In this Python tutorial, I will guide you step-by-step to create a Fingerprint and Speech Access Control System using the DFRobot Unihiker, DFRobot Gravity: Text to Speech Voice Synthesizer Module V2.0, and DFRobot Gravity: Capacitive Fingerprint Sensor.
Objectives
1. Add new fingerprints to the system.
2. Verify fingerprints with a speech response.
3. Delete all fingerprints from the system.
By the end of this tutorial, you'll have a working system that can manage up to 80 fingerprints and provide audible feedback.
1. DFRobot Unihiker: A compact development board with a built-in screen and Wi-Fi/Bluetooth support.
2. DFRobot Gravity: Text to Speech Voice Synthesizer Module V2.0: A module that converts text to speech in English or Chinese.
3. DFRobot Gravity: Capacitive Fingerprint Sensor (I2C): A high-quality fingerprint sensor that can store up to 80 fingerprints.

Ensure the Unihiker is Powered Off
Before connecting any devices, make sure the Unihiker is not powered on. Disconnect the USB cable if it is attached.
Connect the Fingerprint Sensor
Plug the I2C connector of the fingerprint sensor into one of the two I2C ports on the Unihiker. Both interfaces work identically, so you can choose either.
Connect the Text to Speech Module
Use the provided wires to connect the voice synthesizer module to one of the I2C ports on the Unihiker. Like the fingerprint sensor, either I2C interface can be used.

Power Up the System
Once all connections are secure, connect the USB cable to power on the Unihiker. The Unihiker starts automatically when the USB cable is connected.
Organize your files into a project folder named: AccessControlSystem. Inside this folder, create three Python script files:
1. add_fingerprints.py: To add new fingerprints to the sensor memory.
2. main.py: To verify fingerprints and provide speech feedback.
3. delete_fingerprints.py: To delete all stored fingerprints from sensor memory.
If you are done the project structure should look like this:
# list folder and files (optional)
$ tree AccessControlSystem/
AccessControlSystem/
|-- add_fingerprints.py
|-- delete_fingerprints.py
`-- main.py
Here is an overview of the Python scripts. Each script serves a specific purpose in the system.
add_fingerprints.py
This script allows you to enroll new fingerprints into the sensor memory.
from pinpong.board import Board
from pinpong.libs.dfrobot_id809 import ID809
from time import sleep
MAX_FINGERPRINTS: int = 80
if __name__ == '__main__':
Board("UNIHIKER").begin()
sensor = ID809()
while not sensor.connected():
print("[INFO] Sensor initializing...")
sleep(1)
while True:
empty_id = sensor.get_empty_id()
if empty_id > MAX_FINGERPRINTS:
print("[ERROR] Maximum number of fingerprints reached")
break
print(f"[INFO] Use empty ID: {empty_id} to enroll a new fingerprint")
if sensor.collection_fingerprint(10) != sensor.error:
print('[INFO] Finger detected, please remove it now')
while sensor.detect_finger():
print('[INFO] Finger still detected, please remove it')
sleep(1)
if sensor.store_fingerprint(empty_id) != sensor.error:
print('[INFO] Finger stored')
sleep(1)
else:
print('[ERROR] Finger storage failed, please try again')
main.py
This script verifies fingerprints against the sensor's memory. If the fingerprint is recognized, the system will provide audio feedback using the text-to-speech module.
from signal import signal, SIGINT
from pinpong.board import Board
from pinpong.libs.dfrobot_speech_synthesis import DFRobot_SpeechSynthesis_I2C
from pinpong.libs.dfrobot_id809 import ID809
from time import sleep
from types import FrameType
from typing import Optional
VOICE_LEVEL: int = 5
VOICE_SPEED: int = 8
VOICE_TONE: int = 6
SECURITY_LEVEL: int = 4
def signal_handler(sig: int, frame: Optional[FrameType]) -> None:
"""
Handles the received signal, logs it, and raises a KeyboardInterrupt to
terminate the execution.
:param sig: The signal number received by the handler.
