In this project we are going to build a safety and security system for an industry or factory.
Things used in this project
Hardware components
Software apps and online services
Arduino IDE
Hand tools and fabrication machines
Hot glue gun (generic)
Soldering iron (generic)
Solder Wire, Lead Free
Digilent Mastech MS8217 Autorange Digital Multimeter
Story
Introduction
In this project we are going to make a project that is related to Industrial/Factory safety and security system. Here we will build up a demo project for this. But it can be implemented in real life.
Hardware list
There are a lot of hardware we have used in this project. All the hardware list has given below:
-Arduino Mega
-Arduino Nano
-Breadboard
-Potentiometer (1K)
-Gas Sensor Module (Model-MQ4 )
-Vibration Sensor Module (Model- MOD-00187)
-PIR Motion Sensor
-Dust Sensor (Model-SEN-00170)
-Temperature Sensor (dht22)
-Buzzer
-Fan
-LCD Monitor (20x4)
-Servo Motor (SG90)
-4 x 4 Matrix Keypad
-LED (5 mm) --> Green-1 and Red-2
Explanation
First we we build up a secured door for the industry/factory. It will be a password protected door. If anyone press the wrong password it would be alert everyone through the buzzer. Only the door will open when someone enter the correct password.
Next we have to take care some machines of the industry or factory. When operating some machines, it can vibrate more than normal situation. So, our vibration sensor will sense the vibration and alert the control room to turn off the machines or speed down to the machines.
Some rooms will be store some important things in a factory or industry. So there will maybe few employees have the right to enter those room. So, there will be a motion sensor in these rooms. So, any unauthorized persons will not be entered these rooms. If authorize persons enter the room they will turn off the motion sensor before entering the room.
Temperature plays an important roles in an industry or factory. So, every time we need to monitor the temperature of machines or products. From the control room they can control the temperature by turning on or off the fans.
Gas emission will also be monitor properly in an industry or factory. Some gas are toxic and that will not good for human health. So, when gas emits in a inaccurate level an exhaust fan will turn on automatically and emit the gases from the industry.
Video
We have provide all the codes and whole circuit diagram in this project. In this project we have used two micro-controller one is Arduino Mega and Arduino Nano. For each board we have provided 2 codes in the code section.
Links
Github Project links: https://github.com/MakerBuddy/Smart_Industry
Custom parts and enclosures
Industrial Safety and Security System
示意图
Industrial Safety and Security System

Industrial Safety Code
C/C++
// Library Files
#include <DHT.h>
#include <LiquidCrystal.h>
#include <Servo.h>
// LCD Display
#include <LiquidCrystal.h> // includes the LiquidCrystal Library
LiquidCrystal lcd(6, 5, 4, 3, 2, 1); // Creates an LC object. Parameters: (rs, enable, d4, d5, d6, d7)
//Temperature
#define DHTPIN 11 // what pin we're connected to
#define DHTTYPE DHT22 // DHT 22 (AM2302)
DHT dht(DHTPIN, DHTTYPE);
int chk;
float hum; //Stores humidity value
float temp; //Stores temperature value
//Motion Sensor
int ledpin1 = 8;// Digital pin n D6
int pir1;
int sensor1 = 10 ;//motion senson1 on D7
//Gas Sensor
const int smoke = A0;
const int exFan = 7; //exFan
const int threshold = 400;//gas sensor
//Vibration sensor
int vibration = A8; //vibration sensor D0
int LedPin3 = 9; //vibration in led D1
//dust sensor
int measurePin = A15;
int ledPower = 52;
unsigned int samplingTime = 280;
unsigned int deltaTime = 40;
unsigned int sleepTime = 9680;
float voMeasured = 0;
float calcVoltage = 0;
float dustDensity = 0;
void setup() {
//Motion
pinMode(sensor1, INPUT); // declare sensor as input
