We make a smart system in which attendance is taken by the RFID module and the controller we use for this is Arduino UNO.

Things used in this project
Hardware components
Software apps and online services
Arduino IDE
Story
Hello geeks, hope you are doing fine. Have you ever wonder that what if we have a smart card to mark our attendance? So keeping this in mind we are writing this article to make an RFID-based attendance syste using Arduino UNO board. You can read the full project on our website as well. The students can enroll themself by just placing the smart card on the reader module. For doing this we are using an RC522 RFID reader and writer module. Do check out more projects on Arduino.

How Does RFID-based attendance system Work?
This system works on radio frequency identification. The smart card which you have to put on the reader module is pre-coded with the roll number. Whenever someone uses a card whose information is not registered in the memory the red LED will go on and the buzzer starts beeping. When the system is on it will ask you to show your smart card. For displaying the contents we are using a 16×2 LCD with an I2C module.

When the RFID reads the card that is coded with the correct details the Green LED will glow. The LCD will display the name of the student and a message “present“.

You can add as many students as you want and also change their names by modifying the code.


If the details of the card are not present then the Red LED will be on and the LCD displays “unauthorized access” as shown below.


Components Required
-Arduino UNO
-RC522 RFID module
-Different RFID tags
-Jumper wires and a breadboard
-Red and green LED
-Buzzer and a 220-ohm resistor
-I2C module
-16×2 LCD display
-USB cable for uploading the code
-RFID based attendance system Circuit Diagram

Arduino 5-volts pin -> VCC of the I2c module
Arduino GND pin -> GND of the I2C module
Arduino analog-4 pin -> SDA of the I2C module
Arduino analog-5 pin -> SCL of the I2C module
Arduino digital-2 pin -> positive leg of buzzer
Arduino digital-4 pin -> positive leg of red LED
Arduino digital-5 pin -> positive leg of green LED
Without I2C module

