Greetings everyone, and welcome to my article tutorial. Today, I'll guide you through the process of creating an Otto Robot using Raspberry Pi Pico.
Project Overview:
This project is about Otto Robot using Raspberry Pi Pico. The Otto Robot, built using a Raspberry Pi Pico , is a compact and interactive humanoid robot designed for fun and learning. Equipped with servomotors, a PCA9685 PWM driver, and an ultrasonic sensor, it can perform actions like greeting, walking, dancing, and avoiding obstacles. Its stable power supply is ensured by a 9V battery regulated through an LM7805 IC.
This robot showcases the capabilities of the Raspberry Pi Pico, controlling multiple peripherals to create smooth, human-like movements. Its obstacle avoidance feature allows it to navigate autonomously, making it both engaging and functional. Perfect for beginners and hobbyists, this project is an exciting way to explore robotics and programming.
Now, let's get started with our project!
Electronic Components Required:

Either 3D print the provided CAD design or purchase a pre-made version (available on Amazon).








For this step, you'll need your 3D printed Otto Robot and 4 micro servo motors. Let's put them together:
Feet Assembly:
1) Insert each micro servo into the feet slots. If they don't fit smoothly, carefully clean the area with a utility knife
2) IMPORTANT: Test that each servo can rotate a full 90 degrees in both directions
3) Once movement is verified, secure each servo using a small screw
Body Assembly:
1) Place 2 micro servos into their designated spots in the robot's body
2) Secure them with screws
3) Attach the legs to the servo hubs
4) Test leg rotation - ensure each can move 90 degrees in both directions from the body
5) Once aligned, secure the legs using the small screws in the leg holes
Cable Management:
1) Guide the servo cables through the body slots and leg holes
Pro Tip: Take extra care when routing the cables - proper cable management will ensure smooth movement and prevent wire damage during operation.

Using the connection diagram above, connect each servo motor to its designated channel on the PCA9685 16 Channel Servo Motor Driver, follow that attached table image.




