Welcome to Pan-Tilt Playground: Part 1, where I will guide you through building your own Pan-Tilt mechanism. But what exactly is a Pan-Tilt? A Pan-Tilt is a mechanical device that allows for precise control over two axes of rotation—pan (horizontal) and tilt (vertical). These devices are widely used in robotics, photography, surveillance, and automation to achieve flexible and accurate positioning. Whether it's for tracking objects, aiming sensors, or stabilizing cameras, a Pan-Tilt system opens up countless possibilities.
In this tutorial, we'll start from scratch, using a combination of clutch servos, 3D-printed parts, and key hardware components to construct a fully functional Pan-Tilt base. This will form the foundation for future tutorials in the series, where we'll explore Python programming, customization, and various real-world applications.
If you already have a Pan-Tilt device or prefer to skip the build process, feel free to jump ahead to future parts of this series. For those eager to build something hands-on, let's get started!
Note: The servos used are clutch servos. Clutch servos are highly beneficial because they come with built-in protection features that prevent damage, making them ideal for beginners or less experienced drivers. Trust me … I killed so many cheap servos already but these servos are awesome.
The goal of this tutorial is to build a robust, customizable Pan-Tilt mechanism from the ground up. By the end of this guide, you'll have a working Pan-Tilt platform that you can control and modify as needed.

Additional Hardware
I've added a few links. You can also get most parts from other manufacturers. It is important that you stick to the measurements.
- 1x PLA (to print all Pan-Tilt parts)
- 14x RX-M3 * 5.7mm Threaded inserts (Ruthex)
- 2x M3 * 12mm Screws (Purecrea)
- 4x M3 * 14mm Screws
- 4x M3 * 5mm Screws
- 2x M3 * 6mm Screws
- 1x Axial needle bearings 30*47*2mm + 2mm Washers (Axk3047)
- 4x Standoff M3 * 30mm



Before starting the assembly, you'll need to 3D print all the necessary parts using the provided STL files. The recommended material for this project is PLA, which is durable and easy to print. The color of the parts is not important, so feel free to use whatever filament you have on hand.
- Plate temperature: 50 - 60 degrees Celsius
- Nozzle temperature: 200 to 220 degrees Celsius
- Layer height: 0.2 mm for a good balance of detail and speed
Note: Some of the items need supports for printing.
Pan construction

Press all 6 threaded inserts into the ground plate, add servo into ground servo holder and use screws to connect.

Add the 4 standoffs to the ground plate.

Attach the bearing ring to the standoffs with screws. Then place the axial needle bearing in the holder.

Attention: It is recommended to set the pan servo to 0 position, before you continue with the construction! To do this, connect the servo to the driver board (servo pin 2).

from pinpong.board import Board
from pinpong.libs.microbit_motor import DFServo
from time import sleep
PAN_SERVO_PIN: int = 2
PAN_SERVO_START_ANGLE: int = 0
if __name__ == '__main__':
Board("UNIHIKER").begin()
pan_servo = DFServo(PAN_SERVO_PIN)
pan_servo.angle(PAN_SERVO_START_ANGLE)
sleep(1)
Tilt construction

Press 2 threaded inserts into the turntable. Now attach the turntable with the pan servo (servo is on zero degree). Use the parts supplied with the servo for this.

Press 4 threaded inserts into the servo mount. Then attach the tilt servo mount.

Place the tilt servo in the holder. After connect the servo with to the driver board (servo pin 3).

Then screw the cover onto the holder.

Attention: Before you attach the last part to the tilt servo, the servo must be moved to 0 degrees!
from pinpong.board import Board
from pinpong.libs.microbit_motor import DFServo
from time import sleep
TILT_SERVO_PIN: int = 3
TILT_SERVO_START_ANGLE: int = 0
if __name__ == '__main__':
Board("UNIHIKER").begin()
tilt_servo = DFServo(TILT_SERVO_PIN)
tilt_servo.angle(TILT_SERVO_START_ANGLE)
sleep(1)

Press 2 threaded inserts into the object holder. With the tilt servo at 0 degrees, attach the object holder. Use the parts supplied by DFRobot. Be careful and try to avoid any pretense.

Done!!!!
Once you've completed your Pan-Tilt build, a world of possibilities opens up! Here are a few ideas on how you can put your new device to use:
- Camera Control: Attach a camera to your Pan-Tilt and use it for object tracking, time-lapse photography, or live streaming with smooth directional movement.
- Sensor Platform: Mount environmental sensors for weather monitoring, obstacle detection, or air quality measurement, adjusting the sensor’s orientation as needed.
- Light Show: Attach LED lights and build a simple light show system with programmed movement patterns.
Remember, this Pan-Tilt is just a foundation. Be creative and experiment with different attachments and control methods to customize it for your needs. The only limit is your imagination!
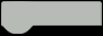