Make Your Own Arduino Powered 4-in-1 Robot Car | Step by Step instructions
Hello and welcome back! In this project, we will learn how to make a 4-in-1 2WD Robot Car using the Arduino UNO platform. This robot can be controlled in four ways, IR remote control, line tracking, Bluetooth control, and WiFi control. I used the Webotricks 2WD Robot Car Starter Kit, so we don’t need any additional chassis or extra components. You can get this kit at an affordable price using this link. Also, you can easily build and program this robot with the provided instructions. Even if you have no experience with robotics, don’t worry! This blog will guide you step by step, making everything simple to understand. Also this is a great way to learn about Arduino, sensors, motor control, and wireless communication.

Components and Supplies
2WD Car Chassis Kit - BUY NOW
L298N Motor Driver Module - BUY NOW
Infrared IR Remote Control Sensor Module - BUY NOW
Jumper-Wires - BUY NOW
USB Cable - Buy Now
Basic Board - Buy Now
18650 Battery Box - Buy Now
Battery charger for 18650 battery
HC Module - Buy Now
UART WI-FI Shield
Jumpers
Step 1
Start by assembling all the necessary components for your robot and ensure you have everything required for the build. unbox your robot package and check all the components inside the box.


Step 2
Second, remove the robot chassis sticker and attach the motor metal brackets to the motors. Then install them on the chassis.

Step 3
Thirdly, install the caster wheel onto the chassis.
Step 4
Now, attach the wheels to the gear motors.
Step 5
After that, install the Arduino UNO board and the motor driver board onto the robot chassis.
Step 6
Next, connect the motors to the motor driver board. After that, install the battery holder onto the chassis.

Step 7
Now, connect the WiFi Shield to the Arduino UNO board. Then, install the IR receiver module at the front of the robot.
Step 8
Afterward, install the tracking sensor modules onto the chassis.
Step 9
Okay, now connect the battery holder to the shield. Then, connect the motor driver board, IR receiver, and line tracking modules to the WiFi shield.
Motor Pins
Black — D9Purple — D12Green — D11Yellow — D7White — D8Red — D6IR Receiver
GND — GND5V — VCCD10 — SLeft Tracking Sensor
VCC — 5VGND — GNDDO — D2Right Tracking Sensor
