So, you're ready to dive into the world of DIY smartwatches? Fantastic! Let's embark on this tech adventure together. We'll use the UNIHIKER single-board computer from DFRobot, and I'll sprinkle in some humor to keep things lively. Buckle up, because this is going to be a fun ride!
What You'll Need:
UNIHIKER Single Board Computer
The brain of your smartwatch. It's compact, powerful, and comes with a 2.8-inch touchscreen.
3D Printer: To print the watch casing. Make sure you have some filament ready.
Python Scripts: We'll be using various scripts for different applications.
Sensors and Actuators: Depending on your use cases, you might need additional sensors such as heart rate monitors and accelerometers.
Step-by-Step Guide:
1. Design the Watch Case:
Fire up your 3D design software and create a sleek, futuristic watch case. Think Iron Man meets James Bond. Here is my watch case for UNIHIKER.
2. Assemble the Hardware:
Once the case is ready, it's time to assemble the hardware. Place the UNIHIKER board inside the case, ensuring the touchscreen is accessible. Connect the battery .
Here is the complete list of prints.
Step 2. Assemble the Hardware:
Once the case is ready, it's time to assemble the hardware. Place the UNIHIKER board inside the case, ensuring the touchscreen is accessible.
Connect the battery to the charger module.
Assemble the battery inside the case.
Here is the final output.
3.Load the Python Scripts:
Now, let's get those Python scripts up and running. Open the file share on the UNIHIKER and copy the complete GIT folder.
pradeeplogu0/WatchHIKER: PyScripts for WtachHIKER
Here are some cool use cases
Heart Rate Monitor
: Keep track of your heart rate and ensure you're not overdoing it at the gym. Or, you know, check if your heart skips a beat when you see your crush. Step Counter: Count your steps and set daily goals. Perfect for those who need a little motivation to get off the couch. Weather Station: Get real-time weather updates on your wrist. Never get caught in the rain without an umbrella again. Notification Unit: Receive notifications from your phone directly on your smartwatch. Stay connected without constantly checking your phone. SOS Trigger: In emergencies, send an SOS signal to your location. Safety first! Time-Lapse Camera: Capture stunning time-lapse videos right from your wrist. Perfect for those epic sunset shots. Traffic Detector: Get real-time traffic updates and avoid those pesky jams. Duco Coin Monitor: Keep track of your cryptocurrency investments. Because who doesn't want to be a crypto millionaire?
1. HeartRate_Monitor
Imagine you're training for a marathon. The heart rate monitor helps you maintain the optimal heart rate zone for endurance training. It alerts you if you're pushing too hard or not enough, ensuring you get the most out of your training sessions. Plus, it's a great way to monitor your recovery after workouts.
To build this system we need two more components.
Beetle ESP32 C3.MAX100 Heart Rate sensor.
First, we have to program the Beetle ESP32 C3 to read the data from the Heart Rate sensor via I2C communication and it should write the data to the serial port. Then we will use UNIHIKER to read and plot the SPO2 and Heart Rate data.
Here is the simple Arduino script that prints the Heart Rate and SPO2 measurements.
#include <Wire.h>
#include "MAX30100_PulseOximeter.h"
#define REPORTING_PERIOD_MS 1000
PulseOximeter pox;
uint32_t tsLastReport = 0;
void setup()
{
Serial.begin(115200);
Serial.print("Initializing pulse oximeter..");
if (!pox.begin()) {
Serial.println("FAILED");
for (;;);
} else {
Serial.println("SUCCESS");
}
}
void loop()
{
pox.update();
if (millis() - tsLastReport > REPORTING_PERIOD_MS) {
Serial.print("Heart rate:");
Serial.print(pox.getHeartRate());
Serial.print("bpm / SpO2:");
Serial.print(pox.getSpO2());
Serial.println("%");
tsLastReport = millis();
}
}
Here is the serial terminal response:
Now let's get a Python script to decode and populate the data.
Next, we can run this script on the UNIHIKER.
