
This guide demonstrates how to build a Bluetooth-controlled car using an ESP32 module, an L298N motor driver, and a readily available 4WD car chassis kit. The project utilizes the freely available "Dabble" mobile app for control, compatible with both Android and iOS devices.
Components and Supplies
4WD Car Chassis Kit - BUY NOW
ESP32 Module - BUY NOW
L298N Motor Driver Module - BUY NOW
Jumper-Wires - BUY NOW
7-12 V DC Battery (in our case lipo 2s battery)
Step 1: Assembly
Mounting the Motors and Chassis
Secure the motors onto the chassis using the provided connectors and screws.
Attach and secure the chassis plates.
Wire the motors: connect red wires together and black wires together.
Attach the L298N motor driver to the chassis using double-sided tape.
Step 2: Circuit Connections
L298N Motor Driver to Motors
Right-side motors → OUT1 & OUT2 (L298N)
Left-side motors → OUT3 & OUT4 (L298N)
L298N VCC → 12V Battery
L298N GND → Common GND
ESP32 to L298N Motor Driver
ESP32 receives 5V and GND from the L298N.
Motor control pins connected based on circuit diagram.

Step 3: Uploading the Code
Before uploading the code, ensure you have installed the ESP32 board support package and the Dabble ESP32 library in the Arduino IDE.
Step 4: Connecting with Dabble App
Download & Install the Dabble mobile app.
Pair with ESP32: Connect to "My Bluetooth Car."
Control the Car: Use the app’s gamepad controls for movement.
Final Demonstration
The car responds to Bluetooth commands sent from the Dabble app, allowing real-time control via a smartphone.
#include <DabbleESP32.h>
#define IN1 16
#define IN2 17
#define IN3 18
#define IN4 19
#define ENA 5
#define ENB 6
void setup() {
pinMode(IN1, OUTPUT);
pinMode(IN2, OUTPUT);
pinMode(IN3, OUTPUT);
pinMode(IN4, OUTPUT);
pinMode(ENA, OUTPUT);
pinMode(ENB, OUTPUT);
Dabble.begin("My Bluetooth Car");
}
void loop() {
Dabble.processInput();
}
GamePad.onPressed(GAMEPAD_UP, []() {
moveForward();
});
GamePad.onPressed(GAMEPAD_DOWN, []() {
moveBackward();
});
GamePad.onPressed(GAMEPAD_LEFT, []() {
turnLeft();
});
GamePad.onPressed(GAMEPAD_RIGHT, []() {
turnRight();
});
GamePad.onPressed(GAMEPAD_STOP, []() {
stopMotors();
});
void moveForward() {
digitalWrite(IN1, HIGH);
digitalWrite(IN2, LOW);
digitalWrite(IN3, HIGH);
digitalWrite(IN4, LOW);
}
void moveBackward() {
digitalWrite(IN1, LOW);
digitalWrite(IN2, HIGH);
digitalWrite(IN3, LOW);
digitalWrite(IN4, HIGH);
}
void turnLeft() {
digitalWrite(IN1, LOW);
digitalWrite(IN2, HIGH);
digitalWrite(IN3, HIGH);
digitalWrite(IN4, LOW);
}
void turnRight() {
digitalWrite(IN1, HIGH);
digitalWrite(IN2, LOW);
digitalWrite(IN3, LOW);
digitalWrite(IN4, HIGH);
}
void stopMotors() {
digitalWrite(IN1, LOW);
digitalWrite(IN2, LOW);
digitalWrite(IN3, LOW);
digitalWrite(IN4, LOW);
}
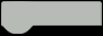