This is a simple digital clock using the DS3231 RTC module and the LilyGo TTGO T-Display. The DS3231 uses the standard library by Andrew Wickert found in the Arduino IDE. The T-Display uses a dedicated TFT_eSPI library found here https://github.com/Xinyuan-LilyGO/TTGO-T-Display/tree/master/TFT_eSPI
Relocate your original TFT_eSPI folder from Documents/Arduino/library and replace with this one till you have finished using the T-Display, then use your original TFT_eSPI library.
The SDA pins on the T_Display is Pin 21
The SCL pin is Pin 22.
The RTC also uses the 3.3v VCC output Pin
HARDWARE LIST
1 DS3231 RTC
TTGO T_Display
CODE
#include <SPI.h>
#include <TFT_eSPI.h> //https://github.com/Xinyuan-LilyGO/TTGO-T-Display/tree/master/TFT_eSPI
#include <DS3231.h>
#include <Wire.h>
//#define TFT_CS 5
//#define TFT_RST 23
//#define TFT_DC 16
//#define SDA 21
//#define SCL 22
DS3231 myRTC;
TFT_eSPI tft = TFT_eSPI(135,240);
byte HOURS;
byte L_HOURS = 0;
byte MINS;
byte L_MINS = 0;
byte SECS;
byte L_SECS = 0;
byte DOW;
byte L_DOW = 3;
byte Date;
byte L_Date = 4;
byte Temp;
byte L_Temp;
bool h12Flag,pmFlag; // Not Used Here
bool century = false;
String Dow []= {"0","Monday","Tuesday","Wednesday","Thursday","Friday","Saturday","Sunday" };
byte Spc []= {0,49,34,14,38,49,38,49}; // X position of Weekday
byte L_Month = 1;
byte Months;
void setup() {
Serial.begin(57600);
Wire.begin();
tft.init();
tft.setRotation(3);
tft.fillScreen(TFT_BLACK);
tft.setTextColor(TFT_WHITE);
tft.setTextSize(4);
tft.drawRect(0,0,239,134,TFT_RED);
tft.drawLine(1,46,238,46,TFT_RED);
tft.drawLine(1,88,238,88,TFT_RED);
tft.setCursor(24,8);
tft.print("00:00:00");
tft.setCursor(Spc[L_DOW],52);
tft.setTextColor(TFT_GREEN);
tft.print(Dow[L_DOW]);
tft.setCursor(30,100);
tft.setTextSize(3);
tft.setTextColor(TFT_YELLOW);
tft.print("04/");
L_Month = myRTC.getMonth(century);
PRINT_Month();
tft.print("/");
if (century) {
tft.print("2024");
}
else {
tft.print("2023");
}
tft.setTextSize(4);
}
void loop() {
SECS = myRTC.getSecond();
if (L_SECS != SECS) {
tft.setTextColor(TFT_BLACK);
PRINT_SECS();
L_SECS = SECS;
tft.setTextColor(TFT_WHITE);
PRINT_SECS();
}
MINS = myRTC.getMinute();
if (L_MINS != MINS) {
tft.setTextColor(TFT_BLACK);
PRINT_MINS();
L_MINS = MINS;
tft.setTextColor(TFT_WHITE);
PRINT_MINS();
}
HOURS = myRTC.getHour(h12Flag,pmFlag);
if (L_HOURS != HOURS) {
tft.setTextColor(TFT_BLACK);
PRINT_HOURS();
L_HOURS = HOURS;
tft.setTextColor(TFT_WHITE);
PRINT_HOURS();
}
DOW = myRTC.getDoW();
if (L_DOW != DOW) {
tft.setTextColor(TFT_BLACK);
PRINT_DOW();
L_DOW = DOW;
tft.setTextColor(TFT_GREEN);
PRINT_DOW();
}
Date = myRTC.getDate();
if (L_Date != Date) {
tft.setTextColor(TFT_BLACK);
tft.setTextSize(3);
PRINT_Date();
L_Date = Date;
tft.setTextColor(TFT_YELLOW);
PRINT_Date();
tft.setTextSize(4);
}
Months = myRTC.getMonth(century);
if (L_Month != Months) {
tft.setTextColor(TFT_BLACK);
tft.setTextSize(3);
PRINT_Month();
L_Month = Months;
tft.setTextColor(TFT_YELLOW);
PRINT_Month();
tft.setTextSize(4);
}
}
void PRINT_SECS() {
tft.setCursor(168,8);
if (L_SECS < 10) {
tft.print("0");
}
tft.print(L_SECS);
}
void PRINT_MINS() {
tft.setCursor(96,8);
if (L_MINS < 10) {
tft.print("0");
}
tft.print(L_MINS);
}
void PRINT_HOURS() {
tft.setCursor(24,8);
if (L_HOURS < 10) {
tft.print("0");
}
tft.print(L_HOURS);
}
void PRINT_DOW() {
tft.setCursor(Spc[L_DOW],52);
tft.print(Dow[L_DOW]);
}
void PRINT_Date() {
tft.setCursor(30,98);
if (L_Date < 10) {
tft.print("0");
}
tft.print(L_Date);
}
void PRINT_Month() {
tft.setCursor(84,100);
if (L_Month < 10) {
tft.print("0");
}
tft.print(L_Month);
}
License 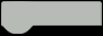
All Rights
Reserved
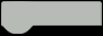
0
More from this category