A simulation of a real life car parking using Arduino, Ultrasonic sensors, LCD Display and RGB LEDs.

Things used in this project
Ā
Hardware components
Story
Ā
The Idea
Ā
Hello!
Ā
This project is based on some car parkings, which is, an indication if the local is free or occupied, so for the simulation, I'm going to use two ultrasonic sensors, simulating two parking spots, two RGB LEDs, indicating if is occupied or not, and an I2C LCD display to show informations with images.
Ā
Thinking on that, I want to make a simple project to show this simulation and I hope I'm helping someone who was trying to do, but wasn't with any idea of how to.
Ā
Some solutions
Ā
The first idea is to make a small, simple and functional project, so I'm not going to use too much wires, components, etc. Instead of using two red LEDs and two green LEDs, I'm going to use only two RGB LEDs, using only red and green lights from it. Here I'm using I2C LCD Display to show the images, because it just need four wires and doesn't use any digital port.
Ā
For the images of the Display, I used custom characters, created using this Custom Character Generator.
For the ultrasonic sensor, I'm going to use this Library: Ultrasonic.h
Ā
Project working
Ā
Here is the video of the project working:
Schematics

Code
#include <Ultrasonic.h> //Library for the ultrasonic sensor
#include<LiquidCrystal_I2C.h> //Library for the display
#include<Wire.h>
LiquidCrystal_I2C lcd (0x27, 16, 2); //Creates an object lcd
Ultrasonic ultrasonic1 (13, 12); //Creates an object ultrasonic1
Ultrasonic ultrasonic2 (11, 10); //Creates an object ultrasonic1
int ledOccupied1 = 9; //LED to indicate if the spot is occupied
int ledFree1 = 8; //LED to indicate if the spot is free
int ledOccupied2 = 7; //LED to indicate if the spot is occupied
int ledFree2 = 6; //LED to indicate if the spot is free
int val1; //val1 as a value variable for ultrasonic sensor
int val2; //val1 as a value variable for ultrasonic sensor
byte SpaceA[] = //Custom character to indicate a free spot
{
0b11111,
0b10001,
0b10011,
0b10111,
0b11111,
0b10011,
0b10011,
0b10011
};
byte SpaceB[] = //Custom character to indicate a free spot
{
0b11111,
0b10001,
0b11001,
0b11101,
0b11111,
0b11001,
0b11001,
0b11001
};
byte SpaceC[] = //Custom character to indicate a free spot
{
0b10011,
0b10011,
0b10011,
0b10011,
0b10000,
0b10000,
0b10000,
0b11111
};
byte SpaceD[] = //Custom character to indicate a free spot
{
0b11001,
0b11001,
0b11001,
0b11001,
0b00001,
0b00001,
0b00001,
0b11111
};
byte NoSpaceA[] = //Custom character to indicate a occupied spot
{
0b11111,
0b10000,
0b10000,
0b11000,
0b11100,
0b11110,
0b10111,
0b10011
};
byte NoSpaceB[] = //Custom character to indicate a occupied spot
{
0b11111,
0b00001,
0b00001,
0b00011,
0b00111,
0b01111,
0b11101,
0b11001
};
byte NoSpaceC[] = { //Custom character to indicate a occupied spot
0b10011,
0b10111,
0b11110,
0b11100,
0b11000,
0b10000,
0b10000,
0b11111
};
byte NoSpaceD[] = { //Custom character to indicate a occupied spot