VCC — 5VGND — GNDDO — D3
Step 10
Afterward, arrange the wires neatly using tape. Then, charge the 18650 Li-ion batteries and place them into the battery holder.
Step 11
Now, let’s upload the programs one by one to the robot car. Follow the instructions below for each step.
IR Control of the Robot Car
Connect your robot to the computer. Then, copy and paste the following code into the Arduino IDE. Also, make sure to include the IRremote library in the Arduino IDE before uploading the code.
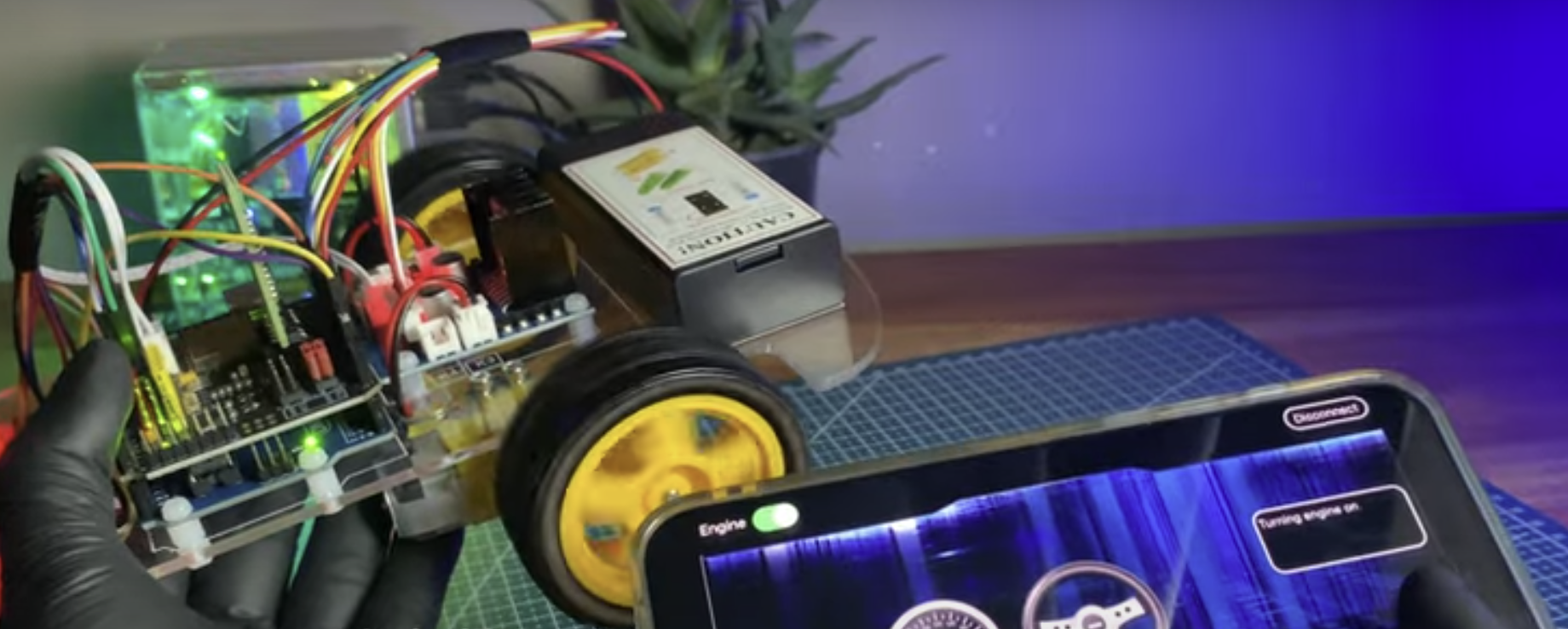
#include "IRremote.hpp"
#define IR_RECEIVE_PIN 10 //IR receiver Signal pin connect to Arduino pin D10
#define speedPinR 9 // RIGHT PWM pin connect MODEL-X ENA
#define RightMotorDirPin1 12 //Right Motor direction pin 1 to MODEL-X IN1
#define RightMotorDirPin2 11 //Right Motor direction pin 2 to MODEL-X IN2
#define speedPinL 6 // Left PWM pin connect MODEL-X ENB
#define LeftMotorDirPin1 7 //Left Motor direction pin 1 to MODEL-X IN3
#define LeftMotorDirPin2 8 //Left Motor direction pin 1 to MODEL-X IN4
#define IR_ADVANCE 24 //code from IR controller "▲" button
#define IR_BACK 82 //code from IR controller "▼" button
#define IR_RIGHT 90 //code from IR controller ">" button
#define IR_LEFT 8 //code from IR controller "<" button
#define IR_STOP 28 //code from IR controller "OK" button
#define IR_turnsmallleft 13 //code from IR controller "#" button
enum DN
{
GO_ADVANCE, //go forward
GO_LEFT, //left turn
GO_RIGHT,//right turn
GO_BACK,//backward
STOP_STOP,
DEF
}Drive_Num=DEF;
bool stopFlag = true;//set stop flag
bool JogFlag = false;
uint16_t JogTimeCnt = 0;
uint32_t JogTime=0;
uint8_t motor_update_flag = 0;
/***************motor control***************/
void go_Advance(void) //Forward
{
digitalWrite(RightMotorDirPin1, HIGH);
digitalWrite(RightMotorDirPin2,LOW);
digitalWrite(LeftMotorDirPin1,HIGH);
digitalWrite(LeftMotorDirPin2,LOW);
analogWrite(speedPinL,150);
analogWrite(speedPinR,150);
}
void go_Left(int t=0) //Turn left
{
digitalWrite(RightMotorDirPin1, HIGH);
digitalWrite(RightMotorDirPin2,LOW);
digitalWrite(LeftMotorDirPin1,LOW);
digitalWrite(LeftMotorDirPin2,HIGH);
analogWrite(speedPinL,0);
analogWrite(speedPinR,150);
delay(t);
}
void go_Right(int t=0) //Turn right
{
digitalWrite(RightMotorDirPin1, LOW);
digitalWrite(RightMotorDirPin2,HIGH);