:type sig: int
:param frame: The current stack frame at the point the signal was received.
:type frame: Optional[FrameType]
:return: None
"""
_ = frame
print(f'[INFO] Signal handler: {sig} triggered. Exiting...')
raise KeyboardInterrupt
if __name__ == '__main__':
Board("UNIHIKER").begin()
speaker = DFRobot_SpeechSynthesis_I2C()
speaker.begin(speaker.V2)
speaker.set_voice(VOICE_LEVEL)
speaker.set_speed(VOICE_SPEED)
speaker.set_tone(VOICE_TONE)
speaker.set_english_pron(speaker.WORD)
sensor = ID809()
while not sensor.connected():
print("[INFO] Sensor initializing...")
sleep(1)
sensor.set_security_level(securityLevel=SECURITY_LEVEL)
signal(SIGINT, signal_handler)
speaker.speak('Access system is ready')
print('[INFO] Access system is ready')
try:
while True:
sensor.ctrl_led(mode=sensor.breathing, color=sensor.blue, blinkCount=0)
if sensor.collection_fingerprint(10) != sensor.error:
sensor.ctrl_led(mode=sensor.breathing, color=sensor.yellow, blinkCount=0)
speaker.speak('Finger detected')
print('[INFO] Finger detected')
while sensor.detect_finger():
sensor.ctrl_led(mode=sensor.fast_blink, color=sensor.white, blinkCount=1)
speaker.speak('Please remove your finger')
sleep(1)
ret = sensor.search()
if ret != 0:
sensor.ctrl_led(mode=sensor.fast_blink, color=sensor.green, blinkCount=2)
speaker.speak('Access granted')
print('[INFO] Access granted')
else:
sensor.ctrl_led(mode=sensor.fast_blink, color=sensor.red, blinkCount=2)
speaker.speak('Access denied')
print('[INFO] Access denied')
sleep(1)
except KeyboardInterrupt:
print('[INFO] Keyboard interrupt detected.')
except Exception as err:
print(f'[ERROR] An unexpected error occurred: {err}')
finally:
print('[INFO] Stopping application...')
speaker.speak('Access system is shutting down')
sensor.ctrl_led(mode=sensor.fade_out, color=sensor.blue, blinkCount=0)
delete_fingerprints.py
This script clears all fingerprints from the sensor's memory.
from pinpong.board import Board
from pinpong.libs.dfrobot_id809 import ID809
from time import sleep
if __name__ == '__main__':
Board("UNIHIKER").begin()
sensor = ID809()
while not sensor.connected():
print("[INFO] Sensor initializing...")
sleep(1)
number = sensor.get_enroll_count()
if number > 0:
print(f"[INFO] Found {number} fingerprints")
print("[INFO] Deleting all fingerprints...")
sensor.del_fingerprint(sensor.DELALL)
else:
print("[INFO] No fingerprints found to delete")
To upload your project, including all Python files, you choose between different options (eq. FTP, SMB, SCP). The online documents will be very helpful.
Here the example for SCP (user: root - password: dfrobot):
# upload via SCP
$ scp -r AccessControlSystem/ [email protected]/root/
You can run all Python scripts also via Unihiker's touch display, but I recommend for add_fingerprints.py and delete_fingerprints.py to use the command line! Here the example (including the SSH connection).
Note: The credentials for SSH by default are user: root and password: dfrobot.
# connect via SSH
$ ssh [email protected]
# change directory
$ cd /root/AccessControlSystem/
# add new fingerprints
$ python3 /root/AccessControlSystem/add_fingerprints.py
# verify fingerprints
$ python3 /root/AccessControlSystem/main.py
# delete all fingerprints
python3 /root/AccessControlSystem/delete_fingerprints.py
This project offers endless opportunities to innovate and expand!
1. Data Logging: Record access attempts in a log file.
2. Enhanced Security: Add facial recognition or IRIS scan.
3. Send Messages: Send messages via E-Mail or SMS.
4. Connect more Devices: Add door locks or servo motors.
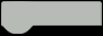