0b11001,
0b11101,
0b01111,
0b00111,
0b00011,
0b00001,
0b00001,
0b11111
};
void setup() {
lcd.init(); //Starts the display
lcd.backlight(); //Turns on the display backlight
//Create the custom characters
lcd.createChar(1, SpaceA);
lcd.createChar(2, SpaceB);
lcd.createChar(3, SpaceC);
lcd.createChar(4, SpaceD);
lcd.createChar(5, NoSpaceA);
lcd.createChar(6, NoSpaceB);
lcd.createChar(7, NoSpaceC);
lcd.createChar(8, NoSpaceD);
//Define all LEDs as Output
pinMode(ledOccupied1, OUTPUT);
pinMode(ledFree1, OUTPUT);
pinMode(ledOccupied2, OUTPUT);
pinMode(ledFree2, OUTPUT);
}
void loop() {
//Value variables will store all informations from the sensors
val1 = ultrasonic1.Ranging(CM);
val2 = ultrasonic2.Ranging(CM);
//If the first spot is occupied
if ((val1 < 10) && (val2 > 10)) {
digitalWrite(ledOccupied1, HIGH);
digitalWrite(ledFree1, LOW);
digitalWrite(ledOccupied2, LOW);
digitalWrite(ledFree2, HIGH);
lcd.clear();
lcd.write(5);
lcd.setCursor(1, 0);
lcd.write(6);
lcd.setCursor(0, 1);
lcd.write(7);
lcd.setCursor(1, 1);
lcd.write(8);
lcd.setCursor(7, 0);
lcd.write(1);
lcd.setCursor(8, 0);
lcd.write(2);
lcd.setCursor(7, 1);
lcd.write(3);
lcd.setCursor(8, 1);
lcd.write(4);
delay(500);
}
//If the second spot is occupied
else if ((val1 > 10) && (val2 < 10)) {
digitalWrite(ledOccupied1, LOW);
digitalWrite(ledFree1, HIGH);
digitalWrite(ledOccupied2, HIGH);
digitalWrite(ledFree2, LOW);
lcd.clear();
lcd.write(1);
lcd.setCursor(1, 0);
lcd.write(2);
lcd.setCursor(0, 1);
lcd.write(3);
lcd.setCursor(1, 1);
lcd.write(4);
lcd.setCursor(7, 0);
lcd.write(5);
lcd.setCursor(8, 0);
lcd.write(6);
lcd.setCursor(7, 1);
lcd.write(7);
lcd.setCursor(8, 1);
lcd.write(8);
delay(500);
}
//If the first and second spots are free
else if ((val1 > 10) && (val2 > 10)) {
digitalWrite(ledOccupied1, LOW);
digitalWrite(ledFree1, HIGH);
digitalWrite(ledOccupied2, LOW);
digitalWrite(ledFree2, HIGH);
lcd.clear();
lcd.write(1);
lcd.setCursor(1, 0);
lcd.write(2);
lcd.setCursor(0, 1);
lcd.write(3);
lcd.setCursor(1, 1);
lcd.write(4);
lcd.setCursor(7, 0);
lcd.write(1);
lcd.setCursor(8, 0);
lcd.write(2);
lcd.setCursor(7, 1);
lcd.write(3);
lcd.setCursor(8, 1);
lcd.write(4);
delay(500);
}
//If the first and second spots are occupied
else if ((val1 < 10) && (val2 < 10)) {
digitalWrite(ledOccupied1, HIGH);
digitalWrite(ledFree1, LOW);
digitalWrite(ledOccupied2, HIGH);
digitalWrite(ledFree2, LOW);
lcd.clear();
lcd.write(5);
lcd.setCursor(1, 0);
lcd.write(6);
lcd.setCursor(0, 1);
lcd.write(7);
lcd.setCursor(1, 1);
lcd.write(8);
lcd.setCursor(7, 0);
lcd.write(5);
lcd.setCursor(8, 0);
lcd.write(6);
lcd.setCursor(7, 1);
lcd.write(7);
lcd.setCursor(8, 1);
lcd.write(8);
delay(500);
}
else {
lcd.clear();
digitalWrite(ledOccupied1, LOW);
digitalWrite(ledFree1, LOW);
digitalWrite(ledOccupied2, LOW);
digitalWrite(ledFree2, LOW);
}
}
The article was first published in hackster, April 10, 2022
cr: https://www.hackster.io/bruno_opaiva/car-parking-simulator-df9caa
author: bruno_opaiva
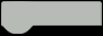