In the previous project, we accomplished controlling the robot's movement by sending data with USB. https://community.dfrobot.com/makelog-313506.html
Now we have completed the basic software and hardware collaboration testing, including hardware construction and software programming. But now it still needs to be connected through wire, and the functions of wireless debugging and IoT command sending and control have not been implemented.
To make the debugging process more advanced, I will now share how I implemented wireless control of the robot using SIoT. After running the program, we could quickly and easily control the movement of the robot by sending instructions directly on the webUI.
Key Steps:
Connect the Unihiker and computer to the same WiFi, then burn the code to the Unihiker.
In Python code, set the access account and password for SIoT, and set the 'topic' format in ‘xxx/xxxxx’.
In the browser, enter the IP of the Unihiker, and it will automatically jump to the SIoT WebUI interface.
In the interface, open the 'topic' control and send corresponding commands, then you can control the robot through SIoT.
1. MQTT and Python Implement the IoT
Next, we use Python programming first to implement IoT command-sending control with SIoT. Unihiker has a built-in SIoT server that allows us to send instructions through the webUI. Unihiker's IoT functions are mainly implemented through the MQTT communication protocol. After uploading the Python program containing SIoT settings, we can access the SIoT project page through the network and directly send commands to the IoT to control the robot.

What is MQTT ?
MQTT ( Message Queuing Telemetry Transport) is a lightweight protocol for communication between IoT devices. It uses a publish/subscribe model where devices can publish messages to a topic. Other devices can subscribe to the topic to receive the messages. This allows different devices to exchange information.
In summary, it's like a mail system for connected devices to send and receive messages. MQTT makes it easy for sensors, mobile devices, and embedded systems to talk to each other.
2. Configure Unihiker's Wifi
The first step to implementing IoT is to connect the Unihiker to the same Wi-Fi network with the computer.

Connect the UNIHIKER to the Wi-Fi:
Plug the Unihiker USB to the computer. Enter the IP address ‘10.1.2.3’ in the browser. Click on Network Settings and enter the Wi-Fi to connect (note it needs to be 2.4G Wi-Fi). Then connect the computer to the same hotspot and enter the board's IP to start programming.

(More tutorials to connect the UNIHIKER: https://www.unihiker.com/wiki/connection)
The remote control of the UNIHIKER is now configured!
3. Python + SIoT Setting to Unihiker
We need to use a server named SIoT to implement IoT.
SIoT is a free MQTT server software that can create local IoT servers easily.
The Unihiker has built-in SIoT server and is enabled by default.
Next, we need to write Python code to subscribe to SIoT and implement MQTT communication for IoT. Let's first try the basic subscription to SIoT and receive messages.
Run the following code separately and send a message on the platform, you will notice the output information in the terminal.
import siot
# event callback function
def on_message_callback(client, userdata, msg):
val = msg.payload.decode()
print(val)
# siot initialization
siot.init(client_id="",server="127.0.0.1",port=1883,user="siot",password="dfrobot")
siot.connect()
siot.loop()
siot.set_callback(on_message_callback)
siot.getsubscribe(topic="ProjectID/DeviceName")
siot.publish(topic="ProjectID/DeviceName", data="hello")
Key function explanations:
1. The callback function 'on_message_callback(client, userdata, msg):' is a SIoT MQTT message callback that handles subscribed MQTT messages when received.
- Receives subscribed MQTT topic messages
- Extracts and decodes the message content
- Passes the message content to the contr() function for processing.
2. Log in to the account and select the IP: siot.init(client_id="",server="127.0.0.1",port=1883,user="siot",password="dfrobot")
- The port 1883 refers to the mqtt communication port of the siot server
- The IP address, user, and password are default and do not need to be modified.
In the siot.init() function, 127.0.0.1 and 1883 indicate:
- ip=127.0.0.1: Connecting to the MQTT broker running locally
- port=1883: Using unencrypted communication
3. Connect to SIoT: siot.connect()
4. The method for the MQTT client. Sets the function to be called when a message arrives:
siot.set_callback(on_message_callback)
5. Subscribe to the SIoT topic (the topic name needs to use the xx/xxx format):
siot.getsubscribe(topic="ProjectID/DeviceName")
6. Sending data to verify the connection and subscription is successful:
siot.publish(topic="ProjectID/DeviceName", data="hello")
7. If the topic does not exist yet, it will be automatically created after uploading the code, do not need extra manual operation is needed.
Running the above code, the terminal receives the server connection succeeded message. At the same time, it receives ‘hello’, which verifies the topic is successfully subscribed and proves Python has established bidirectional communication with the server.

Next, let's try sending a message on the SIoT web.
4. SIoT WebUI Control Setting
After completing the Python code to subscribe to SIoT, we have finished setting up the topic on the SIoT server. We just need to access the Unihiker IP address in the Chrome browser to enter the SIoT control interface, where we can wirelessly send information to control the robot.
Enter wireless IP, 'start', then 'open page'

Login to SIoT, select the topic name from the uploaded program, and send data in the 'send message'
Send content in 'send message', and receive the message in the terminal, which proves SIoT web has established communication with Python.

5. Code in Remote Control
Now we can directly control the robot's movement by sending action messages on the SIoT webUI. In this control, I send 'w', 's', 'a', 'd' to control the robot's movement, to simulate the keyboard control of forward, backward, left and right in a game. Of course you can also set other commands to control the movement.
import time
import serial
def act(key):
if key == "w": #go forward
buf = [0x55,0x55,0x05,0x06,0x15,0x01,0x00]
ser.write(buf)
if key == 's': #go backward
buf = [0x55,0x55,0x05,0x06,0x16,0x01,0x00]
ser.write(buf)
if key == 'a': #left
buf = [0x55,0x55,0x05,0x06,0x07,0x01,0x00]
ser.write(buf)
if key == 'd': #right
buf = [0x55,0x55,0x05,0x06,0x08,0x01,0x00]
ser.write(buf)
ser=serial.Serial("/dev/ttyUSB0",9600)
import siot
# event callback function
def on_message_callback(client, userdata, msg):
val = msg.payload.decode()
act(val)
# siot initialization
siot.init(client_id="",server="127.0.0.1",port=1883,user="siot",password="dfrobot")
siot.connect()
siot.loop()
siot.set_callback(on_message_callback)
siot.getsubscribe(topic="ProjectID/DeviceName")
siot.publish(topic="ProjectID/DeviceName", data="hello")
while True:
time.sleep(1)

We can also open the SIoT page by entering the IP address in the mobile browser and directly for control.
Now we can remotely control the robot with IoT.
Overall, remotely controlling hexapod robots through USB serial communication and SIoT is a challenging project. In this process, we implemented USB serial communication and utilized SIoT to achieve remote control of devices within the LAN. Although the hexapod robot is one of the case studies, this control method combining serial communication and IoT technology can be applied to many other hardware devices.
I hope this project can provide some inspiration and allow us to see the application potential of integrating software, hardware, and internet technology in intelligent device control.
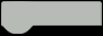