Before connecting the Raspberry Pi Pico to the circuit, ensure the code is uploaded to it. We will use the Arduino IDE to program the Raspberry Pi Pico, so make sure the Arduino IDE is installed on your device. Follow these steps:
1) Connect the Raspberry Pi Pico to your device using a USB cable.
2) Open the Arduino IDE, go to the "File" menu, and click on "Preferences."
3) In the "Additional Board Manager URLs" field, paste the following URL: https://github.com/earlephilhower/arduino-pico/releases/download/global/package_rp2040_index.json
4) Click "OK" to save the changes.
5) Navigate to the "Board Manager," search for "Pico," and install the relevant board as shown in the attached image.
6) Once the board is installed successfully, select the appropriate board and port from the Arduino IDE.
Then upload this code:
Note: For individual mode codes (walking, dancing, etc.), visit my GitHub repository: https://github.com/ShahbazCoder1/Otto-Robot-using-Raspberry-Pi-Pico-/tree/main
// Otto Robot Using Raspberry Pi Pico
// Code Written by: Md Shahbaz Hahsmi Ansari
// Published on Roboattic Lab
#include <Wire.h>
#include <Adafruit_PWMServoDriver.h>
Adafruit_PWMServoDriver pwm = Adafruit_PWMServoDriver();
#define SERVO_FREQ 50
#define SERVOMIN 150
#define SERVOMAX 600
// Define servo channels on PCA9685
#define LeftLeg 0
#define RightLeg 1
#define LeftFoot 2
#define RightFoot 3
// Calibration values
int YL = 0; // Left Leg trim
int YR = 0; // Right Leg trim
int RL = 0; // Left Foot trim
int RR = 0; // Right Foot trim
void setup() {
Serial.begin(9600);
// Initialize PCA9685
pwm.begin();
pwm.setPWMFreq(SERVO_FREQ);
}
int angleToPulse(int angle) {
angle = constrain(angle, 0, 180);
return map(angle, 0, 180, SERVOMIN, SERVOMAX);
}
void setServo(uint8_t servoNum, int angle) {
pwm.setPWM(servoNum, 0, angleToPulse(angle));
}
void homePosition() {
setServo(LeftLeg, 90);
setServo(RightLeg, 90);
setServo(LeftFoot, 90);
setServo(RightFoot, 80);
}
void hello() {
setServo(LeftFoot, 60);
for (int i = 0; i < 3; i++) {
setServo(RightLeg, 60);
delay(300);
setServo(RightLeg, 90);
delay(300);
setServo(RightFoot, 60);
delay(300);
setServo(RightFoot, 90);
delay(300);
}
homePosition();
}
void walk() {
// Basic walking sequence
// Step 1: Lift left foot
setServo(LeftFoot, 70);
delay(200);
// Step 2: Move left leg forward
setServo(LeftLeg, 120);
delay(200);
// Step 3: Lower left foot
setServo(LeftFoot, 90);
delay(200);
// Step 4: Lift right foot
setServo(RightFoot, 70);
delay(200);
// Step 5: Move right leg forward
setServo(RightLeg, 120);
delay(200);
// Step 6: Lower right foot
setServo(RightFoot, 90);
delay(200);
homePosition();
}
void dance() {
// Dance move 1: Side-to-side sway
for (int i = 0; i < 3; i++) {
setServo(LeftLeg, 80);
setServo(RightLeg, 100);
delay(300);
setServo(LeftLeg, 100);
setServo(RightLeg, 80);
delay(300);
homePosition();
}
// Foot tapping
for (int i = 0; i < 3; i++) {
setServo(LeftFoot, 60);
delay(200);
setServo(LeftFoot, 90);
delay(200);
setServo(RightFoot, 60);
delay(200);
setServo(RightFoot, 90);
delay(200);
homePosition();
}
// Twisting legs
for (int i = 0; i < 3; i++) {
setServo(LeftLeg, 70);
setServo(RightLeg, 110);
delay(300);
setServo(LeftLeg, 110);
setServo(RightLeg, 70);
delay(300);
homePosition();
}
// Wave legs up and down
for (int i = 0; i < 3; i++) {
setServo(LeftLeg, 60);
setServo(RightLeg, 120);
delay(300);
setServo(LeftFoot, 60);
setServo(RightFoot, 120);
delay(300);
homePosition();
}
// Sideways shuffle
for (int i = 0; i < 3; i++) {
setServo(LeftLeg, 70);
setServo(RightLeg, 110);
setServo(LeftFoot, 80);
setServo(RightFoot, 100);
delay(200);
setServo(LeftLeg, 110);
setServo(RightLeg, 70);
setServo(LeftFoot, 100);
setServo(RightFoot, 80);
delay(200);
homePosition();
}
// Return to home position
homePosition();
}
void loop() {
homePosition();
delay(500);
hello();
delay(5000);
for (int i = 0; i < 5; i++) {
walk();
}
delay(5000);
dance();
delay(5000);
}





Using the circuit diagram above, connect the PCA9685 16-Channel Servo Motor Driver to the Raspberry Pi Pico. Next, connect the Ultrasonic Sensor to the Raspberry Pi Pico. Refer to the circuit diagram to create the power source using an LM7805 IC and a 9V battery.
Pro Tip: To simplify the connections and reduce wiring complexity, consider using a PCB board, as we have done, to streamline the circuit assembly. Gerber File: https://drive.google.com/file/d/1AsC0VhEfRiCAE09JpRLL3uXAu8ZMR3mb/view?usp=drive_link
A demonstration video of this project can be viewed here: Watch Now
Thank you for your interest in this project. If you have any questions or suggestions for future projects, please leave a comment and I will do my best to assist you.
For business or promotional inquiries, please contact me via email at Email.
I will continue to update this article with new information. Donât forget to follow me for updates on new projects and subscribe to my YouTube channel (YouTube: roboattic Lab) for more content. Thank you for your support.
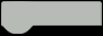