pins (IO25 and IO32) of the display I will connect two colors from the RGB LED module through 220 Ohm current limiting resistors. To simplify the code, we need to install the TFT_eWidget library which contains ready-made buttons, gauges or graphs, and also tft_setup library.
Here is what the finished project looks like with the uploaded code, which you can download from the given link. Pressing the buttons turns the LEDs of the corresponding color on and off.

This is an example where we use ports 25 and 32 as outputs and with them we can control certain processes.
Next I will present you another simple example where one of these ports (25) is used as an input, and on it we can read the information generated by the temperature sensor. The display shows the current temperature in degrees Celsius.

You can also download the code and schematic for this project at the link given at the end of the project.
And finally, a short conclusion.
This time I tried to explain to you first how to install this nice display module in Arduino IDE, and then to make some simple projects using TFT-eSPI library which is very often used for various projects with graphic environment. In some of my next videos I will show you how to install some more complex useful code on this compact module.

#include "TFT_eSPI.h"
#include "TFT_eWidget.h"
TFT_eSPI tft = TFT_eSPI();
uint16_t cal[5] = { 286, 3478, 196, 3536, 2 };
const int led1 = 25;
const int led2 = 32;
ButtonWidget btn1 = ButtonWidget(&tft);
ButtonWidget btn2 = ButtonWidget(&tft);
ButtonWidget* btns[] = { &btn1, &btn2 };
void btn1_pressed(void) {
if (btn1.justPressed()) {
bool state = !btn1.getState();
btn1.drawSmoothButton(state, 2, TFT_WHITE, state ? "ON" : "OFF");
digitalWrite(led1, state ? HIGH : LOW);
}
}
void btn2_pressed(void) {
if (btn2.justPressed()) {
bool state = !btn2.getState();
btn2.drawSmoothButton(state, 2, TFT_WHITE, state ? "ON" : "OFF");
digitalWrite(led2, state ? HIGH : LOW);
}
}
void initButtons() {
uint16_t w = 100;
uint16_t h = 50;
uint16_t x = (tft.width() - w) / 2;
uint16_t y = tft.height() / 2 - h - 10;
btn1.initButtonUL(x, y, w, h, TFT_WHITE, TFT_BLACK, TFT_RED, "LED1", 2);
btn1.setPressAction(btn1_pressed);
btn1.drawSmoothButton(false, 2, TFT_BLACK);
y = tft.height() / 2 + 10;
btn2.initButtonUL(x, y, w, h, TFT_WHITE, TFT_BLACK, TFT_GREEN, "LED2", 2);
btn2.setPressAction(btn2_pressed);
btn2.drawSmoothButton(false, 2, TFT_BLACK);
}
void handleButtons() {
uint8_t nBtns = sizeof(btns) / sizeof(btns[0]);
uint16_t x = 0, y = 0;
bool touched = tft.getTouch(&x, &y);
for (uint8_t b = 0; b < nBtns; b++) {
if (touched) {
if (btns[b]->contains(x, y)) {
btns[b]->press(true);
btns[b]->pressAction();
}
} else {
btns[b]->press(false);
btns[b]->releaseAction();
}
}
}
void setup() {
pinMode(led1, OUTPUT);
pinMode(led2, OUTPUT);
tft.begin();
tft.setRotation(0);
tft.fillScreen(TFT_BLACK);
tft.setTouch(cal);
initButtons();
}
void loop() {
handleButtons();
delay(50);
}
#include <OneWire.h>
#include <DallasTemperature.h>
#include <TFT_eSPI.h> // Graphics and font library for ST7735 driver chip
#include <SPI.h>
//#include <WiFi.h>
#define TFT_BLACK 0x0000 // black
#define ONE_WIRE_BUS 25
OneWire oneWire(ONE_WIRE_BUS);
DallasTemperature sensors(&oneWire);
TFT_eSPI tft = TFT_eSPI(); // Invoke library, pins defined in User_Setup.h
void setup(void)
{
tft.init();
tft.setRotation(3);
Serial.begin(9600);
sensors.begin();
}
void loop(void)
{
Serial.print(" Requesting temperatures...");
sensors.requestTemperatures(); // Send the command to get temperature readings
Serial.println("DONE");
delay (1000);
tft.fillScreen(TFT_YELLOW);
tft.setCursor(20, 0, 2);
tft.setTextColor(TFT_BLUE,TFT_YELLOW);
tft.setTextSize(3);
tft.println("Temperature is: ");
tft.setCursor(60,180, 2);
tft.setTextColor(TFT_BLUE,TFT_YELLOW);
tft.setTextSize(3);
tft.println("Degrees C ");
tft.setCursor(40, 75, 4);
tft.setTextColor(TFT_BLUE,TFT_YELLOW);
tft.setTextSize(4);
tft.println(sensors.getTempCByIndex(0));
delay(2000);
}
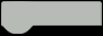