RFID based attendance system Code
With I2C module
// Techatronic.com
#include
#include
#include
#include
// Set the LCD address to 0x27 for a 16 chars and 2 line display
LiquidCrystal_I2C lcd(0x27, 16, 2);
#define SS_PIN 10
#define RST_PIN 9
#define LED_G 5 //define green LED pin
#define LED_R 4 //define red LED pin
#define BUZZER 2 //buzzer pin
MFRC522 mfrc522(SS_PIN, RST_PIN); // Create MFRC522 instance.
void setup()
{
Serial.begin(9600); // Initiate a serial communication
SPI.begin(); // Initiate SPI bus
mfrc522.PCD_Init(); // Initiate MFRC522
lcd.begin();
lcd.backlight(); // Turn on the blacklight and print a message.
pinMode(LED_G, OUTPUT);
pinMode(LED_R, OUTPUT);
pinMode(BUZZER, OUTPUT);
noTone(BUZZER);
}
void loop()
{
// Look for new cards
if ( ! mfrc522.PICC_IsNewCardPresent())
{
lcd.setCursor(3,0);
lcd.print("SHOW YOUR");
lcd.setCursor(4,1);
lcd.print("ID CARD");
return;
}
else{
lcd.clear();
}
// Select one of the cards
if ( ! mfrc522.PICC_ReadCardSerial())
{
return;
}
//Show UID on serial monitor
Serial.print("UID tag :");
String content= "";
byte letter;
for (byte i = 0; i < mfrc522.uid.size; i++)
{
Serial.print(mfrc522.uid.uidByte[i] < 0x10 ? " 0" : " ");
Serial.print(mfrc522.uid.uidByte[i], HEX);
content.concat(String(mfrc522.uid.uidByte[i] < 0x10 ? " 0" : " "));
content.concat(String(mfrc522.uid.uidByte[i], HEX));
}
Serial.println();
content.toUpperCase();
if (content.substring(1) == "36 B1 03 32") //change here the UID of the card/cards that you want to give access
{
lcd.print("STUDENT 01");
lcd.setCursor(0,1);
lcd.print("PRESENT");
digitalWrite(LED_G, HIGH);
tone(BUZZER, 500);
delay(300);
noTone(BUZZER);
delay(3000);
digitalWrite(LED_G, LOW);
lcd.clear();
}
else if (content.substring(1) == "81 93 40 43") //change here the UID of the card/cards that you want to give access
{
lcd.print("STUDENT 02");
lcd.setCursor(0,1);
lcd.print("PRESENT");
digitalWrite(LED_G, HIGH);
tone(BUZZER, 500);
delay(300);
noTone(BUZZER);
delay(3000);
digitalWrite(LED_G, LOW);
lcd.clear();
}
else if (content.substring(1) == "91 69 3E 43") //change here the UID of the card/cards that you want to give access
{
lcd.print("STUDENT 03");
lcd.setCursor(0,1);
lcd.print("PRESENT");
digitalWrite(LED_G, HIGH);
tone(BUZZER, 500);
delay(300);
noTone(BUZZER);
delay(3000);
digitalWrite(LED_G, LOW);
lcd.clear();
}
else {
lcd.print("UNAUTHORIZE");
lcd.setCursor(0,1);
lcd.print("ACCESS");
digitalWrite(LED_R, HIGH);
tone(BUZZER, 300);
delay(2000);
digitalWrite(LED_R, LOW);
noTone(BUZZER);
lcd.clear();
}
}
Without I2C module
#include
#include
#include "LiquidCrystal.h"
LiquidCrystal lcd(A0, A1, A2, A3, A4, A5);
#define SS_PIN 10
#define RST_PIN 9
#define LED_G 5 //define green LED pin
#define LED_R 4 //define red LED pin
#define BUZZER 2 //buzzer pin
MFRC522 mfrc522(SS_PIN, RST_PIN); // Create MFRC522 instance.
void setup()
{
Serial.begin(9600); // Initiate a serial communication
SPI.begin(); // Initiate SPI bus
mfrc522.PCD_Init(); // Initiate MFRC522
lcd.begin(16,2); // Turn on the blacklight and print a message.
pinMode(LED_G, OUTPUT);
pinMode(LED_R, OUTPUT);
pinMode(BUZZER, OUTPUT);
noTone(BUZZER);
}
void loop()
{
// Look for new cards
if ( ! mfrc522.PICC_IsNewCardPresent())
{
lcd.setCursor(3,0);
lcd.print("SHOW YOUR");
lcd.setCursor(4,1);
lcd.print("ID CARD");
return;
}
else{
lcd.clear();
}
// Select one of the cards
if ( ! mfrc522.PICC_ReadCardSerial())
{
return;
}
//Show UID on serial monitor
Serial.print("UID tag :");
String content= "";
byte letter;
for (byte i = 0; i < mfrc522.uid.size; i++)
{
Serial.print(mfrc522.uid.uidByte[i] < 0x10 ? " 0" : " ");
Serial.print(mfrc522.uid.uidByte[i], HEX);
content.concat(String(mfrc522.uid.uidByte[i] < 0x10 ? " 0" : " "));
content.concat(String(mfrc522.uid.uidByte[i], HEX));
}
Serial.println();
content.toUpperCase();
if (content.substring(1) == "36 B1 03 32") //change here the UID of the card/cards that you want to give access
{
lcd.print("STUDENT 01");
lcd.setCursor(0,1);
lcd.print("PRESENT");
digitalWrite(LED_G, HIGH);
tone(BUZZER, 500);
delay(300);
