I. Introduction
When I first got my Unihiker, I noticed it not only could run Python but also had a touchscreen, WiFi module, and USB serial port. After a quick brainstorming, I came up with a magical combo - quickly implementing IoT remote control over a serial port robot!
Luckily I found my hexapod robot at the bottom of my bed where it's been collecting dust for years. It's a walking robot that mimics insect movement, with 6 independent legs each connected to a set of servos. Walking, turning, and other moves are achieved by coordinating these 6 sets of servos.
Since the Unihiker can receive serial data over USB, I can establish communication between the robot and Unihiker using a USB to TTL module. Of course, you could also connect any other actuators over the USB interface.

To achieve this idea, two key steps need to be addressed:
1. How to send data and control another device via USB serial port
2. How to enable wireless remote send data via WiFi using SIoT
In this tutorial, we try to solve the first step.
II. Hardware Connections
Notice:
- The tx and rx need to be crossed over, tx to rx and rx to tx (Rx--Receive pin Tx--Transmit pin)
- The ground wires of the UniHiker and servo driver need to be connected

III. Unihiker + USB Control Robot
After the hardware device is connected, we need to test and make sure it successfully connects to the robot and sends the corresponding action instructions to the robot.
1. Connect Unihiker to Computer
To quickly verify if the command array data works, first connect the UniHiker's type-c to a computer and try uploading a program via USB, using the default IP address 10.1.2.3.
2. Identify the Number of USB Serial Port on the UniHiker:
We can determine the serial number of the USB to TTL module on Unihiker by plugging and unplugging the module.
- Unplug the USB to TTL module and open the terminal on the UniHiker, enter the command 'ls /dev/tty*'
- Plug in the USB TTL module and open the terminal on the UniHiker, enter the command 'ls /dev/tty*'
- Compare the serial port listings between the two times, the added port is the USB serial port that needs to be controlled.

If a new USB serial port is not detected, you may need to install the USB driver. Search for 'how to install USB driver on arm64 Debian system', I used CH340. The Unihiker has been integrated so I do not need to install the driver separately.
3. Confirm the command for controlling the robot
Next, we need to clarify which instructions can drive the robot to perform relevant actions.
I checked the robot's documentation, and noticed that the direction settings use hex format commands as follows.
forward -- [0x55,0x55,0x05,0x06,0x15,0x01,0x00]
backward -- [0x55,0x55,0x05,0x06,0x16,0x01,0x00]
left -- [0x55,0x55,0x05,0x06,0x07,0x01,0x00]
right -- [0x55,0x55,0x05,0x06,0x08,0x01,0x00]
4. PySerial Library
At this time we can complete the communication between Unihiker and the robot through the USB to TTL module. And we have know what instructions need to be sent.
Now we need to write Python code to make Unihiker receive execution instructions and send serial port data. You need to use the PySerial library here.
Specifically, PySerial is a Python library that allows you to open serial ports in Python programs, set parameters like baudrate, data bits, stop bits, and parity, and send and receive data. It provides functions for reading and writing serial data, and supports synchronous and asynchronous operations, timeout settings and event callbacks.
The UniHiker board already has PySerial built-in, so we don't need to pip install it and can directly use it.
These functions are used:
'import serial'
'serial.Serial("/dev/ttyUSBport",baud rate)'
5. Code
Load Serial library
- Select the USB port "/dev/ttyUSBport" on Unihiker (as mentioned on III.2), the baud rate defaults to 9600
- Execute the command to go ‘forward’, 'backward', 'left', 'right'.
import serial
import time
ser = serial.Serial("/dev/ttyUSBport",9600)
forward = [0x55,0x55,0x05,0x06,0x15,0x01,0x00]
backward = [0x55,0x55,0x05,0x06,0x16,0x01,0x00]
left = [0x55,0x55,0x05,0x06,0x07,0x01,0x00]
right = [0x55,0x55,0x05,0x06,0x08,0x01,0x00]
ser.write(forward)
time.sleep(2)
ser.write(backward)
time.sleep(2)
ser.write(left)
time.sleep(2)
ser.write(right)
time.sleep(2)
With this code, we can control the robot's forward, backward, left, and right movements, and debug it by connecting it to the USB control. But this method always seems less elegant. I will continue to try the remote control method and share it with you next time.
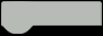