Welcome to an exciting adventure where we combine the power of Near Field Communication (NFC) tags, the versatility of the UNIHIKER device, and the mesmerizing glow of LEDs. In this tutorial, you will learn how to read NFC tag IDs using a mobile device and trigger REST API calls to a UNIHIKER board via WLAN.
The UNIHIKER will be running a Python Flask web service that controls an LED ring, changing its colors based on the API calls. By the end of this journey, you will have a working setup that beautifully integrates mobile NFC interactions with colorful LED displays.

Our journey begins with the assembly of our hardware. Follow these steps to mount the LED ring to the UNIHIKER board:
STEP 1 - Prepare the Components: Lay out the UNIHIKER board, the 12 LED RGB Ring, and the necessary cables.
STEP 2- Connect the LED Ring: Use the PH2.0-3P cable to connect the LED ring to the appropriate port on the UNIHIKER board (P24). Ensure that the connections are secure.
STEP 3 - Power Up: Connect the UNIHIKER board to a power source (USB). Once powered, the board should recognize the LED ring.

Create a new project directory named "NeoPixelAPI" on your local device. Inside the new directory "NeoPixelAPI" create the Python file named "main.py".
Content of "main.py":
from pinpong.board import Board, Pin, NeoPixel
from flask import Flask, jsonify
COLORS: dict = {
'red': (255, 0, 0),
'green': (0, 255, 0),
'blue': (0, 0, 255),
'black': (0, 0, 0),
'white': (255, 255, 255)
}
app = Flask(__name__)
@app.route(rule='/api/colors', methods=['GET'])
def get_available_colors():
"""
This method is an API route that returns the available colors. The values are from the COLORS dictionary.
:return: A JSON object containing the status and the available colors.
"""
return jsonify({'status': 'success',
'message': COLORS}), 200
@app.route(rule='/api/<string:color>', methods=['GET'])
def set_color(color):
"""
This method is an API route that sets the color of an LED strip. It takes in a color parameter as a string and
returns a JSON response indicating the status and message. The color parameter is used to set the color of each
LED in the strip. It is expected to be a valid color value that matches one of the predefined colors in the COLORS
dictionary. If the provided color is valid, the method iterates through each LED in the strip and sets the color to
the corresponding value from the COLORS dictionary.
The method returns a success response with a status code of 200 and a message indicating the color that was set if
the provided color is valid. If the provided color is not valid, the method returns an error response with a
status code of 400 and a message indicating that the color is not valid.
:param color: The color value to set for the LED strip.
:return: Response indicating the status and message.
"""
global led
api_color = str(color.lower())
if api_color in COLORS:
for pixel in range(led.num):
led[pixel] = COLORS[api_color]
return jsonify({'status': 'success',
'message': f"{color} is valid."}), 200
else:
return jsonify({'status': 'error',
'message': f"{color} is not valid."}), 400
if __name__ == '__main__':
Board().begin()
try:
led = NeoPixel(Pin(Pin.P24), 12)
led.clear()
except Exception as err:
print(err)
app.run(host='0.0.0.0')
I will show the usage of SCP (to upload project) and SSH (to run the REST API service). This requires that you are connected from the local computer to UNIHIKER at least via WLAN and/or USB.
```bash
# upload script with SCP
scp -r ~/NeoPixelAPI [email protected]:/root/NeoPixelAPI
# login with SSH
ssh -C4 [email protected]
# change directory
cd ~/NeoPixelAPI
```
Now start the Python Flask Web API Server. Follow the terminal output to observe successful start.
```bash
# run simple Flask server
python3 main.py
___________________________
| |
| PinPong v0.5.1 |
| Designed by DFRobot |
|___________________________|
[01] Python3.7.3 Linux-4.4.143-67-rockchip-g01bbbc5d1312-aarch64-with-debian-10.13 Board: UNIHIKER
selected -> board: UNIHIKER serial: /dev/ttyS3
[10] Opening /dev/ttyS3
[32] Firmata ID: 3.7
[22] Arduino compatible device found and connected to /dev/ttyS3
[40] Retrieving analog map...
[42] Auto-discovery complete. Found 30 Digital Pins and 30 Analog Pins
------------------------------
All right. PinPong go...
------------------------------
* Serving Flask app 'main' (lazy loading)
* Environment: production
WARNING: This is a development server. Do not use it in a production deployment.
Use a production WSGI server instead.
* Debug mode: off
* Running on all addresses.
WARNING: This is a development server. Do not use it in a production deployment.
* Running on http://10.0.0.14:5000/ (Press CTRL+C to quit)
```
In my example you can see “Running on http://10.0.0.14:5000/” . This is the WLAN IP for UNIHIKER in my home environment. For you this can be different! You can test the API inside your local WLAN:
### Example usage:
```
GET http://[IP address]/api/colors
Response:
{
"message":{
"black":[0,0,0],
"blue":[0,0,255],
"green":[0,255,0],
"red":[255,0,0],
"white":[255,255,255]
},
"status":"success"
}
GET http://[IP address]/api/red
Response:
{
"status": "success",
"message": "red is valid."
}
GET http://[IP address]/api/black
Response:
{
"status": "success",
"message": "black is valid."
}
GET http://[IP address]/api/invalid_color
Response:
{
"status": "error",
"message": "invalid_color is not valid."
}
```
If you have successfully completed the tests and have a modern mobile phone, you can use it to read the NFC tags and trigger actions. When the NFC tag is scanned, the mobile send the configured HTTP GET request to the UNIHIKER's Flask web service, triggering the LED color change. On IPhone you can make use of Application: Shortcuts.

After all NFC tags get scanned and specific actions are defined. You can start to control the LEDs via your Mobile.

Congratulations! You have successfully created an interactive setup where NFC tag scans trigger LED color changes via REST API calls to a UNIHIKER device. This project demonstrates the powerful combination of hardware and software, offering endless possibilities for further customization and expansion.
Enjoy UNIHIKER exploring and enhancing your creation!
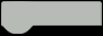