Introducing "SunByte, " my Unihiker SBC project. No specific reason for the name, just felt right. Got this board months ago, captivated by its features. It became my go-to for creative projects.
My previous project was Smart home using this board. AndI really am in love with that. Next, I decided to make Digital clock with it. My aims were to make it look both aesthetic and functional.
So here we are. My first problem was to find a better GUI framework. For my previous projects I used PYQT. That's a good framework too, but I felt entraped in it and I decided to look for a new and better framework. And that's when I found the Kivy Framework for python. Its ease of understanding, smooth performance, and rich customization options provided the perfect solution for my clock project.
So in this "How to", I'll go through the process of installation of Kivy and every other step needed for this project.
Kivy is an open source framework and is reliable solution for developing cross platform applications. And has a smooth performance.
There are two methods to install kivy on unihiker. The first one is PPA and the second is by using pip. Later one works smoothly without any hinderance. While the first one isn't a friendly option.
First of all we have to be logged into the Unihiker via SSH on VS code. You can follow unihiker wiki guide or my previous project DIY Smart Home using Unihiker.
Next in root shell, or command line terminal run the following command.
pip install kivy[base] kivy_examples --pre --extra-index-url https://kivy.org/downloads/simple/
and it'll take some time to completely install it. Make sure you stay connected to the internet all the time.

Once the installation is complete you can run the following command,
sudo apt-get update
And again, it'll take some time to update the list. Once it is finished you can check that wether kivy got properly installed or not by importing it.
python
import kivy

If there is no error then you are good to go.
Okay now since we are making a digital clock, that means we will be dealing with timezones and Clock. And if you bought Unihiker and you are in different time zone, then if you run the eaxmple "Alarm Clock" you'll see that time is not correct. That's because it is set to default settings which is Chinese Time Zone, Shanghai City. You can see it by running the following command,
timedatectl
Now if you want to change the time zone simply go back to the terminal and run the following command. This following command will list the available timezones.
timedatectl list-timezones

And now use this following command to set your current time zone.
sudo timedatectl set-timezone
I'm living in Pakistan so my time zone is Asia/Karachi And the command looked like this,
sudo timedatectl set-timezone Asia/Karachi
And again by running following command we can see our newly set time zone.
timedatectl

That's it. Now we can move further.
Before steping into code, we need to get our Weather API.
API stands for Application Programming Interface. APIs are simple request and response method to communicate between two different applications. Different applications comes with their specific APIs, by utilizng them developers can interate those applications into a new application. Take API as a Waiter in a resturaunt, customer comes in and give order to the waiter. The waiter takes the order and goes back in the kitchen to the chef and tell him the order placed by the customer. Cheif or cook prepares the order and waiter takes it and serve it to the customer. Now APIs do the same thing as the waiter. They take the request by the developer or program and send it to the application. Which generate the response and serves it back. I guess this will be helpful example.
Anyway, here we have to follow few steps to get our API key.
First head to the https://home.openweathermap.org/users/sign_up
and signup for an account.

Once you are finished doing signup and loging in. Go to your API key tab and there you will see a generated API key. This API key is specific for each user and is our key to weather updates so don't share it with others. Anyway you can name it like I did Multan. This is optional step but useful for organizing multiple api keys.

This is the API key tab, where you can manage your keys
Note: API key will take some time for activation after generating it. After that you can start using it.
Now since we have our API key we can move forward to programming.
from kivy.app import App
from kivy.uix.label import Label
from kivy.uix.floatlayout import FloatLayout
from kivy.uix.image import Image
from kivy.clock import Clock
import requests
from kivy.config import Config
from kivy.graphics import Color,Rectangle
from pinpong.board import *
from pinpong.extension.unihiker import *
from kivy.core.window import Window
Most of these libraries are for kivy and gets installed with installation of kivy. Two libraries are for unihiker board itself.
One library is the system library and is ususally available to use but if still somehow it isn't you can install it with the folowing command,
pip install requests
And this is it. We have everything setup.
Now before explaining other part of code let me first tell the important part here, and that is the url structure for api.
api_key = "I'm your api key you can copy me from website profile"
This is your api key variable. You have to replace it's content with the API key that we generated in Weather API step.
city_name = 'Multan'
This is your city name, where you are living right now. I'm living in Multan.
url = f'https://api.openweathermap.org/data/2.5/weather?q={city_name}&appid={api_key}&units=metric'
And this is the URL structure, which will be being used by python to get response from. It has following parts:
Part A:
https://api.openweathermap.org/data/2.5/weather?
This is the main address or url of the page. And from this page we will be requesting our data.
Part B:
q={city_name}&appid={api_key}
And this part contains our city name. The place of which, we are requesting weather updates.
And the API key which stores our account information.
This part is critical part. Because a slight change in it will result in an error.
Part C:
units=metric
And the last part is the Unit part.
As you now there are two main types of units, i.e, Metric (°C, m/s) and Imperial (°F, mph). Americans use the Imperial system while we here in Asia use this Metric system. So by changing this line you can set your units to either option.
Okay so now we know what our URL means, we can move further.
Download it from here Source Code on Github
Fill structure is pretty straight forward. There's a main Digital_Clock.py file
and then there are folders for Icons, Backgound images and custom fonts.
And none of these belongs to me except the source code.
You can download them online.
You Can either directly clone the Github repo from the link down below into unihiker or you can download the the files and copy paste them manually into your root/ folder via VS Code.

_R3JQ5qc2Sw.png?auto=compress%2Cformat&w=740&h=555&fit=max)
This is the following code go through.
Initialization and SetupImport necessary modules and libraries such as Kivy components, requests, and others.Initialize the UNIHIKER board.
Building the GUI
Define the DigitalClock class inheriting from App.Create a layout using FloatLayout and set a background image with a label displaying "HACKHOBBY LAB".Add labels for time, date, city, temperature, humidity, and weather description. Also, include icons for temperature and humidity.Schedule functions for updating time, weather, and adjusting screen orientation and brightness.Time and Date Update
Implement functions (get_time and get_date) to fetch and display the current time and date.Use Kivy's Clock to periodically update the time and date.
Weather Information
Fetch weather information from OpenWeatherMap using the provided API key and city name.Parse the JSON response and update labels with temperature, humidity, and weather description.Dynamically position and display a weather icon based on weather conditions.
Dynamic Icon Positioning
Implement a function to calculate the X-position for the weather icon based on the length of the weather description text.Adjust the icon's position on the screen accordingly.Load and display the weather icon using Kivy's Image widget.
Screen Orientation and Brightness Control
Implement functions to adjust screen orientation based on accelerometer readings.Control screen brightness using button inputs (button_a and button_b) with the help of the os module.
Application Execution
Start the Kivy application by instantiating the DigitalClock class and calling its run method.That's it. This is our main structure and parts of program.
Now we Can Run it and see our the fruit of our hardwork.
.



Here are few things that I need to explain.
Firstly, This API key for Open Weather Map has limitations as this is the free plan.The number of calls are limited. It's around 60 calls per minute I guess. But that doesn't mean anything bad. Because we are not going to call for it more than that.
The Dynamic Icon update method is bit crude. I couldn't find anything good that's why I decided to use this method to update the weather icon position based on the length of the word. (Smoke, Haze, Clear Sky or Snow, etc ).
Other then that, I'm somewhat satisfied with this setup and will also add more features like auto updating the city and timezone. But for me it'll take time. But if you are interested then you can send a Pull Request on Github.
So that's it for now, Thanks :)
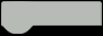