
Welcome to the world of DIY smart homes!
In this project, we'll be harnessing the power of the new Unihiker Single Board Computer (SBC) to create a smart home setup. Imagine controlling lights and monitoring room temperature with just a few clicks - that's the essence of what we're building.
Using Unihiker as our brains, we'll connect three buttons to control three LEDs via Nodemcu using MQTT - a nifty communication protocol. Plus, we'll toss in a DHT11 sensor to keep tabs on the room temperature.
This project serves as a beginner-friendly gateway to the home automation. With straightforward steps and minimal components, you'll be amazed at the magic you can create within your living space. Let's dive in and turn your home a tad smarter!
Prerequisites:
Visual Studio Code installed on your computer.OpenSSH installed on both your computer and the Unihiker.
Steps:
Install the Remote - SSH Extension:
Open Visual Studio Code.Go to the Extensions view by clicking on the square icon on the left sidebar (or press Ctrl+Shift+X).
Search for "Remote - SSH" extension and install it.

Open Remote-SSH Extension:

In Visual Studio Code, press F1 or Ctrl+Shift+P to open the command palette.
Type and select Remote-SSH: Connect to Host....
Enter the Unihiker SSH connection string: [email protected].
It'll prompt you to add configure file, then OS-type which is Linux and then Password which is dfrobot by default.
Connect to Unihiker:Visual Studio Code will open a new window connecting to your Unihiker via SSH.
It may prompt for your SSH key passphrase which is dfrobot by default.
Once connected, you'll be working within VS Code but with direct access to your Unihiker's filesystem and terminal.
Start Coding:
You can now edit files, run commands, and work on your Unihiker project within Visual Studio Code just as if you were working directly on the device.
So There are few main libraries that I'm mentioning below.
NodeMcu:
(PubSubClient.h), This library is of course for the MQTT protocol at the NodeMcu end.
(DHT.h), This library for the DHT11 sensor.
And some other necessary standard libraries for Nodemcu.
Installation:
Just navigate to the Library manager and search for the DHT sensor library by the DFrobot and install it.
And do the same for PubSubClient.
Python(Unihiker):
(PyQT5), This is the GUI library for python. You can use the default GUI library for UNihiker. I used this one because I'm more comfortable with it.
(paho.mqtt.client), This is the MQTT library for the python.
And some other necessary library for unihiker like the pinpong library which is for accessing GPIO pins and the on-board sensors. For Example, I used On-board light sensor to capture ambient light and update the text as per defined instructions. This is just for testing purpose and has really nothing to do with our main code but you can assign special tasks to it.
Installation:
Just Open the terminal and type followings to install these libraries.
Pyqt5 Library
pip install PyQt5
Paho Mqtt
pip install paho-mqtt
And you are good to go now.
Instructions for MQTT Configuration in Python:
MQTT Broker Address:
Set the MQTT_ADDRESS variable to the IP address (or hostname) of your MQTT broker where messages will be sent and received.
MQTT Credentials:
Enter the MQTT username and password in MQTT_USER and MQTT_PASSWORD variables respectively. Ensure these credentials provide secure access to your MQTT broker. Consider using strong and unique passwords for enhanced security.
Topic Configuration:
MQTT_TOPIC_test:
This topic is designated for Python's subscription to receive data from an ESP device. Configure this topic to match the topic the ESP device is publishing data to.
topic:
Python will use this topic to publish data to the ESP device. Ensure it aligns with the topic the ESP is subscribed to for receiving Python's messages.
Instructions for NodeMCU MQTT Configuration:
MQTT Broker Configuration:
mqtt_server:
Set this variable to the IP address (or hostname) of the MQTT broker you are connecting the NodeMCU to.
Topic Configuration:
one_topic:
This is the MQTT topic to which the NodeMCU will publish data. Ensure it matches the topic the Python side is subscribed to for receiving messages.
two_topic:
NodeMCU will subscribe to this topic to receive data from the Python side. Verify that this topic aligns with the one Python uses for publishing messages.
MQTT Credentials:
mqtt_username and mqtt_password:
Enter the MQTT username and password that match the credentials set in your MQTT broker. Ensure they provide appropriate access rights for communication.
Client ID:clientID:
This is the unique client ID assigned to the NodeMCU for MQTT communication. Ensure it's distinct and identifiable within your MQTT broker environment.

The Python code combines MQTT functionality and PyQt5 to create a smart home control interface. Key elements include:
MQTT Connection:
Establishes connection to an MQTT broker with credentials and subscribes to a specific topic for incoming messages.
GUI Elements:
Utilizes PyQt5 to create a window with labeled buttons for controlling lights and a fan.
Loads different images (ON/OFF states) for buttons.
Displays temperature readings using an LCD widget.
Button Interactions:
Each button's click toggles its state and sends corresponding MQTT messages to control devices (e.g., bulbs, fan) connected to an ESP8266.
Light Sensing:
Reads ambient light intensity and updates a label to indicate "Day" or "Night".
Integration:
Integrates MQTT client functions with PyQt5's event handling for seamless interaction between MQTT messages and the graphical interface.

This code configures an ESP8266-based NodeMCU to interact with an MQTT broker for smart home device control. Key elements include:
Wi-Fi Setup:
Connects the NodeMCU to a Wi-Fi network using specified SSID and password.
MQTT Configuration:
Sets MQTT server details (broker address), topics for publishing and subscribing, and MQTT credentials (username and password).
Sensor and Output Handling:
Integrates a DHT sensor for temperature and humidity readings (DHT11).
Defines GPIO pins to control outputs (lights and a fan).
MQTT Connection and Communication:
Establishes a connection to the MQTT broker and subscribes to a specific topic to receive messages.
Periodically publishes temperature readings to a designated topic.
Responds to incoming MQTT messages, controlling outputs (lights and fan) based on specific commands received.
The code enables the NodeMCU to serve as an MQTT client, facilitating communication between the Python-based control interface and physical devices, allowing users to remotely control and monitor home appliances.

DHT11 Sensor Wiring:
DHTPIN:
Defined as DHTPIN D2 in the code, which means the DHT sensor is connected to digital pin D2 on the NodeMCU.
Connect the DHT11 sensor's VCC pin to the NodeMCU's 5V pin.Connect the DHT11 sensor's GND pin to the NodeMCU's GND pin.Connect the DHT11 sensor's DATA pin to digital pin D2 on the NodeMCU.Output Devices (LEDs in my case):
Defined output pins:
int Out1 = D4; int Out2 = D5; int Out3 = D1;.
Connect the positive (anode) terminals of the LEDs to these output pins (D4, D5, D1).Connect the negative (cathode) terminals of the LEDs or the fan to the NodeMCU's GND pin.And if you are using a 3v cell then Connect it's Positive terminal to the Vin or 5v line, and negative to GND terminal.
Download all required files from the Github Link.
First Program the Nodemcu by opening the Arduino code file. Change the credentials as per you want.
And hit Upload.
Now as for the Unihiker side. Copy the files into the root directory. You can do this either by using the Local Web page of Unihiker at 10.1.2.3 (http://10.1.2.3/pc/file-upload)
You can open the python main file and make changes to credentials.
After that you can directly run it from the VScode terminal or by selecting it from the Unihiker GUI.
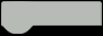