
This is a social distancing detector that makes sure that you are far enough away from people.

The picture above should be sufficient - as they say, a picture is worth a thousand words! If not, the code has wiring comments. The power connections are standard. The Trig pin goes to digital pin 6, and the Echo pin goes to digital pin 5. The piezo VCC goes to digital pin 13.

The code below is the Arduino source code for the sensor. The link is the GitHub repository for the HC-SR04 in case this doesn't work. It would be very helpful if you commented and said what your modifications to the distance sensitivity in the code were. Upload the code in the Arduino IDE.
// HC-SR04 code
int const trigPin = 6;
int const echoPin = 5;
int const buzzPin = 13;
void setup()
{
pinMode(trigPin, OUTPUT); // trig pin triggers the ultrasonic sensor
pinMode(echoPin, INPUT); // echo pin should be input to get pulse width
pinMode(buzzPin, OUTPUT); // buzz pin is output to control buzzer
}
void loop()
{
// Duration will be the input pulse width and distance will be the distance to the obstacle in centimeters
int duration, distance;
// Output pulse with 1ms width on trigPin
digitalWrite(trigPin, HIGH);
delay(1);
digitalWrite(trigPin, LOW);
// Measure the pulse input in echo pin
duration = pulseIn(echoPin, HIGH);
// Distance is half the duration devided by 29.1 (from datasheet)
distance = (duration/2) / 29.1;
// if distance less than 0.5 meter and more than 0 (0 or less means over range)
if (distance <= 100 && distance >= 0) {
// Buzz
digitalWrite(buzzPin, HIGH);
} else {
// Don't buzz
digitalWrite(buzzPin, LOW);
}
// Waiting 60 ms won't hurt any one
delay(60);
}

Plug in your 9v battery to your Arduino (or leave it plugged into the computer) and put your hand in front of the sensor.
You should hear a piercing shriek. If you don't, check your wiring. Most of my problems stem from either loose wires, wires that are not fully inserted into the breadboard, or wrong wiring. The code works, and has been fine-tuned to what works for my sensor. If yours seems to be off, adjust the code to 6 ft/1 m.

You are done! Congratulations!
If you have any problems, don't hesitate to comment them.
Have fun, and merry making!
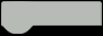
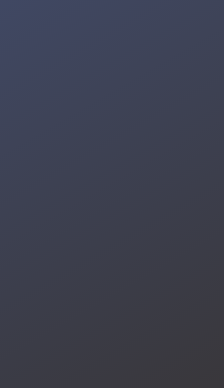