noTone(BUZZER);
delay(3000);
digitalWrite(LED_G, LOW);
lcd.clear();
}
else if (content.substring(1) == "81 93 40 43") //change here the UID of the card/cards that you want to give access
{
lcd.print("STUDENT 02");
lcd.setCursor(0,1);
lcd.print("PRESENT");
digitalWrite(LED_G, HIGH);
tone(BUZZER, 500);
delay(300);
noTone(BUZZER);
delay(3000);
digitalWrite(LED_G, LOW);
lcd.clear();
}
else if (content.substring(1) == "91 69 3E 43") //change here the UID of the card/cards that you want to give access
{
lcd.print("STUDENT 03");
lcd.setCursor(0,1);
lcd.print("PRESENT");
digitalWrite(LED_G, HIGH);
tone(BUZZER, 500);
delay(300);
noTone(BUZZER);
delay(3000);
digitalWrite(LED_G, LOW);
lcd.clear();
}
else {
lcd.print("UNAUTHORIZE");
lcd.setCursor(0,1);
lcd.print("ACCESS");
digitalWrite(LED_R, HIGH);
tone(BUZZER, 300);
delay(2000);
digitalWrite(LED_R, LOW);
noTone(BUZZER);
lcd.clear();
}
}
We hope that you understand the concept of the project and must try to make it on your own. For more interesting projects visit our official website.
HAPPY LEARNING!
Code
// Techatronic.com
#include <SPI.h>
#include <MFRC522.h>
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
// Set the LCD address to 0x27 for a 16 chars and 2 line display
LiquidCrystal_I2C lcd(0x27, 16, 2);
#define SS_PIN 10
#define RST_PIN 9
#define LED_G 5 //define green LED pin
#define LED_R 4 //define red LED pin
#define BUZZER 2 //buzzer pin
MFRC522 mfrc522(SS_PIN, RST_PIN); // Create MFRC522 instance.
void setup()
{
Serial.begin(9600); // Initiate a serial communication
SPI.begin(); // Initiate SPI bus
mfrc522.PCD_Init(); // Initiate MFRC522
lcd.begin();
lcd.backlight(); // Turn on the blacklight and print a message.
pinMode(LED_G, OUTPUT);
pinMode(LED_R, OUTPUT);
pinMode(BUZZER, OUTPUT);
noTone(BUZZER);
}
void loop()
{
// Look for new cards
if ( ! mfrc522.PICC_IsNewCardPresent())
{
lcd.setCursor(3,0);
lcd.print("SHOW YOUR");
lcd.setCursor(4,1);
lcd.print("ID CARD");
return;
}
else{
lcd.clear();
}
// Select one of the cards
if ( ! mfrc522.PICC_ReadCardSerial())
{
return;
}
//Show UID on serial monitor
Serial.print("UID tag :");
String content= "";
byte letter;
for (byte i = 0; i < mfrc522.uid.size; i++)
{
Serial.print(mfrc522.uid.uidByte[i] < 0x10 ? " 0" : " ");
Serial.print(mfrc522.uid.uidByte[i], HEX);
content.concat(String(mfrc522.uid.uidByte[i] < 0x10 ? " 0" : " "));
content.concat(String(mfrc522.uid.uidByte[i], HEX));
}
Serial.println();
content.toUpperCase();
if (content.substring(1) == "36 B1 03 32") //change here the UID of the card/cards that you want to give access
{
lcd.print("STUDENT 01");
lcd.setCursor(0,1);
lcd.print("PRESENT");
digitalWrite(LED_G, HIGH);
tone(BUZZER, 500);
delay(300);
noTone(BUZZER);
delay(3000);
digitalWrite(LED_G, LOW);
lcd.clear();
}
else if (content.substring(1) == "81 93 40 43") //change here the UID of the card/cards that you want to give access
{
lcd.print("STUDENT 02");
lcd.setCursor(0,1);
lcd.print("PRESENT");
digitalWrite(LED_G, HIGH);
tone(BUZZER, 500);
delay(300);
noTone(BUZZER);
delay(3000);
digitalWrite(LED_G, LOW);
lcd.clear();
}
else if (content.substring(1) == "91 69 3E 43") //change here the UID of the card/cards that you want to give access
{
lcd.print("STUDENT 03");
lcd.setCursor(0,1);
lcd.print("PRESENT");
digitalWrite(LED_G, HIGH);
tone(BUZZER, 500);
delay(300);
noTone(BUZZER);
delay(3000);
digitalWrite(LED_G, LOW);
lcd.clear();
}
else {
lcd.print("UNAUTHORIZE");
lcd.setCursor(0,1);
lcd.print("ACCESS");
digitalWrite(LED_R, HIGH);
tone(BUZZER, 300);
delay(2000);
digitalWrite(LED_R, LOW);
noTone(BUZZER);
lcd.clear();
}
}
SPI library for Teensy & Arduino IDE — Read More
Arduino RFID Library for MFRC522 — Read More
Wire library used on Teensy boards — Read More
Library for the LiquidCrystal LCD display connected to an Arduino board. — Read More
The article was first published in hackster, July 24, 2021
cr: https://www.hackster.io/electronicprojects/rfid-based-attendance-system-using-arduino-d3d719
author: Techatronic
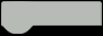