You will be able to know the I2C address of your module that's connected to SDA & SCL Arduino by using I2C scanner code.

Things used in this project
Hardware components
Story
When you are going to use I2C modules for your Arduino Projects, sometimes the manufacturer didn't give the address of I2C device inside the chip. It can be a serious problem if the address is unknown or you write the wrong address.
This method is used for scanning the I2C Device inside your module that connected to I2C bus in Arduino (SDA, SCL). In Arduino Uno / Nano / Pro Mini I2C bus is connected to A4 (SDA) and A5 (SCL), for Arduino Mega 2560 I2C bus is connected to D20 (SDA) and D21 (SCL)
Here I am going to show you how to scan I2C address with a simple code.
Schematics
Wiring diagram of Arduino and GY-87 Module

Code
I2C Scanner Arduino Code
C/C++
Write your code in Arduino IDE Software, save it. Select the port (i.e COM3) and choose your Arduino board type (i.e Arduino Uno / Genuino) then compile it to your Arduino.
/ ---------------------------------------------------------------- /
// Arduino I2C Scanner
// Re-writed by Arbi Abdul Jabbaar
// Using Arduino IDE 1.8.7
// Using GY-87 module for the target
// Tested on 10 September 2019
// This sketch tests the standard 7-bit addresses
// Devices with higher bit address might not be seen properly.
/ ---------------------------------------------------------------- /
#include <Wire.h> //include Wire.h library
void setup()
{
Wire.begin(); // Wire communication begin
Serial.begin(9600); // The baudrate of Serial monitor is set in 9600
while (!Serial); // Waiting for Serial Monitor
Serial.println("\nI2C Scanner");
}
void loop()
{
byte error, address; //variable for error and I2C address
int nDevices;
Serial.println("Scanning...");
nDevices = 0;
for (address = 1; address < 127; address++ )
{
// The i2c_scanner uses the return value of
// the Write.endTransmisstion to see if
// a device did acknowledge to the address.
Wire.beginTransmission(address);
error = Wire.endTransmission();
if (error == 0)
{
Serial.print("I2C device found at address 0x");
if (address < 16)
Serial.print("0");
Serial.print(address, HEX);
Serial.println(" !");
nDevices++;
}
else if (error == 4)
{
Serial.print("Unknown error at address 0x");
if (address < 16)
Serial.print("0");
Serial.println(address, HEX);
}
}
if (nDevices == 0)
Serial.println("No I2C devices found\n");
else
Serial.println("done\n");
delay(5000); // wait 5 seconds for the next I2C scan
}
The article was first published in hackster, September 10, 2019
cr: https://www.hackster.io/abdularbi17/how-to-scan-i2c-address-in-arduino-eaadda
author: Arbi Abdul Jabbaar
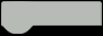