An IoT Moisture sensor that sends moisture data from an Arduino Nano 33 IoT to the Arduino IoT Cloud

Things used in this project
Hardware components
Software apps and online services
Arduino IoT Cloud
Arduino Web Editor
Story
Earlier this year, my parents had our lawn redone. To keep our lawn fresh and green, my dad had to water the lawn every day but had to make sure he was not overwatering the soil, otherwise, the grass would die. To help my dad, I created this small device that could sense the moisture level of the soil and send messages through the Arduino IoT Cloud to my computer, so we could know when to water the lawn.
Notes:
This project is battery powered, so to achieve a secured fit to the Nano 33 IoT, you may need to solder headers or jumper wires onto the battery clip
You must be careful when water is poured over this. If you plan on using it in an outdoor environment as I did, make sure you put a static-proof plastic cover over your nano 33 IoT, moisture sensor, and battery to ensure the safety of your device. If you plan on using this indoors, like the cover picture, you do not need to have a cover, but you must be careful when pouring water over the moisture sensor as there are components fixed to it. Water away from the moisture sensor and keep the soil underneath the white line for safety purposes, else you risk damaging your sensor and board.
Link to Arduino's Getting Started guide for Arduino IoT Cloud if you need: https://docs.arduino.cc/cloud/iot-cloud/tutorials/iot-cloud-getting-started
Building Sequence:
1. Connect a moisture sensor to the Nano 33 IoT board. The red wire goes to 3.3V, the black wire goes to GND, and the yellow wire goes to Analog Pin A1 on the board. You can choose any analog pin you like, however, make sure you change the pin number inside the code. Change the number inside the analogRead function inside the code. A snippet of the lines is below.
//Read data from Analog Pin 1 on Nano 33 IoT and print to Serial Monitor for debugging
soilMoistureLevel = analogRead(1);
Serial.println(soilMoistureLevel);
2. Next, connect a cable to your Nano 33 IoT board and create the variables listed below inside your thing
-Class Variable
-string message
-int moistureLevel
-CloudPercentage moisturePercent
3. Then, set up your Nano 33 IoT as the device for your thing and enter your network credentials in the fields below.

4. Copy and Paste the code below into the sketch tab of your thing and upload it to your Arduino. Once it has been uploaded, open the serial monitor to check whether the moisture sensor is functioning or not. If it is not, try troubleshooting by resetting the code back to its original state or checking the moisture sensor's pin if you had changed the moisture sensor's pin
5. Next, create a dashboard with a few widgets. Below is the dashboard I use.
Here is the list that shows what variable each widget is connected to
-PLANT MOISTURE widget ==> moisturePercent
-MOISTURE VALUE widget ==> moistureValue
-the graph in the bottom left ==> moisturePercent
-MESSENGER widget ==> message

6. Reload the dashboard. if you see the data in the moisture value widget fluctuate by a few digits, then you have completed this project. Congratulations! If you have any problems, questions, or advice, please comment below and I will get back to you as soon as possible.
A demonstration of the project is below.
Thanks for reading!
Schematics
Circuit Diagram using Paint 3D (since I don't have fritzing)

Code
#include "thingProperties.h"
int soilMoistureLevel;
int soilMoisturePercent;
int airValue = 830;
int waterValue = 360;
void setup() {
// Initialize serial and wait for port to open:
Serial.begin(9600);
// Defined in thingProperties.h
initProperties();
// Connect to Arduino IoT Cloud
ArduinoCloud.begin(ArduinoIoTPreferredConnection);
/*
The following function allows you to obtain more information
related to the state of network and IoT Cloud connection and errors
the higher number the more granular information you’ll get.
The default is 0 (only errors).
Maximum is 4
*/
setDebugMessageLevel(2);
ArduinoCloud.printDebugInfo();
}
void loop() {
ArduinoCloud.update(); // Update the Cloud's data
//Read data from Analog Pin 1 on Nano 33 IoT and print to Serial Monitor for debugging
soilMoistureLevel = analogRead(1);
Serial.println(soilMoistureLevel);
//Convert soilMoistureLevel integer into percentage by using air and water values defined above and mapping with 0 - 100
soilMoisturePercent = map(soilMoistureLevel, airValue, waterValue, 0, 100);
Serial.println(soilMoisturePercent); //Print to Serial Monitor for debugging
//Update Arduino IoT Cloud values
moistureLevel = soilMoistureLevel;
moisturePercent = soilMoisturePercent;
//If the moisture percentage is less than 30%
if(moisturePercent < 30)
{
//Send message to Arduino Cloud messenger on dashboard
message = "Water Levels Low. Watering Required!";
}
//If the moisture percentage is greater than 50%
if(moisturePercent > 50)
{
//Send message to Arduino Cloud messenger on dashboard
message = "Water Levels Sufficient.";
}
}
//End of Code
The article was first published in hackster, August 31, 2021
cr: https://www.hackster.io/buddhimaan/iot-moisture-sensor-788327
author: buddhimaan
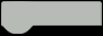