digitalWrite(LeftMotorDirPin1,HIGH);
digitalWrite(LeftMotorDirPin2,LOW);
analogWrite(speedPinL,150);
analogWrite(speedPinR,0);
delay(t);
}
void go_Back(int t=0) //Reverse
{
digitalWrite(RightMotorDirPin1, LOW);
digitalWrite(RightMotorDirPin2,HIGH);
digitalWrite(LeftMotorDirPin1,LOW);
digitalWrite(LeftMotorDirPin2,HIGH);
analogWrite(speedPinL,150);
analogWrite(speedPinR,100);
delay(t);
}
void stop_Stop() //Stop
{
digitalWrite(RightMotorDirPin1, LOW);
digitalWrite(RightMotorDirPin2,LOW);
digitalWrite(LeftMotorDirPin1,LOW);
digitalWrite(LeftMotorDirPin2,LOW);
}
/**************detect IR code***************/
void do_IR_Tick()
{
if(IrReceiver.decode())
{
uint16_t command = IrReceiver.decodedIRData.command;
if(command==IR_ADVANCE)
{
Drive_Num=GO_ADVANCE;
}
else if(command==IR_RIGHT)
{
Drive_Num=GO_RIGHT;
}
else if(command==IR_LEFT)
{
Drive_Num=GO_LEFT;
}
else if(command==IR_BACK)
{
Drive_Num=GO_BACK;
}
else if(command==IR_STOP)
{
Drive_Num=STOP_STOP;
}
command = 0;
IrReceiver.resume();
}
}
/**************car control**************/
void do_Drive_Tick()
{
switch (Drive_Num)
{
case GO_ADVANCE:go_Advance();JogFlag = true;JogTimeCnt = 1;JogTime=millis();break;//if GO_ADVANCE code is detected, then go advance
case GO_LEFT: go_Left();JogFlag = true;JogTimeCnt = 1;JogTime=millis();break;//if GO_LEFT code is detected, then turn left
case GO_RIGHT: go_Right();JogFlag = true;JogTimeCnt = 1;JogTime=millis();break;//if GO_RIGHT code is detected, then turn right
case GO_BACK: go_Back();JogFlag = true;JogTimeCnt = 1;JogTime=millis();break;//if GO_BACK code is detected, then backward
case STOP_STOP: stop_Stop();JogTime = 0;break;//stop
default:break;
}
Drive_Num=DEF;
//keep current moving mode for 200 millis seconds
if(millis()-JogTime>=200)
{
JogTime=millis();
if(JogFlag == true)
{
stopFlag = false;
if(JogTimeCnt <= 0)
{
JogFlag = false; stopFlag = true;
}
JogTimeCnt--;
}
if(stopFlag == true)
{
JogTimeCnt=0;
stop_Stop();
}
}
}
void setup()
{
pinMode(RightMotorDirPin1, OUTPUT);
pinMode(RightMotorDirPin2, OUTPUT);
pinMode(speedPinL, OUTPUT);
pinMode(LeftMotorDirPin1, OUTPUT);
pinMode(LeftMotorDirPin2, OUTPUT);
pinMode(speedPinR, OUTPUT);
stop_Stop();
IrReceiver.begin(IR_RECEIVE_PIN, DISABLE_LED_FEEDBACK);
}
void loop()
{
do_IR_Tick();
do_Drive_Tick();
}
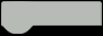