
How to Make an Arduino Obstacle Avoiding Car With Mecanum Wheel

Greetings everyone, and welcome to my article tutorial. Today, I'll guide you through the process of creating an Arduino Obstacle Avoiding Car with Mecanum Wheel.
Project Overview:
In this project, we will use an Ultrasonic Sensor to measure distances. Based on the detected distance, the car will take appropriate actions. For example, if an obstacle is detected within 20 cm, the car will change its path by performing an omnidirectional movement.
Without further ado, let's dive into the project and get started!




Below are the components required for making this Arduino Obstacle Avoiding Car with Mecanum Wheel:
International:
(Amazon)
• Arduino Uno: https://amzn.to/3zJpqrU
• L298D Motor Driver: https://amzn.to/3vA9dBO
• UltraSonic Sensor: https://amzn.to/3vA9dBO
• Gear Motor: https://amzn.to/3vA9dBO
• Mecanum Wheel: https://bit.ly/4bOr5P6
• Battery Holder: https://amzn.to/3vA9dBO
• Battery: (Get it in an old power bank)
India:
• Arduino Uno: https://bit.ly/3QQdbDN
• L298D Motor Driver: https://bit.ly/3cOLKX2
• UltraSonic Sensor: https://bit.ly/3cOLKX2
• Gear Motor: https://bit.ly/3cOLKX2
• Mecanum Wheel: https://bit.ly/3UPQQri
• Battery Holder: https://bit.ly/3cOLKX2
• Battery: https://bit.ly/3cOLKX2
Tools
• Soldering iron kit
• Wirecutter
• Glue gun



I used Tinkercad to plan and design my project. I designed this chassis with three things in mind: easy assembly, 3D printability, and affordability. After finalizing the design, I exported the file in STL format to ensure it was ready for 3D printing. The attached file includes all necessary components and dimensions for accurate printing and straightforward assembly. For even greater affordability, you can also consider CNC cutting the chassis frame from an acrylic sheet.




Follow the steps:
- Mount the Arduino uno and L298D Motor Driver Sheild to the chassis.- Connect the Gear Motor wire to the Motor Driver motor pin. (as shown in the circuit diagram)- Mount the Ultrasonic Sensor to the Chassis front part carefully using the hot glue.- Follow this Ultrasonic Sensor connection:

~ Connect the USB cable to the Arduino Uno.
~ Disconnect the Echo and Trig pins of the Ultrasonic Sensor to ensure the code uploads successfully.
CODE:
GitHub: ShahbazCoder1/Arduino-Obstacle-Avoiding-Car-With-Mecanum-Wheel
//Arduino Obstacle Avoiding Car With Mecanum Wheel
//Created By roboattic Lab
//Please Subscribe and Support us - https://youtube.com/@roboatticLab
//Contact me here https://www.instagram.com/roboattic_lab/
//You need to include AF Motor.h library before uploading the sketch, otherwise you'll get compilation error message.
#include <AFMotor.h> // Include the AFMotor library for motor control
AF_DCMotor motor1(1, MOTOR12_1KHZ);
AF_DCMotor motor2(2, MOTOR12_1KHZ);
AF_DCMotor motor3(3, MOTOR34_1KHZ);
AF_DCMotor motor4(4, MOTOR34_1KHZ);
// Define the ultrasonic sensor pins
const int trigPin = A1; // Change to a digital pin if needed
const int echoPin = A0; // Change to a digital pin if needed
void setup() {
Serial.begin(9600); // Start serial communication for debugging
// Set motor speed (adjust as needed)
pinMode(trigPin, OUTPUT);
pinMode(echoPin, INPUT);
motor1.setSpeed(150);
motor2.setSpeed(150);
motor3.setSpeed(150);
motor4.setSpeed(150);
randomSeed(analogRead(0)); // Initialize random seed
}
long duration;
int distance;
void loop() {
// Get the distance from the ultrasonic sensor
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
duration = pulseIn(echoPin, HIGH);
distance = (duration / 2) / 29.1;
// Print the distance to the serial monitor (for debugging)
Serial.print("Distance: ");
Serial.print(distance);
Serial.println(" cm");
// Obstacle avoidance logic
if (distance < 20) { // Adjust the threshold distance as needed
// Obstacle detected - Stop the car
stopCar();
// Back up for a short time
backUp(1000);
// Turn to the left or right randomly to avoid the obstacle
int randomTurn = random(0, 2);
if (randomTurn == 0) {
strafeLeft(1000); // Adjust strafing time as needed
} else {
strafeRight(1000);
}
// Move forward again
moveForward();
} else {
// No obstacle detected - Move forward
moveForward();
}
delay(50); // Delay between readings
}
// Function to move the car forward
void moveForward() {
motor1.setSpeed(150);
motor1.run(FORWARD);
motor2.setSpeed(150);
motor2.run(FORWARD);
motor3.setSpeed(150);
motor3.run(FORWARD);
motor4.setSpeed(150);
motor4.run(FORWARD);
}
// Function to stop the car
void stopCar() {
motor1.run(RELEASE);
motor2.run(RELEASE);
motor3.run(RELEASE);
motor4.run(RELEASE);
}
// Function to move the car backward
void backUp(unsigned long duration) {
motor1.setSpeed(150);
motor1.run(BACKWARD);
motor2.setSpeed(150);
motor2.run(BACKWARD);
motor3.setSpeed(150);
motor3.run(BACKWARD);
motor4.setSpeed(150);
motor4.run(BACKWARD);
delay(duration);
stopCar();
}
// Function to strafe the car left
void strafeLeft(unsigned long duration) {
motor1.setSpeed(150);
motor1.run(BACKWARD);
motor2.setSpeed(150);
motor2.run(FORWARD);
motor3.setSpeed(150);
motor3.run(BACKWARD);
motor4.setSpeed(150);
motor4.run(FORWARD);
delay(duration);
stopCar();
}
// Function to strafe the car right
void strafeRight(unsigned long duration) {
motor1.setSpeed(150);
motor1.run(FORWARD);
motor2.setSpeed(150);
motor2.run(BACKWARD);
motor3.setSpeed(150);
motor3.run(FORWARD);
motor4.setSpeed(150);
motor4.run(BACKWARD);
delay(duration);
stopCar();
}
Once the code has been uploaded, insert the battery into the Battery Holder to activate the project. A demonstration video of this project can be viewed here: Watch Now.
Thank you for your interest in this project. If you have any questions or suggestions for future projects, please leave a comment and I will do my best to assist you.
For business or promotional inquiries, please contact me via email at Email.
I will continue to update this article with new information. Don’t forget to follow me for updates on new projects and subscribe to my YouTube channel (YouTube: roboattic Lab) for more content. Thank you for your support.
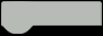