Here is the demo using the UNIHIKER smartwatch.
2. Step Counter
You're on a mission to lead a healthier lifestyle. The step counter motivates you to reach your daily step goals.
UNIHIKER is already equipped with a Gyroscope and Accelerometer sensor. So, we can use that to count the steps. Here is the simple sketch from the UNIHIKER example.
import tkinter as tk
from tkinter import ttk
from pinpong.extension.unihiker import *
from pinpong.board import Board,Pin
from unihiker import GUI
import time
class StepCounterGUI:
def __init__(self):
self.root = tk.Tk()
self.root.title("Step Counter")
self.root.geometry("240x320")
self.root.configure(bg="#1e1e1e")
self.steps = 0
self.setup_ui()
Board().begin()
self.update_values()
def setup_ui(self):
# Accelerometer Frame
acc_frame = ttk.LabelFrame(self.root, text="Accelerometer")
acc_frame.pack(fill="x", padx=5, pady=5)
self.acc_labels = {}
for axis in ['X', 'Y', 'Z']:
label = tk.Label(
acc_frame,
text=f"{axis}: 0.00",
font=("Arial", 12),
bg="#1e1e1e",
fg="#00ff00"
)
label.pack(pady=2)
self.acc_labels[axis] = label
# Gyroscope Frame
gyro_frame = ttk.LabelFrame(self.root, text="Gyroscope")
gyro_frame.pack(fill="x", padx=5, pady=5)
self.gyro_labels = {}
for axis in ['X', 'Y', 'Z']:
label = tk.Label(
gyro_frame,
text=f"{axis}: 0.00",
font=("Arial", 12),
bg="#1e1e1e",
fg="#00ff00"
)
label.pack(pady=2)
self.gyro_labels[axis] = label
# Steps Frame
steps_frame = ttk.LabelFrame(self.root, text="Steps")
steps_frame.pack(fill="x", padx=5, pady=5)
self.steps_label = tk.Label(
steps_frame,
text="0",
font=("Arial", 24, "bold"),
bg="#1e1e1e",
fg="#00ff00"
)
self.steps_label.pack(pady=10)
# Status bar
self.status_bar = tk.Label(
self.root,
text="Running",
bg="#1e1e1e",
fg="#ffffff",
bd=1,
relief=tk.SUNKEN
)
self.status_bar.pack(side=tk.BOTTOM, fill=tk.X)
def update_values(self):
try:
# Update accelerometer values
acc_x = accelerometer.get_x()
acc_y = accelerometer.get_y()
acc_z = accelerometer.get_z()
self.acc_labels['X'].config(text=f"X: {acc_x:.2f}")
self.acc_labels['Y'].config(text=f"Y: {acc_y:.2f}")
self.acc_labels['Z'].config(text=f"Z: {acc_z:.2f}")
# Update gyroscope values
gyro_x = gyroscope.get_x()
gyro_y = gyroscope.get_y()
gyro_z = gyroscope.get_z()
self.gyro_labels['X'].config(text=f"X: {gyro_x:.2f}")
self.gyro_labels['Y'].config(text=f"Y: {gyro_y:.2f}")
self.gyro_labels['Z'].config(text=f"Z: {gyro_z:.2f}")
# Update step count
if accelerometer.get_strength() > 1.5:
self.steps += 1
self.steps_label.config(text=str(self.steps))
self.status_bar.config(text=f"Last update: {time.strftime('%H:%M:%S')}")
except Exception as e:
self.status_bar.config(text=f"Error: {str(e)}")
self.root.after(100, self.update_values)
def run(self):
self.root.mainloop()
if __name__ == "__main__":
app = StepCounterGUI()
app.run()
3. Online WeatherStation
You're planning a weekend camping trip. The online weather station on your smartwatch provides real-time weather updates, helping you decide the best time to set up camp and avoid any unexpected weather changes. It also helps you pack appropriately, ensuring you have the right gear for the conditions.
This Python script can read the weather data from the open weather API and show it to you. You can add as many cities as you want.
