Anemometer is an instrument that measures the speed of the wind. It consists of a series of cups mounted at the end of arms that rotate in the wind. The speed with which the cups rotate indicates the wind speed.
In this case, the speed of rotation of the Cups is proportional to the voltage on the yellow wire. This voltage ranges from 0 V to 5 V depending on the wind speed from 0 to 100 km / h. Then we bring this signal to the analog input of the microcontroller. We read the current wind speed on a scale consisting of a strip of 50 RGB Leds marked in km / h and m / s. The color of the corresponding Led changes from blue at low wind speeds, then to shades of green, to red and magenta at very high wind speeds. At wind speeds greater than 60km / h White warning LED and audible signal are activated.
The device is very simple to build and contains only a few components:
Let me mention that the Anemometer signal is compatible with 5 V microcontrollers, so if we use a 3.3 V microcontroller such as ESP32, we should also use a level converter board. The warning LED, buzzer, and RGB LED strip are connected to the digital pins of the microcontroller. The button is used to select between "line" and "dot" mode to display the value.
Arduino code is relatively simple and can be used to display the value of any sensor that has an analog output. It is only necessary to make a change in the line:
" int w = map(sensorValue, 0, 1023, 0, NUM_PIXEL); "
based on the voltage range generated by the sensor. If the range is from 0 to 5 V as in this case, then there is no need for any changes.
The Anemometer Kit comes with a 4m cable, but I extended it to 12m and it worked perfectly. In terms of accuracy, I compared it with my professional anemometers brand "TFA" and "La Crosse", and the results were almost identical, which means that at least in my case the anemometer is factory calibrated. If there are any deviations, it can be easily fixed with a small modification of the line of code indicated earlier.

I will use 12cm fan to generate air flow. Depending on the distance, we will also change the flow. When switched on, the Anemometer operates in "TEST" mode, and now all the diodes are switched on successively one by one to the maximum and then returned. After that, the device is started in Dot mode, and only one LED diode show the value of the wind speed. If we press the button, the device switches to Line mode and the value is displayed as a line . When the wind speed reaches a certain speed previously defined in the code, a warning sound and light signal is activated
Finally, the device is placed in a suitable box made of PVC material with a thickness of 3 mm, and the scale is drawn with a marker.

#include "Wire.h" // imports the wire library for talking over I2C
#include <Adafruit_NeoPixel.h>
int buttonPin = A1; // momentary push button on pin 0
int oldButtonVal = 0;
#define NUM_PIXEL 64
#define PIN 9
Adafruit_NeoPixel strip = Adafruit_NeoPixel(NUM_PIXEL, PIN, NEO_GRB + NEO_KHZ800);
int nPatterns = 3;
int lightPattern = 1;
int out = 0 ;
int w = 0 ;
int sensorValue = 0;
void setup()
{
Serial.begin(9600); //turn on serial monitor
strip.begin();
clearStrip();
strip.show();
strip.setBrightness(15);
testing();
pinMode(buttonPin, INPUT);
digitalWrite(buttonPin, HIGH); // button pin is HIGH, so it drops to 0 if pressed
}
void testing(){
for(int L = 0; L<64; L++) {
clearStrip();
strip.setPixelColor(L,wheel(((205+(L*3)) & 255)));//Gradient from blue (cold) to green (ok) to red (warm), first value here 205 = start color, second value here 42 = end color
strip.show();
delay(100);
}
for(int L = 63; L>=0; L--) {
clearStrip();
strip.setPixelColor(L,wheel(((205+(L*3)) & 255)));//Gradient from blue (cold) to green (ok) to red (warm), first value here 205 = start color, second value here 42 = end color
strip.show();
delay(100);
}
}
void dot() {
sensorValue = analogRead(A0);
int w = map(sensorValue, 0, 1023, 0, NUM_PIXEL);
for(uint16_t L = 0; L<w; L++) {
clearStrip();
strip.setPixelColor(L,wheel(((205+(L*3)) & 255))); //Gradient from blue (cold) to green (ok) to red (warm), first value here 205 = start color, second value here 42 = end color
}
strip.show(); //Output on strip
delay(100);
}
void line() {
sensorValue = analogRead(A0);
int w = map(sensorValue, 0, 1023, 0, NUM_PIXEL);
for(uint16_t L = 0; L<w; L++) {
strip.setPixelColor(L,wheel(((205+(L*3)) & 255))); //Gradient from blue (cold) to green (ok) to red (warm), first value here 205 = start color, second value here 42 = end color
}
strip.show(); //Output on strip
clearStrip();
delay(100);
}
void loop() {
// read that stapressurete of the pushbutton value;
int buttonVal = digitalRead(buttonPin);
if (buttonVal == LOW && oldButtonVal == HIGH) {// button has just been pressed
lightPattern = lightPattern + 1;
}
if (lightPattern > nPatterns) lightPattern = 1;
oldButtonVal = buttonVal;
switch(lightPattern) {
case 1:
dot();
break;
case 2:
line();
break;
}
if (sensorValue > 150)
{tone(11,1000);
delay(50);
noTone(11);
}
else {delay(50);
}
}
//Color wheel ################################################################
uint32_t wheel(byte WheelPos) {
if(WheelPos < 85) {
return strip.Color(WheelPos * 3, 255 - WheelPos * 3, 0);
}
else if(WheelPos < 205) {
WheelPos -= 85;
return strip.Color(255 - WheelPos * 3, 0, WheelPos * 3);
}
else {
WheelPos -= 205;
return strip.Color(0, WheelPos * 3, 255 - WheelPos * 3);
}
}
void clearStrip(){
for(int i = 0; i < NUM_PIXEL; i++) {
strip.setPixelColor(i, 0);
}
}
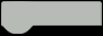