This is a fire alarm system that we make using nodemcu and flame sensor.
Things used in this project
Hardware components
Software apps and online services
Arduino IDE
Story
Hey Techies, Today we are going to make a very useful and trending project which is needed to be installed in every house. It is a Blynk Fire Alarm system. This is a fire alarm that rings whenever it detects fire. For detecting the flames we are using a flame sensor. Make the circuit according to the diagram which is given below then upload the given code to the nodemcu. For more information regarding this project visit the original post of this project also bookmark TECHATRONIC.COM as all my further projects and tutorials will be pre-uploaded there. Anyways, let's began.
Working of the Project:
The flame sensor that we use is responsible for detecting the fire. When the sensor successfully detects the fire then it sends HIGH signals to the nodemcu. After it, you will receive an alert on your smartphone that fire is detected. This project is very simple in working and also very interesting in making. This system is very cheap in price and if we install it in our homes and other places then we can prevent the accidents happens due to the fire. You will need the following materials for making this project.
Material Required:
-NodeMCU (ESP8266 MOD)
-Flame sensor
-Jumper wires
-Breadboard
-Phone with Wi-Fi connection
Circuit Design:
This is the circuit diagram for this project.

Code for the Project:
NOTE: Copy this code and then paste it to the IDE software.
//TECHATRONIC.COM
// BLYNK LIBRARY
// https://github.com/blynkkk/blynk-library
// ESP8266 LIBRARY
// https://github.com/ekstrand/ESP8266wifi
#define BLYNK_PRINT Serial
#include <ESP8266WiFi.h>
#include <BlynkSimpleEsp8266.h>
BlynkTimer timer;
char auth[] = "your-auth-code"; //Auth code sent via Email
char ssid[] = "your-wifi-ssid"; //Wifi name
char pass[] = "your-wifi-password"; //Wifi Password
int flag=0;
void notifyOnFire()
{
int isButtonPressed = digitalRead(D1);
if (isButtonPressed==1 && flag==0) {
Serial.println("Fire DETECTED");
Blynk.notify("Alert : Fire detected");
flag=1;
}
else if (isButtonPressed==0)
{
flag=0;
}
}
void setup()
{
Serial.begin(9600);
Blynk.begin(auth, ssid, pass);
pinMode(D1,INPUT_PULLUP);
timer.setInterval(1000L,notifyOnFire);
}
void loop()
{
Blynk.run();
timer.run();
}
We hope that you liked this project. Thanks for reading.
HAPPY LEARNING!
Code snippet #1
Plain text
void notifyOnFire()
{
int isButtonPressed = digitalRead(D1);
if (isButtonPressed==1 && flag==0) {
Serial.println("Fire DETECTED");
Blynk.notify("Alert : Fire detected");
flag=1;
}
else if (isButtonPressed==0)
{
flag=0;
}
}
Code snippet #2
Plain text
void notifyOnFire()
{
int isButtonPressed = digitalRead(D1);
if (isButtonPressed==1 && flag==0) {
Serial.println("Fire DETECTED");
Blynk.notify("Alert : Fire detected");
flag=1;
}
else if (isButtonPressed==0)
{
flag=0;
}
}
Code snippet #3
Plain text
void setup()
{
Serial.begin(9600);
Blynk.begin(auth, ssid, pass);
pinMode(D1,INPUT_PULLUP);
timer.setInterval(1000L,notifyOnFire);
}
void loop()
{
Blynk.run();
timer.run();
}
Code snippet #4
Plain text
void setup()
{
Serial.begin(9600);
Blynk.begin(auth, ssid, pass);
pinMode(D1,INPUT_PULLUP);
timer.setInterval(1000L,notifyOnFire);
}
void loop()
{
Blynk.run();
timer.run();
}
Code snippet #5
Plain text
//TECHATRONIC.COM
// BLYNK LIBRARY
// https://github.com/blynkkk/blynk-library
// ESP8266 LIBRARY
// https://github.com/ekstrand/ESP8266wifi
#define BLYNK_PRINT Serial
#include <ESP8266WiFi.h>
#include <BlynkSimpleEsp8266.h>
BlynkTimer timer;
char auth[] = "your-auth-code"; //Auth code sent via Email
char ssid[] = "your-wifi-ssid"; //Wifi name
char pass[] = "your-wifi-password"; //Wifi Password
int flag=0;
void notifyOnFire()
{
int isButtonPressed = digitalRead(D1);
if (isButtonPressed==1 && flag==0) {
Serial.println("Fire DETECTED");
Blynk.notify("Alert : Fire detected");
flag=1;
}
else if (isButtonPressed==0)
{
flag=0;
}
}
void setup()
{
Serial.begin(9600);
Blynk.begin(auth, ssid, pass);
pinMode(D1,INPUT_PULLUP);
timer.setInterval(1000L,notifyOnFire);
}
void loop()
{
Blynk.run();
timer.run();
}
Code snippet #6
Plain text
//TECHATRONIC.COM
// BLYNK LIBRARY
// https://github.com/blynkkk/blynk-library
// ESP8266 LIBRARY
// https://github.com/ekstrand/ESP8266wifi
#define BLYNK_PRINT Serial
#include <ESP8266WiFi.h>
#include <BlynkSimpleEsp8266.h>
BlynkTimer timer;
char auth[] = "your-auth-code"; //Auth code sent via Email
char ssid[] = "your-wifi-ssid"; //Wifi name
char pass[] = "your-wifi-password"; //Wifi Password
int flag=0;
void notifyOnFire()
{
int isButtonPressed = digitalRead(D1);
if (isButtonPressed==1 && flag==0) {
Serial.println("Fire DETECTED");
Blynk.notify("Alert : Fire detected");
flag=1;
}
else if (isButtonPressed==0)
{
flag=0;
}
}
void setup()
{
Serial.begin(9600);
Blynk.begin(auth, ssid, pass);
pinMode(D1,INPUT_PULLUP);
timer.setInterval(1000L,notifyOnFire);
}
void loop()
{
Blynk.run();
timer.run();
}
The article was first published in hackster, September 7, 2021
cr: https://www.hackster.io/electronicprojects/blynk-fire-alarm-e2073a#things
author: Techatronic
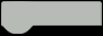