Here is the integration with the Windows environment.
Here is the response from the UNIHIKER Smartwatch.
4. Safety Notification Unit
The UNIHIKER's notification unit discreetly alerts you to any detected movement in your house. You can quickly glance at your wrist to see if it's urgent, allowing you to stay focused on your safety.
To build this system we need two components.
Beetle ESP32 C660Ghz Human Detection Sensor
Here is the Node-Red flow to get and publish data to the cloud.
Then we can use Python to read back the cloud data and populate the GUI.
5. SOS Trigger
You're hiking in a remote area and accidentally twist your ankle. With the SOS trigger, you can quickly send an emergency alert with your location to a trusted contact or emergency services. This feature provides peace of mind, knowing that help is just a button press away in case of an emergency.
6. IP Camera Monitor
In today's fast-paced world, security and surveillance are paramount. Imagine a solution that combines cutting-edge technology with simplicity and efficiency. Enter the UNIHIKER single-board computerâa compact powerhouse designed to elevate your IP camera monitoring system to new heights.
Applications
Home Security: Keep your family and property safe with 24/7 monitoring and instant alertsBusiness Surveillance: Protect your assets and ensure the safety of your employees with a reliable surveillance system.Industrial Monitoring: Oversee large industrial sites with ease, ensuring operational efficiency and security.
7. Traffic Detector
You're heading to an important meeting and want to avoid traffic delays. The traffic detector provides real-time traffic updates, helping you choose the fastest route. You arrive at your meeting on time, stress-free, and ready to impress.
8. Duco Coin Miner Monitor and Crypto Monitor
You're an active cryptocurrency trader and want to stay updated on market trends. The Duco coin monitor on your smartwatch provides real-time updates on the value of your cryptocurrencies. You can quickly check your investments and make informed decisions, even when you're on the go.
In the dynamic world of cryptocurrency, staying updated with real-time data is crucial. Imagine having a compact, powerful device that keeps you informed about your crypto investments anytime, anywhere. Introducing the UNIHIKER single-board computerâa revolutionary tool designed to enhance your cryptocurrency monitoring experience.
9. AQI Monitor
You live in a city with fluctuating air quality levels. The air quality monitor on your smartwatch provides real-time data on pollution levels. You use this information to decide when it's safe to go for a run or when to stay indoors, protecting your health and well-being.
10. Smart Watch
You like to express your personality through your accessories. With customizable watch faces, you can switch between different designs based on your mood or the occasion. Whether it's a professional look for work, a sporty design for workouts, or a fun, colorful face for weekends, your smartwatch always matches your style.
11. Audio Recorder
You're attending a lecture and want to capture every detail. The audio recorder on your smartwatch allows you to record the lecture and listen to it later, ensuring you don't miss any important information. It's also great for recording personal notes or meetings.
For this noise level detector, we can use the scripts from UNIHIKER examples.
