Creating a cooling controller using Arduino is a simple and efficient project that leverages temperature sensors, control mechanisms, and display modules to regulate temperature. The system consists of several components, including a temperature sensor (LM35, DHT22, or DS18B20), a cooling device such as a 12V fan or thermoelectric cooler (TEC), and an Arduino Nano as the central controller. Additional components include a relay module to control the cooling device, a 16x2 LCD display with I2C for user feedback, a potentiometer for setting the desired temperature, and an optional SD card module for data logging. The controller can also be enhanced with IoT capabilities for remote monitoring. Below is a detailed implementation guide with code.
Components and Working Principle
The temperature sensor measures the real-time temperature, which is compared to the user-defined setpoint. When the temperature exceeds this setpoint, the cooling device is activated via a relay module. The LCD display shows the current temperature, setpoint, and the status of the cooling system. A button provides manual override functionality, and a potentiometer allows users to adjust the temperature setpoint.
Code
#include
#include
// Pin Definitions
const int tempSensorPin = A0; // LM35 connected to Analog pin A0
const int relayPin = 8; // Relay module connected to Digital pin 8
const int setPointPin = A1; // Potentiometer for setting temperature setpoint
const int buttonPin = 2; // Button for manual override
const int decimalPrecision = 1; // Decimal places for display
// Variables
float temperature = 0; // Current temperature
float setPoint = 25.0; // Desired temperature
bool coolingActive = false; // Cooling status
bool manualOverride = false; // Manual control toggle
// Initialize LCD
LiquidCrystal_I2C lcd(0x27, 16, 2);
void setup() {
pinMode(relayPin, OUTPUT);
pinMode(buttonPin, INPUT_PULLUP);
lcd.begin();
lcd.backlight();
// Display initialization message
lcd.print("Cooling Ctrl Init");
delay(2000);
lcd.clear();
// Ensure relay is off at start
digitalWrite(relayPin, LOW);
}
void loop() {
// Read temperature
temperature = readTemperature();
// Read setpoint from potentiometer
setPoint = readSetPoint();
// Check button for manual override
if (digitalRead(buttonPin) == LOW) {
manualOverride = !manualOverride;
delay(500); // Debounce delay
}
// Control cooling device
if (!manualOverride) {
coolingActive = temperature > setPoint;
}
digitalWrite(relayPin, coolingActive ? HIGH : LOW);
// Display data
lcd.setCursor(0, 0);
lcd.print("Temp: ");
lcd.print(temperature, decimalPrecision);
lcd.print("C");
lcd.setCursor(0, 1);
lcd.print("SetPt: ");
lcd.print(setPoint, decimalPrecision);
lcd.print("C");
if (manualOverride) {
lcd.print(" M");
}
delay(1000); // Update every second
}
// Function to read temperature from LM35
float readTemperature() {
int sensorValue = analogRead(tempSensorPin);
float voltage = sensorValue * (5.0 / 1023.0);
return voltage * 100.0; // Convert voltage to Celsius for LM35
}
// Function to read setpoint from potentiometer
float readSetPoint() {
int potValue = analogRead(setPointPin);
return map(potValue, 0, 1023, 15, 30); // Map potentiometer value to 15-30°C range
}
Explanation
The setup() function initializes the LCD display and ensures the relay (connected to the cooling device) is off initially.
The readTemperature() function uses the LM35 sensor's analog output to calculate the ambient temperature in Celsius.
The readSetPoint() function maps the potentiometer's analog input to a temperature range of 15â30°C, allowing dynamic adjustment of the desired temperature.
In the loop() function, the current temperature is compared with the setpoint. If the temperature exceeds the setpoint and manual override is not active, the relay is triggered to turn on the cooling device.
A button allows users to manually toggle the cooling device on or off, overriding automatic control.
The LCD displays the current temperature, setpoint, and the cooling device's status, with an "M" indicator for manual mode.
Additional Features
Data Logging: Add an SD card module to log temperature and setpoint data over time.
IoT Integration: Incorporate a Wi-Fi module like ESP8266 to enable remote monitoring and control.
Advanced Cooling: Replace the relay with a PWM control circuit for more precise cooling.
This cooling controller is versatile and can be used in refrigeration systems, greenhouses, or any application requiring temperature regulation.
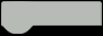