This project demonstrates how to build a non-contact infrared thermometer using an Arduino Uno. The system uses an MLX90614 infrared temperature sensor to measure the temperature of an object within a specific range. An ultrasonic sensor detects if an object is within range, and a buzzer provides auditory feedback based on whether the temperature is above or below a predefined threshold. Optionally, a 16x2 LCD display can be added to show the measured temperature.
Components and supplies
5V Passive Buzzer - Buy Now
16 X 2 LCD display - Buy Now
2S LiPo Battery
1 Jumper-Wires - Buy Now
1 Arduino Uno - Buy Now
1 Breadboard - Buy Now
1 Ultrasonic Sensor - Buy Now
MLX90614 infrared temperature sensor
Step 1: Circuit Connections
MLX90614 Infrared Sensor:
VCC → 5V (Arduino)
GND → GND (Arduino)
SDA → A4 (Arduino - I2C)
SCL → A5 (Arduino - I2C)
Ultrasonic Sensor (HC-SR04):
VCC → 5V (Arduino)
GND → GND (Arduino)
Trig → Digital Pin 9 (Arduino)
Echo → Digital Pin 10 (Arduino)
Buzzer:
Positive → Digital Pin 6 (Arduino)
Negative → GND (Arduino)
16x2 LCD Display (Optional - I2C Module):
VCC → 5V (Arduino)
GND → GND (Arduino)
SDA → A4 (Arduino - I2C)
SCL → A5 (Arduino - I2C)
Step 2: Upload the Code
Upload the Arduino code to read temperature data from the MLX90614 sensor, measure distance using the ultrasonic sensor, and trigger the buzzer based on temperature levels.
Step 3: Testing and Calibration
Ensure the MLX90614 sensor is properly reading temperature values. Open the serial monitor in the Arduino IDE and observe the temperature readings.
Adjust the ultrasonic sensor range to detect objects within the desired distance (default: 10 cm).
Verify buzzer tones: Check if the buzzer emits different sounds for high and low temperatures.
Optional: Connect an LCD display to show real-time temperature readings.
Step 4: Running the System
Power the Arduino using a 7-12V DC battery.
Place an object within range of the ultrasonic sensor.
Observe the temperature reading and listen to the buzzer feedback.
If an LCD is connected, verify the display of temperature values.
Troubleshooting and Optimization
Power Supply: Ensure the battery is fully charged.
Sensor Calibration: Adjust the MLX90614 and ultrasonic sensor placements for better accuracy.
Buzzer Sound: Modify the frequency values in the code for distinct alerts.
LCD Display Issues: Check I2C connections and install the required libraries in the Arduino IDE.

#include <Wire.h>
#include <Adafruit_MLX90614.h>
#include <NewPing.h>
Adafruit_MLX90614 mlx = Adafruit_MLX90614();
#define TRIG_PIN 9
#define ECHO_PIN 10
#define BUZZER_PIN 6
#define MAX_DISTANCE 100
NewPing sonar(TRIG_PIN, ECHO_PIN, MAX_DISTANCE);
void setup() {
Serial.begin(9600);
mlx.begin();
pinMode(BUZZER_PIN, OUTPUT);
}
void loop() {
delay(500);
int distance = sonar.ping_cm();
if (distance > 0 && distance <= 10) {
delay(2000); // Allow time for stable reading
float temp = mlx.readObjectTempC();
Serial.print("Temperature: ");
Serial.print(temp);
Serial.println(" °C");
if (temp > 30.0) {
tone(BUZZER_PIN, 1000, 500); // High temperature alert
} else {
tone(BUZZER_PIN, 500, 500); // Low temperature alert
}
}
}
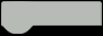