# -*- coding: UTF-8 -*-
# MindPlus
# Python
from unihiker import Audio
import tkinter as tk
from tkinter import ttk
import time
import threading
from datetime import datetime
import os
class AudioRecorder:
def __init__(self):
self.root = tk.Tk()
self.root.title("Audio Recorder")
self.root.geometry("240x320")
self.root.configure(bg="#1e1e1e")
self.audio = Audio()
self.recording = False
self.elapsed_time = 0
self.recordings_dir = "recordings"
if not os.path.exists(self.recordings_dir):
os.makedirs(self.recordings_dir)
self.setup_ui()
def setup_ui(self):
# Main container frame
main_frame = tk.Frame(self.root, bg="#1e1e1e")
main_frame.pack(expand=True, fill="both")
# Toggle button for start/stop
self.toggle_btn = tk.Button(
main_frame,
text="START",
command=self.toggle_recording,
bg="#00ff00",
fg="#000000",
width=20,
height=2,
font=("Arial", 16, "bold")
)
self.toggle_btn.pack(pady=10)
# Status text
self.status_label = tk.Label(
main_frame,
text="Ready to Record",
font=("Arial", 16),
bg="#1e1e1e",
fg="#00ff00"
)
self.status_label.pack(pady=10)
# Recording indicator
self.canvas = tk.Canvas(
main_frame,
width=100,
height=100,
bg="#1e1e1e",
highlightthickness=0
)
self.canvas.pack(pady=10)
self.indicator = self.canvas.create_oval(
25, 25, 75, 75,
fill="#1e1e1e",
outline="#ff0000",
width=2
)
# Timer display
self.timer_label = tk.Label(
main_frame,
text="00:00",
font=("Arial", 24),
bg="#1e1e1e",
fg="#ffffff"
)
self.timer_label.pack(pady=10)
def update_timer(self):
while self.recording:
self.elapsed_time += 1
minutes = self.elapsed_time // 60
seconds = self.elapsed_time % 60
self.timer_label.config(text=f"{minutes:02d}:{seconds:02d}")
time.sleep(1)
def animate_indicator(self):
pulse_state = True
while self.recording:
self.canvas.itemconfig(
self.indicator,
fill="#ff0000" if pulse_state else "#1e1e1e"
)
pulse_state = not pulse_state
time.sleep(0.5)
def toggle_recording(self):
if not self.recording:
# Start Recording
self.recording = True
self.elapsed_time = 0
# Generate unique filename
timestamp = datetime.now().strftime("%Y%m%d_%H%M%S")
self.current_file = os.path.join(self.recordings_dir, f"recording_{timestamp}.wav")
self.status_label.config(text="Recording...")
self.toggle_btn.config(
text="STOP",
bg="#ff0000",
fg="#ffffff"
)
# Start recording in separate thread
threading.Thread(target=self.audio.start_record, args=(self.current_file,), daemon=True).start()
# Start timer and animation
threading.Thread(target=self.update_timer, daemon=True).start()
threading.Thread(target=self.animate_indicator, daemon=True).start()
else:
# Stop Recording
self.recording = False
self.audio.stop_record()
self.status_label.config(text=f"Saved: {os.path.basename(self.current_file)}")
self.toggle_btn.config(
text="START",
bg="#00ff00",
fg="#000000"
)
self.canvas.itemconfig(self.indicator, fill="#1e1e1e")
def run(self):
self.root.mainloop()
if __name__ == "__main__":
app = AudioRecorder()
app.run()
12. Noise Level Detector
You live in a bustling city where noise pollution is a constant concern. The noise level detector on your smartwatch monitors the ambient noise levels around you and alerts you if they exceed safe limits. This feature helps you identify and avoid noisy areas, ensuring a quieter and more peaceful environment. It's especially useful for protecting your hearing and maintaining a calm atmosphere in your daily life.
For this noise level detector, we can use these enhanced scripts from UNIHIKER examples.
