.Introduction:
This project is an application of artificial intelligence image recognition in the scenario of stray animal protection. The project uses the built-in offline cat/dog detection function of the Unihiker K10. In this way, the Automatic Cat Feeder can accurately identify and serve stray cats in need of help. When the Cat Feeder recognizes a cat and detects that the cat has approached the cat food bowl, it will automatically dispense food for feeding. Other animals entering the recognition range will not be feed.
2.Operation Instructions:
Gradually move the cat model (or a photo of a cat) into the recognition zone. The Cat Feeder will identify the cat, and the cat food dispenser will activate to release food. As the cat lowers its head to eat, the cat face recognition frame will vanish. The K10 initiates a 10-second countdown. Throughout this period, the machine will refrain from dispensing additional food to prevent the issue of repeated feeding that can occur when a cat repeatedly lowers and raises its head while eating. If an alternative animal model, such as a pheasant model, is introduced into the recognition area, the K10 will not recognize it, and the cat food dispenser will remain inactive.
3.Material Preparation:
4. Production Steps:
(Tip: Before beginning assembly, ensure that the 3D printed parts are pre-printed. The files for the printed parts are located at the end of the article.)






5. Code
/*!
* MindPlus
* esp32s3bit
*
*/
#include "unihiker_k10.h"
#include "AIRecognition.h"
// Dynamic variables
volatile float mind_n_time;
// Create an object
UNIHIKER_K10 k10;
AIRecognition ai;
uint8_t screen_dir=2;
// Main program start
void setup() {
k10.begin();
pinMode(P1, OUTPUT);
k10.initScreen(screen_dir);
ai.initAi();
k10.initBgCamerImage();
k10.setBgCamerImage(false);
k10.creatCanvas();
ai.switchAiMode(ai.NoMode);
mind_n_time = 0;
ai.switchAiMode(ai.Cat);
k10.setBgCamerImage(true);
}
void loop() {
if ((mind_n_time==5)) {
for (int index = 0; index < 5; index++) {
k10.canvas->canvasText((String("Waiting For ") + String((String(mind_n_time).toInt()))), 1, 0x0000FF);
mind_n_time -= 1;
delay(1000);
}
k10.canvas->canvasClear(1);
}
if ((mind_n_time==0)) {
if (ai.isDetectContent(AIRecognition::Cat)) {
if ((ai.getCatData(AIRecognition::Length)>180)) {
k10.canvas->canvasText("Feeding...", 1, 0x0000FF);
k10.canvas->updateCanvas();
digitalWrite(P1, HIGH);
delay(3000);
digitalWrite(P1, LOW);
while (!(100>ai.getCatData(AIRecognition::Length))) {}
mind_n_time = 5;
}
}
}
}
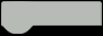