A simple Python class to control the screen (on, off, brightness) of the UNIHIKER as an alternative for using the ‘brightness’ script (https://www.unihiker.com/wiki/Troubleshooting/How_can_I_adjust_the_screen_brightness_of_UNIHIKER/) via system call …
CODE
# UnihikerScreen: A simple class to control the screen (on, off & brightness) of a Unihiker ...
from periphery import PWM
class UnihikerScreen:
PWM_CHIP = 1 # '/sys/class/pwm/pwmchip1/.'
PWM_CHANNEL = 0 # ./pwm0
MAX_BRIGHTNESS = 0.99 # max. duty cycle
def __init__(self, pwm_chip=PWM_CHIP, pwm_channel=PWM_CHANNEL):
# Initialize the PWM device
self.pwm = PWM(pwm_chip, pwm_channel)
self.pwm.frequency = 1000 # Set a default frequency (1 kHz)
self.pwm.polarity = "normal" # Set polarity to 'normal'
self.pwm.enable()
self._brightness = UnihikerScreen.MAX_BRIGHTNESS
def on(self):
# Set duty cycle to the last set brightness to turn the screen on
if self._brightness == 0:
self._brightness = UnihikerScreen.MAX_BRIGHTNESS
self.pwm.duty_cycle = self._brightness
def off(self):
# Set duty cycle to 0% to turn the screen off
self.pwm.duty_cycle = 0.0
def brightness(self, percent):
# Set the brightness as a percentage (0 to 100)
if 0 <= percent < 100:
self._brightness = percent / 100.0
elif percent == 100:
self._brightness = UnihikerScreen.MAX_BRIGHTNESS
else:
raise ValueError("Brightness percent must be between 0 and 100")
self.pwm.duty_cycle = self._brightness
def __del__(self):
# Clean up the PWM device
self.pwm.close()
Example usage:
Copy the code into a file UnihikerScreen.py into the current directory and …
CODE
from UnihikerScreen import UnihikerScreen
import time
screen = UnihikerScreen()
# Turn off ...
screen.off()
time.sleep(1)
# Turn back on ...
screen.on()
time.sleep(1)
# Set brightness to 25% ...
screen.brightness(25)
time.sleep(1)
# Turn off ...
screen.off()
time.sleep(1)
# Turn back on (to 25% brightness) ...
screen.on()
time.sleep(1)
Code and samples at https://gist.github.com/dxcfl/7ee3e0768c4eecec47311dc5614b6831
License 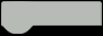
All Rights
Reserved
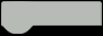
0
More from this category