# -*- coding: UTF-8 -*-
# MindPlus
# Python
from pinpong.extension.unihiker import *
from pinpong.board import Board,Pin
from unihiker import Audio
import tkinter as tk
from tkinter import ttk
import time
import threading
import math
class NoiseMonitor:
def __init__(self):
self.root = tk.Tk()
self.root.title("Noise Monitor")
self.root.geometry("240x320")
self.root.configure(bg="#1e1e1e")
self.audio = Audio()
self.setup_ui()
self.start_monitoring()
def setup_ui(self):
# Title
self.title_label = tk.Label(
self.root,
text="NOISE MONITOR",
font=("Arial", 16, "bold"),
bg="#1e1e1e",
fg="#00ff00"
)
self.title_label.pack(pady=10)
# Canvas for visualization
self.canvas = tk.Canvas(
self.root,
width=200,
height=150,
bg="#1e1e1e",
highlightthickness=0
)
self.canvas.pack(pady=10)
# Create bars with peak indicators
self.bars = []
self.peaks = []
self.peak_speeds = []
num_bars = 16
for i in range(num_bars):
# Create main bar
bar = self.canvas.create_rectangle(
i*12 + 5, 150,
i*12 + 13, 150,
fill="#00ff00"
)
self.bars.append(bar)
# Create peak indicator
peak = self.canvas.create_rectangle(
i*12 + 5, 150,
i*12 + 13, 148,
fill="#ffffff"
)
self.peaks.append(peak)
self.peak_speeds.append(0)
# Numerical display
self.level_label = tk.Label(
self.root,
text="0",
font=("Arial", 36, "bold"),
bg="#1e1e1e",
fg="#00ff00"
)
self.level_label.pack(pady=10)
# Status bar
self.status_bar = tk.Label(
self.root,
text="Monitoring...",
bg="#1e1e1e",
fg="#ffffff",
bd=1,
relief=tk.SUNKEN
)
self.status_bar.pack(side=tk.BOTTOM, fill=tk.X)
def update_visualization(self, level):
max_height = 150
peak_fall_speed = 0.5
for i, (bar, peak) in enumerate(zip(self.bars, self.peaks)):
# Calculate bar height with some randomness
variation = math.sin(time.time() * 10 + i) * 5
adjusted_level = max(0, min(100, level + variation))
# Calculate height and color
height = max_height - (adjusted_level * 1.2)
# Rainbow color effect
hue = (i / len(self.bars)) * 360
rgb = self.hsv_to_rgb(hue, 1, 1 if adjusted_level > 0 else 0.2)
color = f'#{rgb[0]:02x}{rgb[1]:02x}{rgb[2]:02x}'
# Update bar
self.canvas.coords(bar, i*12 + 5, height, i*12 + 13, max_height)
self.canvas.itemconfig(bar, fill=color)
# Update peak
peak_y = float(self.canvas.coords(peak)[1])
if height < peak_y: # New peak
self.canvas.coords(peak, i*12 + 5, height, i*12 + 13, height + 2)
self.peak_speeds[i] = 0
else: # Peak falling
self.peak_speeds[i] += peak_fall_speed
new_y = min(max_height, peak_y + self.peak_speeds[i])
self.canvas.coords(peak, i*12 + 5, new_y, i*12 + 13, new_y + 2)
# Update level display
self.level_label.config(text=str(int(level)))
# Update status with smooth color transition
if level > 80:
status = "Very Loud!"
color = "#ff0000"
elif level > 60:
status = "Loud"
color = "#ffff00"
else:
status = "Normal"
color = "#00ff00"
self.status_bar.config(text=status, fg=color)
def hsv_to_rgb(self, h, s, v):
h = float(h)
s = float(s)
v = float(v)
h60 = h / 60.0
h60f = math.floor(h60)
hi = int(h60f) % 6
f = h60 - h60f
p = v * (1 - s)
q = v * (1 - f * s)
t = v * (1 - (1 - f) * s)
r, g, b = 0, 0, 0
if hi == 0: r, g, b = v, t, p
elif hi == 1: r, g, b = q, v, p
elif hi == 2: r, g, b = p, v, t
elif hi == 3: r, g, b = p, q, v
elif hi == 4: r, g, b = t, p, v
elif hi == 5: r, g, b = v, p, q
return (
int(r * 255),
int(g * 255),
int(b * 255)
)
def monitor_audio(self):
while True:
try:
level = self.audio.sound_level()
self.root.after(0, self.update_visualization, level)
time.sleep(0.1)
except Exception as e:
print(f"Error: {e}")
time.sleep(1)
def start_monitoring(self):
threading.Thread(target=self.monitor_audio, daemon=True).start()
def run(self):
self.root.mainloop()
if __name__ == "__main__":
app = NoiseMonitor()
app.run()
Conclusion
Building a 3D-printed smartwatch with the UNIHIKER single-board computer is not only a fun project but also a great way to learn about hardware and software integration. Plus, you'll have a unique gadget that you can proudly say you built yourself. So, what are you waiting for? Get started on your smartwatch adventure today!
Happy building, and may your smartwatch be the envy of all your tech-savvy friends!
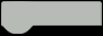