
A chiming digital clock using an Arduino Nano.
This is a progression from a previous project but now using an ST7735S SPI 0.96in colour display. A DS3231 is used for timekeeping and the chimes are played from a DFPlayer Mini.
Resistors are used to drop the voltage to the display which requires 3.3v on the input pins.
Each chime sound starts 20 seconds before the hour so that the first bong is exactly on the hour.
Format a micro SD card using Fat32 and put the files from the chimes.zip onto it dragging them over one at a time, from the first to the last. This will ensure they are on the card in the correct physical order.
When you compile and flash the Arduino Nano, be sure to temporarily remove at least one of the serial connections to the dfplayer or the flash will fail.
/* **************************************************************************
* Digital Clock Project using ST7735 80 x 160 (RGB) .96 inch IPS Display
* With an Arduino Nano and a DS3231 RTC
*
* Pin Used With The Arduino Nano :
* A5 SCL RTC
* A4 SDA RTC
*
* D5 RST ST7735
* D6 CS
* D7 DC
* D13 SCL/SCK *These markings are confusing*
* D11 MOSI/SDA
*
**************************************************************************
*/
#include <Adafruit_GFX.h>
#include <Adafruit_ST7735.h>
#include <DFRobotDFPlayerMini.h>
#include <SPI.h>
#include <DS3231.h> //https://github.com/NorthernWidget/DS3231/releases
#include <Wire.h>
#define TFT_RST 5
#define TFT_CS 6
#define TFT_DC 7
Adafruit_ST7735 tft = Adafruit_ST7735(TFT_CS, TFT_DC, TFT_RST);
DFRobotDFPlayerMini myDFPlayer;
DS3231 myRTC;
// inverted color definitions
#define BLACK 0xFFFF
#define WHITE 0x0000
#define BLUE 0x07FF
#define RED 0xFFE0
#define GREEN 0xF81F
#define YELLOW 0xF800
#define BROWN 0x9F6D
#define L_BLUE 0x51E4
byte L_Hour = 18; // Last Hour
byte L_Min = 43; // Last Minute
byte L_Sec = 10; // Last Second
byte L_Dow = 6; // Last Day Of The Week
byte L_Date = 11; // Last Date
byte L_Month = 11; // Last Month
byte L_Temp = 28; // Last Temperature
byte L_Year; // Last Year
byte YEAR = 20; // Year 2000
byte YEAR_2; // Last 2 digits of the year
byte Sec,Min,HOUR,Hour2,Dow,Date,MONTH,Temp; // Variables from RTC
bool h12Flag,pmFlag; //Unused 12h pm Flags
bool century = false;
String DAYS_OF_WEEK [] {"Dummy","Monday","Tuesday","Wednesday","Thursday","Friday","Saturday","Sunday"};
void setup () {
Serial.begin(9600);
myDFPlayer.begin(Serial);
tft.initR (INITR_BLACKTAB);
Wire.begin();
tft.setRotation (3); // Display Lasdscape
tft.fillScreen (BLACK);
LinesDraw(); // Draw Lines Routine
MONTH = myRTC.getMonth(century);
if (century) { // Year 2100
YEAR = 21;
}
YEAR_2 = myRTC.getYear();
L_Year = YEAR_2;
TextDraw(); // Display All Screen Text
tft.setTextSize(3); // Time Text Size
}
void loop (){
Sec = myRTC.getSecond();
if (Sec != L_Sec) {
Sec_Print();
}
Min = myRTC.getMinute();
if (Min != L_Min) {
Min_Print();
}
HOUR = myRTC.getHour(h12Flag, pmFlag);
if (HOUR != L_Hour) {
Hour_Print();
}
Dow = myRTC.getDoW();
if (L_Dow != Dow) {
tft.setTextSize(2);
Dow_Print();
tft.setTextSize(3);
}
Date = myRTC.getDate();
if (L_Date != Date) {
tft.setTextSize(2);
Date_Print();
MONTH = myRTC.getMonth(century);
if (L_Month != MONTH) {
Month_Print();
YEAR_2 = myRTC.getYear();
if (YEAR_2 != L_Year) {
if (century) {
YEAR = 21;
}
Year_Print();
}
}
tft.setTextSize(3);
}
Temp = myRTC.getTemperature();
if (L_Temp != Temp) {
tft.setTextSize(2);
Temp_Print();
tft.setTextSize(3);
}
if ((Min == 59) && (Sec == 40)) {
Hour2 = HOUR +1;
chime();
}
}
void LinesDraw() { //Draw The Screen Borders
tft.drawLine(3,54,157,54,RED);
tft.drawLine(3,55,157,55,RED);
tft.drawLine(3,80,157,80,RED);
tft.drawLine(3,81,157,81,RED);
tft.drawLine(112,56,112,79,RED);
tft.drawLine(113,56,113,79,RED);