pinMode(ledpin1, OUTPUT); // declare LED as output
//gas sensor
pinMode(smoke, INPUT);
pinMode(exFan, OUTPUT); //Buzzpin for fire alarm
//temperature
dht.begin();
//Vibration
pinMode (vibration, INPUT);
pinMode(LedPin3, OUTPUT);
//Display
lcd.begin(16, 2);
lcd.print("Smart Industry");
// Serial.begin(9600);/
}
void loop() {
gasSensor();
temperature();
vibration_sensor();
motionDetected1();
lcd.clear();
lcd.println(temp);
}
//Vibration
void vibration_sensor() {
int vibrationvalue = analogRead(vibration);
// Serial.println(vibrationvalue);/
if (vibrationvalue == 0) {
digitalWrite(LedPin3, HIGH);
// Serial.println("\n Vibration");/
delay(1000);
}
else {
digitalWrite(LedPin3, LOW);
Serial.println("\n No Vibration");
delay(1000);
}
// delay(500);
}
//temperature
void temperature() {
delay(1000);
//Read data and store it to variables hum and temp
hum = dht.readHumidity();
temp = dht.readTemperature();
// Serial.print("Humidity: ");
// Serial.print(hum);
// Serial.print(" %, Temp: ");
// Serial.print(temp);
// Serial.println(" Celsius");
delay(1000);
}
//Motion Sensor Detection
void motionDetected1() {
pir1 = digitalRead (sensor1);
// Serial.println(pir1);
if (pir1 == HIGH) {
digitalWrite(ledpin1, HIGH);
// Serial.println("\n Motion Detected");/
// Serial.println(pir1);
delay(50);
digitalWrite(ledpin1, LOW);
}
else {
digitalWrite(ledpin1, LOW);
// Serial.println(" Motion Not Detected");/
//Serial.println(pir1);
delay(50);
}
}
//gas sensor
void gasSensor() {
int analogValue = analogRead(smoke);
// Serial.println("\n Gas value = ");/
// Serial.println(analogValue);/
if (analogValue > threshold) {
digitalWrite(exFan, HIGH);
}
else if (analogValue == threshold) {
digitalWrite(exFan, LOW);
delay(400);
//digitalWrite(Buzzerpin, LOW);
}
else {
digitalWrite(exFan, LOW);
}
// Serial.println(analogRead(A0));
delay(500);
}
Industrial Security Code (Only for Door)
C/C++
#include <Keypad.h>
#include <LiquidCrystal.h>
#include <Servo.h>
Servo myservo;
int pos = 0; // LCD Connections
const byte rows = 4;
const byte cols = 4;
char key[rows][cols] = {
{'1', '2', '3', 'A'},
{'4', '5', '6', 'B'},
{'7', '8', '9', 'C'},
{'*', '0', '#', 'D'}
};
byte rowPins[rows] = {9, 8, 7, 6};
byte colPins[cols] = {5, 4, 3, 2};
Keypad keypad = Keypad(makeKeymap(key), rowPins, colPins, rows, cols);
const char* password = "4567";
int currentposition = 0;
int redled = 10;
int greenled = 11;
int invalidcount = 12;
void setup()
{
// keypad
Serial.begin(9600);
pinMode(redled, OUTPUT);
pinMode(greenled, OUTPUT);
myservo.attach(12); //SERVO ATTACHED//
}
void loop()
{
mykeypad();
}
void mykeypad() {
char code = keypad.getKey();
if (code)
Serial.print(code);
if (code != NO_KEY) {
if (code == password[currentposition]) {
++currentposition;
if (currentposition == 4) {
digitalWrite(greenled, HIGH);
unlockdoor();
currentposition = 0;
digitalWrite(greenled, LOW);
}
}
else {
++invalidcount;
incorrect();
currentposition = 0;
}
}
}
//Door Open
void unlockdoor()
{
delay(900);
for (pos = 90; pos >= 0; pos -= 5) {
myservo.write(pos); // tell servo to go to position in variable 'pos'
delay(5); // waits 15ms for the servo to reach the position
}
delay(3000);
for (pos = 0; pos <= 90; pos += 5) { // in steps of 1 degree
myservo.write(pos); // tell servo to go to position in variable 'pos'
delay(15);
currentposition = 0;
}
}
//Wrong Code
void incorrect()
{
delay(500);
digitalWrite(redled, HIGH);
delay(3000);
digitalWrite(redled, LOW);
}
Industrial Safety and Security System
MakerBuddy / Smart_Industry
A smart security and safety system of an industry. This is a Arduino based project. — Read More
The article was first published in hackster, September 10, 2021
cr: https://www.hackster.io/makerbuddy/industrial-safety-security-system-ed77c1
author:
Khaled Md. Saifullah;
Shahadat Hossain Afridi;
Avijit Datta
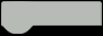