This project demonstrates how to build a simple line-following robot using an Arduino Uno. The robot detects a black line on a white surface using two IR sensors and moves accordingly with the help of an L298N motor driver module. This project is an excellent starting point for robotics enthusiasts and can be further enhanced for advanced applications like obstacle avoidance and path optimization.

Components and supplies
2 IR Sensor Modules - BUY NOW
1 Arduino-Uno - BUY NOW
2WD Car Chassis Kit - BUY NOW
L298N Motor Driver Module - BUY NOW
Jumper-Wires - BUY NOW
7-12 V DC Battery (in our case lipo 2s battery)
Step 1: Circuit Connections
IR Sensors:
VCC → 5V (Arduino)
GND → GND (Arduino)
OUT1 → Digital Pin 11 (Arduino)
OUT2 → Digital Pin 12 (Arduino)
Motor Driver (L298N):
Motor A (Left Wheel) → L298N OUT1 & OUT2
Motor B (Right Wheel) → L298N OUT3 & OUT4
L298N IN1 & IN2 → Digital Pins 5 & 6 (Arduino)
L298N IN3 & IN4 → Digital Pins 9 & 10 (Arduino)
L298N VCC → 7-12V DC Battery
L298N GND → Common GND with Arduino
L298N 5V Output → 5V (Arduino)
Step 2: Upload the Code
Upload the given Arduino code to control the robot's movement based on the IR sensor readings.
Step 3: Testing and Calibration
Before testing the robot, calibrate the IR sensors using their onboard potentiometers:
Adjust the sensor sensitivity so that the LED indicator remains off when over the black line and on when over the white surface.
Ensure the motors rotate in the correct direction when moving forward. If needed, reverse the motor wires connected to the L298N motor driver.
Step 4: Running the Robot
Place black electrical tape on a white surface to create a path.
Turn on the robot using the battery switch.
Observe the robot as it follows the black line.
Troubleshooting and Optimization
Battery Condition: Ensure the battery is charged for optimal performance.
Line Width: Adjust the black tape width if the robot struggles to follow it.
Sensor Placement: Modify the sensor distance to improve detection.
Motor Speed: Adjust the PWM frequency in the code to fine-tune movement.

#define LEFT_SENSOR 11
#define RIGHT_SENSOR 12
#define MOTOR_LEFT_FORWARD 5
#define MOTOR_LEFT_BACKWARD 6
#define MOTOR_RIGHT_FORWARD 9
#define MOTOR_RIGHT_BACKWARD 10
void setup() {
pinMode(LEFT_SENSOR, INPUT);
pinMode(RIGHT_SENSOR, INPUT);
pinMode(MOTOR_LEFT_FORWARD, OUTPUT);
pinMode(MOTOR_LEFT_BACKWARD, OUTPUT);
pinMode(MOTOR_RIGHT_FORWARD, OUTPUT);
pinMode(MOTOR_RIGHT_BACKWARD, OUTPUT);
}
void loop() {
int leftState = digitalRead(LEFT_SENSOR);
int rightState = digitalRead(RIGHT_SENSOR);
if (leftState == LOW && rightState == LOW) {
moveForward();
} else if (leftState == HIGH && rightState == LOW) {
turnRight();
} else if (leftState == LOW && rightState == HIGH) {
turnLeft();
} else {
stopMotors();
}
}
void moveForward() {
digitalWrite(MOTOR_LEFT_FORWARD, HIGH);
digitalWrite(MOTOR_LEFT_BACKWARD, LOW);
digitalWrite(MOTOR_RIGHT_FORWARD, HIGH);
digitalWrite(MOTOR_RIGHT_BACKWARD, LOW);
}
void turnRight() {
digitalWrite(MOTOR_LEFT_FORWARD, HIGH);
digitalWrite(MOTOR_LEFT_BACKWARD, LOW);
digitalWrite(MOTOR_RIGHT_FORWARD, LOW);
digitalWrite(MOTOR_RIGHT_BACKWARD, HIGH);
}
void turnLeft() {
digitalWrite(MOTOR_LEFT_FORWARD, LOW);
digitalWrite(MOTOR_LEFT_BACKWARD, HIGH);
digitalWrite(MOTOR_RIGHT_FORWARD, HIGH);
digitalWrite(MOTOR_RIGHT_BACKWARD, LOW);
}
void stopMotors() {
digitalWrite(MOTOR_LEFT_FORWARD, LOW);
digitalWrite(MOTOR_LEFT_BACKWARD, LOW);
digitalWrite(MOTOR_RIGHT_FORWARD, LOW);
digitalWrite(MOTOR_RIGHT_BACKWARD, LOW);
}
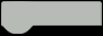