tft.drawRect(1,26,159,80,RED);
tft.drawRect(2,27,157,78,RED);
tft.fillRect(114,55,44,25,BROWN); // Temperature Box
}
void TextDraw() { //Set up Startup Screen
tft.setTextSize(3);
tft.setTextColor(WHITE);
tft.setCursor(10,30);
tft.print(L_Hour);
tft.print(":");
tft.print(L_Min);
tft.print(":");
tft.print(L_Sec);
tft.setTextSize(2);
tft.setTextColor(GREEN);
tft.setCursor(4 +((108-(DAYS_OF_WEEK [L_Dow].length())*12)/2),60);
tft.print(DAYS_OF_WEEK [L_Dow]);
tft.setTextColor(L_BLUE);
tft.setCursor(20,86);
tft.print("11/11/");
tft.print(YEAR);
tft.print(L_Year);
tft.setTextColor(YELLOW);
tft.setCursor(120,60);
tft.print("28c");
}
void Sec_Print () { // Print Seconds
tft.setTextColor(BLACK);
tft.setCursor(118,30);
if (L_Sec < 10) {
tft.print("0");
}
tft.print(L_Sec);
L_Sec = Sec;
tft.setTextColor(WHITE);
tft.setCursor(118,30);
if (L_Sec < 10) {
tft.print("0");
}
tft.print(L_Sec);
}
void Min_Print () { // Print Minutes
tft.setTextColor(BLACK);
tft.setCursor(64,30);
if (L_Min < 10) {
tft.print("0");
}
tft.print(L_Min);
L_Min = Min;
tft.setTextColor(WHITE);
tft.setCursor(64,30);
if (L_Min < 10) {
tft.print("0");
}
tft.print(L_Min);
}
void Hour_Print () { // Print Hours
tft.setTextColor(BLACK);
tft.setCursor(10,30);
if (L_Hour < 10) {
tft.print("0");
}
tft.print(L_Hour);
L_Hour = HOUR;
tft.setTextColor(WHITE);
tft.setCursor(10,30);
if (L_Hour < 10) {
tft.print("0");
}
tft.print(L_Hour);
}
void Dow_Print() { // Print Days Of The Week String
tft.setTextColor(BLACK);
tft.setCursor(4 +((108-(DAYS_OF_WEEK [L_Dow].length())*12)/2),60);
tft.print(DAYS_OF_WEEK [L_Dow]);
L_Dow = Dow;
tft.setTextColor(GREEN);
tft.setCursor(4 +((108-(DAYS_OF_WEEK [L_Dow].length())*12)/2),60);
tft.print(DAYS_OF_WEEK[L_Dow]);
}
void Date_Print() { // Print Date
tft.setTextColor(BLACK);
tft.setCursor(20,86);
if (L_Date < 10) {
tft.print("0");
}
tft.print(L_Date);
L_Date = Date;
tft.setTextColor(L_BLUE);
tft.setCursor(20,86);
if (L_Date < 10) {
tft.print("0");
}
tft.print(L_Date);
}
void Month_Print(){ // Print Month
// 56x 86y
tft.setTextColor(BLACK);
tft.setCursor(56,86);
if (L_Month < 10) {
tft.print("0");
}
tft.print(L_Month);
L_Month = MONTH;
tft.setTextColor(L_BLUE);
tft.setCursor(56,86);
if (L_Month < 10) {
tft.print("0");
}
tft.print(L_Month);
}
void Temp_Print() { // Print Temperature
tft.setTextColor(BROWN);
tft.setCursor(120,60);
if (L_Temp < 10) {
tft.print("0");
}
tft.print(L_Temp);
L_Temp = Temp;
tft.setTextColor(YELLOW);
tft.setCursor(120,60);
if (L_Temp < 10) {
tft.print("0");
}
tft.print(L_Temp);
}
void Year_Print() { // Print the Year
tft.setTextColor(BLACK);
tft.setCursor(91,86);
tft.print(YEAR);
tft.print(L_Year);
tft.setTextColor(L_BLUE);
tft.setCursor(91,86);
tft.print(YEAR);
L_Year = YEAR_2;
tft.print(L_Year);
}
void chime() {
myDFPlayer.volume(15);
switch (Hour2) {
case 6:
myDFPlayer.play(6);
break;
case 7:
myDFPlayer.play(7);
break;
case 8:
myDFPlayer.play(8);
break;
case 9:
myDFPlayer.play(9);
break;
case 10:
myDFPlayer.play(10);
break;
case 11:
myDFPlayer.play(11);
break;
case 12:
myDFPlayer.play(12);
break;
case 13:
myDFPlayer.play(1);
break;
case 14:
myDFPlayer.play(2);
break;
case 15:
myDFPlayer.play(3);
break;
case 16:
myDFPlayer.play(4);
break;
case 17:
myDFPlayer.play(5);
break;
case 18:
myDFPlayer.play(6);
break;
case 19:
myDFPlayer.play(7);
break;
case 20:
myDFPlayer.play(8);
break;
case 21:
myDFPlayer.play(9);
break;
case 22:
myDFPlayer.play(22);
break;
case 23:
myDFPlayer.play(23);
break;
case 24:
myDFPlayer.play(24);
break;
case 1:
myDFPlayer.play(13);
break;
case 2:
myDFPlayer.play(14);
break;
case 3:
myDFPlayer.play(15);
break;
case 4:
myDFPlayer.play(16);
break;
case 5:
myDFPlayer.play(17);
break;
}
}
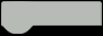