It is summer. It is hot. You are sweating in places you did not know could sweat. Let us build a DIY mini fan controller which cools you down a bit. With the Unihiker, an analog position slider (potentiometer) and the DC Fan Module, this small project can be realized in just a few minutes!
1. Turn off your Unihiker!
2. Connect the fan to Pin P22 on the Unihiker.
3. Connect the Potentiometer to Pin P21 on the Unihiker.
4. Connect the Unihiker via USB with your system (to power up).

from pinpong.board import Board, Pin, PWM
from time import sleep
POT_PIN: int = Pin.P21
FAN_PIN: int = Pin.P22
MIN_DUTY: int = 200
DELAY_SECONDS: float = 0.5
if __name__ == '__main__':
Board("UNIHIKER").begin()
potentiometer = Pin(POT_PIN, Pin.ANALOG)
fan = PWM(Pin(FAN_PIN))
try:
while True:
analog_value = potentiometer.read_analog()
fan_duty = int(analog_value * 1023 / 4095)
if fan_duty < MIN_DUTY: fan_duty = 0
fan.duty(fan_duty)
print(f"Analog: {analog_value}, Duty: {fan_duty}")
sleep(DELAY_SECONDS)
except Exception as err:
print(f"[ERROR] {err}")
finally:
fan.deinit()
So, what is going on in this code? Well, it all starts when you tell your Unihiker board, "Hey buddy, time to wake up and be smart." Next, the potentiometer (that slider you connected) is set up to read how far you have moved it. It gives you a number between 0 and 4095.
Then there is the fan. You do not just blast it at full speed (unless you are feeling dramatic). Instead, the fan is controlled using something called PWM. With a bit math the potentiometer position value will be converted into a number the fan understands — called duty cycle. The higher the number, the harder it blows.
While all this happens, the code keeps looping — reading the slider, updating the fan, and printing (to terminal) out what is going on.
Save the file with a cool name like windinator.py.
You can upload your windinator.py file to the Unihiker using several methods — whatever floats your data boat! Whether it is FTP, SMB, or SCP (for the terminal warriors), the Unihiker is flexible and ready.
Not sure how to do that? No worries — the exact steps can vary a bit, so it is best to check the official Unihiker documentation for your preferred method.
Now run the script! You can start the windinator.py via terminal (SSH session) or directly via touch screen menu on Unihiker.
If you own, for example, an ESP32-S3 AI Cam Module and a 300 degree servo motor, then expand this project with face tracking! Then the fan could follow you if you move back and forth at your desk.
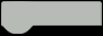